Optimizing Actor Message Exchange in Akka
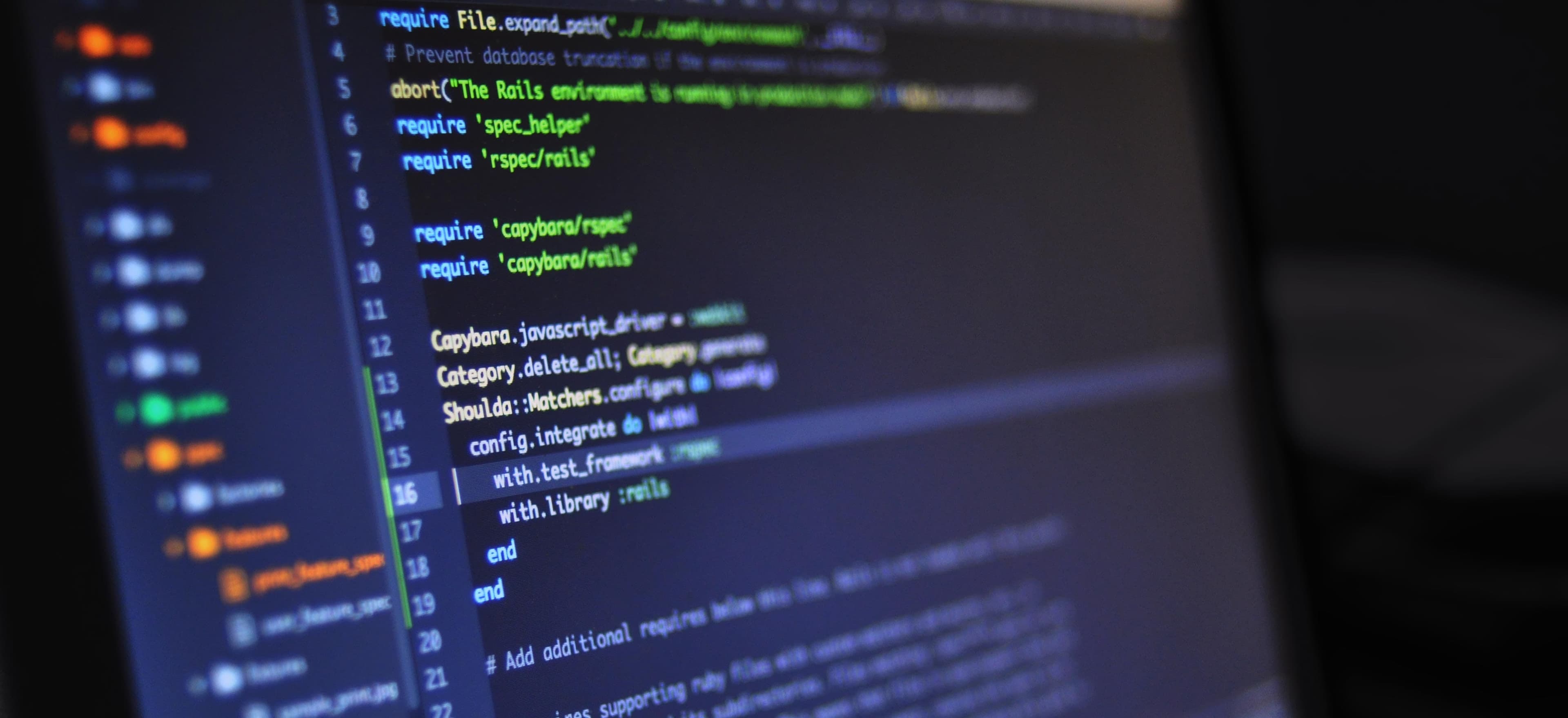
- Published on
Maximizing Performance Through Efficient Message Exchange in Akka
When building concurrent and distributed applications in Java, Akka stands out as a powerful toolkit for simplifying the implementation of high-performance, resilient, and scalable systems. At the heart of Akka lies the concept of actors, which communicate through message passing. This communication mechanism is crucial for the performance of an Akka-based system.
In this post, we will explore strategies to optimize message exchange between actors in Akka, focusing on key considerations and best practices to maximize performance.
Understanding the Actor Model
At the core of Akka's concurrency model is the actor, which encapsulates state and behavior and communicates exclusively through asynchronous message passing. This model ensures a high degree of isolation and encapsulation, promoting a scalable and resilient architecture.
Tip 1: Use Immutable Messages
In Akka, messages exchanged between actors should be immutable. Immutability ensures that messages can be safely shared and processed concurrently without the risk of data corruption. Moreover, immutable messages align with the principles of functional programming, enabling a more predictable and manageable concurrency model.
public class ImmutableMessage {
private final String content;
public ImmutableMessage(String content) {
this.content = content;
}
public String getContent() {
return content;
}
}
By using immutable messages, you can avoid the complexities associated with shared mutable state, leading to more predictable and efficient message exchange.
Tip 2: Embrace Asynchronous Communication
Akka promotes asynchronous communication, allowing actors to continue processing messages while awaiting the arrival of new ones. Leveraging asynchronous communication enhances system responsiveness and resource utilization.
public class SampleActor extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.match(ImmutableMessage.class, this::processMessage)
.build();
}
private void processMessage(ImmutableMessage message) {
// Process the message asynchronously
}
}
Asynchronous communication contributes to improved system throughput and responsiveness, vital for achieving high-performance in Akka-based applications.
Tip 3: Implement Message Compression and Serialization
In a distributed environment, the size of messages can impact network and system performance. Employing message compression techniques can significantly reduce the overhead associated with message exchange. Additionally, using efficient serialization mechanisms, such as Google Protocol Buffers or Apache Avro, can minimize the size of messages transmitted between actors.
public class SampleActor extends AbstractActor {
private final CompressionAlgorithm compression = CompressionAlgorithm.LZ4;
@Override
public Receive createReceive() {
return receiveBuilder()
.match(ImmutableMessage.class, this::processMessage)
.build();
}
private void processMessage(ImmutableMessage message) {
byte[] compressedMessage = compression.compress(message.getContent().getBytes());
// Process the compressed message
}
}
By employing message compression and efficient serialization, you can enhance the overall efficiency of message exchange within your Akka system, particularly in distributed scenarios.
Tip 4: Optimize Mailbox and Dispatcher Configuration
Akka provides customizable mailboxes and dispatchers that influence how messages are queued and processed by actors. Tailoring these configurations to match the specific requirements of your application can significantly impact message exchange performance.
For example, using a priority mailbox can ensure that critical messages are processed with higher precedence, enhancing the overall responsiveness of the system.
prio-mailbox {
mailbox-type = "com.example.CustomPriorityMailbox"
}
By optimizing the mailbox and dispatcher settings, you can align message processing with the application's demands, promoting efficient utilization of system resources.
Tip 5: Use Cluster Sharding for Scalability
In distributed Akka systems, cluster sharding provides a mechanism for distributing actors across multiple nodes while ensuring that messages destined for a particular actor are routed effectively. By utilizing cluster sharding, you can achieve horizontal scalability and efficient message routing in a distributed environment.
ClusterSharding.get(system).shardRegion("Entity");
Cluster sharding simplifies the distribution and management of actors in a clustered Akka system, enabling effective load distribution and optimal message exchange across the cluster.
Tip 6: Measure and Tune Performance
To truly optimize message exchange in an Akka-based system, it is imperative to measure and analyze performance metrics. Leveraging tools such as Kamon or Akka's built-in monitoring capabilities allows you to gather insights into message processing times, actor behaviors, and system throughput.
By monitoring and analyzing performance metrics, you can identify bottlenecks and inefficiencies, enabling targeted optimizations to enhance message exchange performance.
Wrapping Up
Efficient message exchange is fundamental to the performance and scalability of Akka-based applications. By embracing immutable messages, asynchronous communication, efficient serialization, and optimized actor configurations, you can elevate the efficiency and responsiveness of message passing within your Akka system. Additionally, leveraging cluster sharding and diligent performance tuning can further enhance the overall message exchange performance, ensuring the seamless operation of concurrent and distributed systems.
Optimizing message exchange in Akka requires a thoughtful blend of technical expertise, architectural understanding, and performance analysis. By incorporating these strategies and best practices, you can unlock the full potential of Akka's powerful actor model, paving the way for high-performance, scalable, and resilient Java applications.
Incorporating these strategies can significantly enhance the efficiency and responsiveness of your Akka-based application, positioning it for seamless operation within concurrent and distributed environments. By optimizing message exchange, you can leverage the full potential of Akka's powerful actor model, paving the way for high-performance, scalable, and resilient Java applications.