10 Common Application Security Pitfalls to Avoid
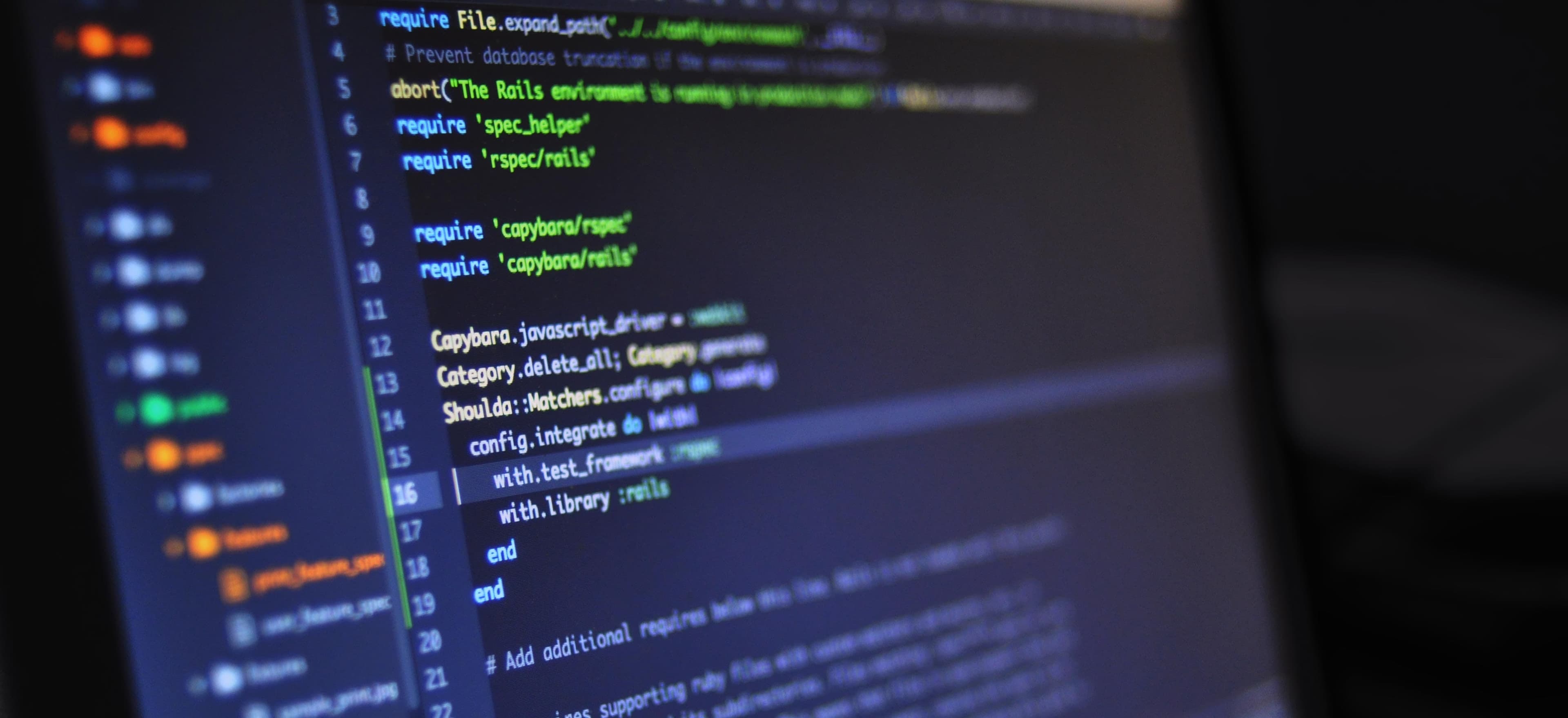
- Published on
Top 10 Common Application Security Pitfalls to Avoid
As developers, it's crucial to prioritize application security at every stage of the development process. Failing to do so can lead to severe vulnerabilities that could compromise the integrity of the entire system. In this article, we'll discuss the top 10 common application security pitfalls to avoid, along with practical tips on how to mitigate these risks.
1. Injection Attacks
Injection attacks, such as SQL injection and NoSQL injection, occur when untrusted data is sent to an interpreter as part of a command or query. To prevent injection attacks, always use parameterized queries or prepared statements when interacting with databases. This approach ensures that user input is never directly concatenated into the query.
For example, in Java using JDBC for database interactions:
String username = request.getParameter("username");
PreparedStatement statement = connection.prepareStatement("SELECT * FROM users WHERE username = ?");
statement.setString(1, username);
ResultSet resultSet = statement.executeQuery();
By using prepared statements, you can effectively mitigate the risk of injection attacks.
2. Cross-Site Scripting (XSS)
Cross-site scripting occurs when an application includes untrusted data in a new web page without proper validation or escaping, allowing an attacker to execute malicious scripts in the victim's browser. To prevent XSS, always sanitize and validate input, and use security controls like Content Security Policy (CSP) to restrict the sources from which certain types of content can be loaded.
For example, using OWASP Java Encoder to encode user input before rendering it in the HTML page:
String userInput = request.getParameter("input");
String encodedInput = ESAPI.encoder().encodeForHTML(userInput);
Utilizing encoding libraries like OWASP Java Encoder can help mitigate the risk of XSS vulnerabilities.
3. Broken Authentication
Improper implementation of authentication and session management can lead to broken authentication, allowing attackers to compromise user accounts, session tokens, or exploit other flaws to assume other users' identities. To avoid this pitfall, always use strong and unique passwords, implement multi-factor authentication, and use secure, industry-standard authentication protocols such as OAuth or OpenID Connect.
4. Insecure Direct Object References
Insecure direct object references occur when an application exposes internal implementation objects, such as files or database keys, to users without proper authorization checks. To prevent this, always perform access control checks to ensure that users are authorized to access the requested resources.
For example, in a file download endpoint:
String requestedFile = request.getParameter("file");
if (userHasAccess(user, requestedFile)) {
// Serve the file
} else {
// Return access denied error
}
By implementing proper access control checks, you can prevent insecure direct object references.
5. Security Misconfiguration
Security misconfigurations, such as default credentials, unnecessary services, and incomplete security settings, can lead to severe vulnerabilities. To avoid security misconfigurations, conduct regular security reviews, follow security best practices, and use automated tools to detect and remediate misconfigurations in your applications and infrastructure.
6. Sensitive Data Exposure
Failing to properly protect sensitive data, such as personally identifiable information (PII) and financial information, can lead to serious consequences. Always encrypt sensitive data at rest and in transit using strong, industry-standard algorithms and protocols. Additionally, ensure that sensitive data is only accessible to authorized users and is never exposed in error messages or logs.
7. Using Components with Known Vulnerabilities
Many applications rely on third-party libraries and components. However, using outdated or vulnerable components can introduce security risks. Regularly update and patch all dependencies, monitor security advisories, and use tools like OWASP Dependency-Check to identify and remediate components with known vulnerabilities.
8. Insufficient Logging and Monitoring
Inadequate logging and monitoring can prevent timely detection and response to security incidents. Ensure that your application logs relevant security events, such as authentication attempts, access control failures, and potential security breaches. Establish thorough monitoring processes and leverage tools like SIEM (Security Information and Event Management) systems to detect and respond to security issues.
9. Insecure Cryptography
Improper use of cryptography, such as using weak algorithms or improper key management, can render encryption ineffective. Always use strong cryptographic algorithms and ensure proper key generation, storage, and rotation practices. Leverage industry best practices and libraries, such as Java Cryptography Architecture (JCA), for secure cryptographic operations.
10. Lack of Input Validation
Failing to validate and sanitize input can lead to various security vulnerabilities, including injection attacks, XSS, and command injection. Always validate and sanitize input from all untrusted sources, including user inputs, API requests, and external data. Utilize input validation libraries and frameworks, such as Hibernate Validator and Apache Commons Validator, to enforce strong input validation rules.
In conclusion, prioritizing application security and avoiding these common pitfalls is paramount in safeguarding your applications and data against potential threats. By implementing robust security measures and best practices, you can create resilient and secure applications that protect both your organization and its users.
Remember, security is not a one-time effort; it's an ongoing process that requires continuous attention and adaptation to emerging threats and vulnerabilities.
For further insights into application security best practices, refer to the OWASP Top 10 project (https://owasp.org/www-project-top-ten/) and the SANS Institute's collection of security resources (https://www.sans.org/). These valuable resources provide comprehensive guidance on addressing and mitigating common security pitfalls.