Optimizing Java Memory Usage in Containers
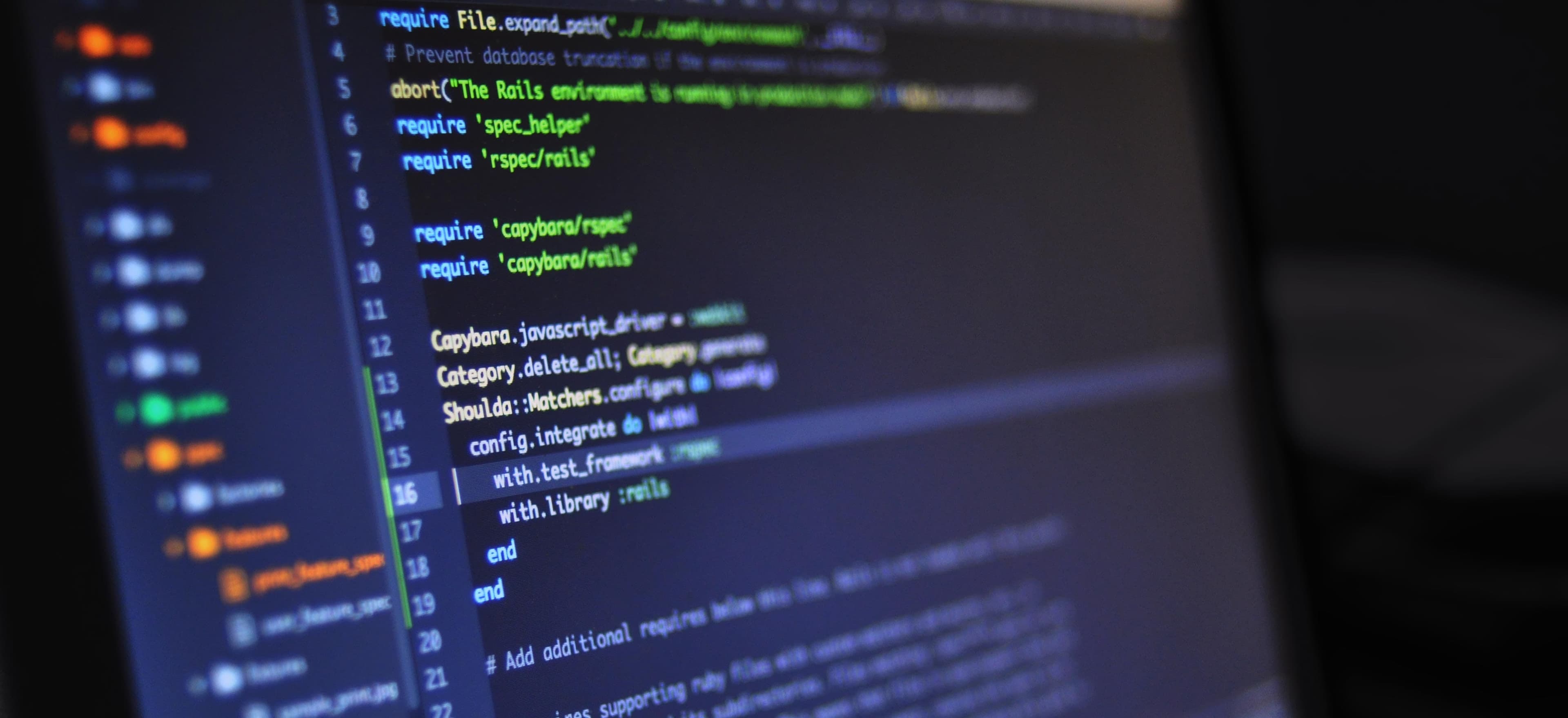
- Published on
Optimizing Java Memory Usage in Containers
When running Java applications in containers, it's essential to ensure that the memory usage is optimized for efficient performance. In this article, we'll explore some best practices and techniques for optimizing Java memory usage in containerized environments.
Understand Java Memory Management
Before delving into optimization techniques, let's briefly review how memory management works in Java.
Java applications rely on the Java Virtual Machine (JVM) to manage memory. The JVM divides the memory into different regions such as Heap, Stack, and Metaspace. The Heap is where the objects are allocated, and it's the primary area that requires optimization. The Stack is used for storing local variables and method call information, while Metaspace holds the class metadata.
Use a Base Image with Smaller Footprint
When containerizing a Java application, start with a base image that has a smaller footprint. Using a smaller base image helps reduce the overall memory footprint of the container. Options like Alpine Linux-based OpenJDK images provide a lightweight base for Java applications.
Here's an example of using a smaller base image with OpenJDK:
FROM adoptopenjdk:11-jre-hotspot as builder
...
Adjust Java Heap Size
Optimizing the Java Heap size is crucial for efficient memory usage. By default, the JVM allocates a certain amount of memory for the Heap based on the host system's configuration. However, when running in a container, it's beneficial to explicitly set the Heap size based on the container's resource limits.
You can set the initial and maximum Heap size using the -Xms
and -Xmx
flags, respectively. For example, to set the initial Heap size to 256MB and the maximum Heap size to 1GB, you can use the following JVM flags:
java -Xms256m -Xmx1g -jar your-application.jar
Setting the appropriate Heap size prevents Java applications from consuming excessive memory within the container.
Enable Container Awareness in Java 8 and Later
Starting from Java 8, JVM provides options for container awareness, which allows the JVM to understand resource constraints enforced by the container environment. This feature helps the JVM tune its internal parameters based on container limits, resulting in better memory utilization.
To enable container awareness, you can use the -XX:+UnlockExperimentalVMOptions
and -XX:+UseCGroupMemoryLimitForHeap
flags when starting the JVM.
java -XX:+UnlockExperimentalVMOptions -XX:+UseCGroupMemoryLimitForHeap -jar your-application.jar
By enabling container awareness, the JVM can adapt its memory allocation based on the container memory limits, leading to improved resource utilization.
Utilize Efficient Garbage Collection Algorithms
Garbage collection plays a significant role in managing memory within the JVM. Choosing the right garbage collection algorithm can impact memory usage and application performance.
For containerized Java applications, consider using the G1 (Garbage First) garbage collector, which is designed to provide low-latency and efficient memory management. You can enable the G1 garbage collector using the -XX:+UseG1GC
flag:
java -XX:+UseG1GC -jar your-application.jar
The G1 garbage collector adapts well to containerized environments and is suitable for applications with diverse memory requirements.
Monitor and Benchmark Memory Usage
Continuous monitoring and benchmarking of memory usage are essential for identifying potential optimizations and diagnosing memory-related issues. Tools like VisualVM and JConsole provide insights into memory usage, garbage collection statistics, and heap visualization.
Additionally, consider utilizing container-specific monitoring tools such as Prometheus and Grafana to gain visibility into memory metrics within the containerized environment.
Key Takeaways
Optimizing Java memory usage in containers is crucial for achieving efficient resource utilization and maximizing application performance. By using a smaller base image, adjusting the Java Heap size, enabling container awareness, utilizing efficient garbage collection algorithms, and monitoring memory usage, you can ensure that your Java applications are well-optimized for containerized environments.
When optimizing Java memory in containers, it's important to strike a balance between memory efficiency and application performance, ensuring that the application operates within the resource constraints imposed by the container environment.
By implementing these best practices and techniques, you can enhance the memory efficiency of your Java applications and maximize the benefits of running them in containerized environments.
Remember, optimizing memory usage in Java applications is an ongoing process, and it's essential to continuously benchmark, analyze, and fine-tune memory-related configurations to keep your applications running smoothly in containerized environments.
For more insights on Java memory management and containerization, check out the resources provided by Oracle's Java documentation.