Improving Code Readability with a Single Return Statement
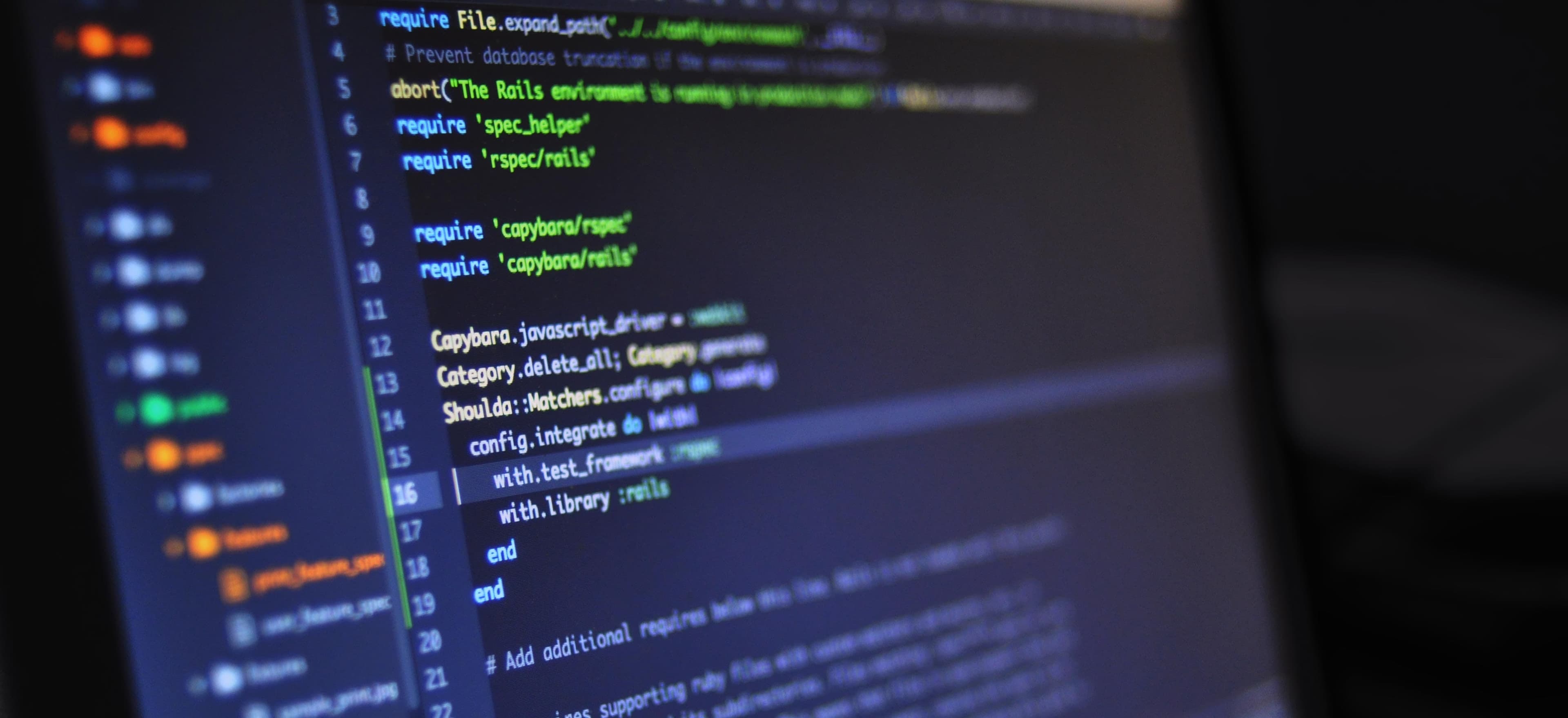
- Published on
Improving Code Readability with a Single Return Statement
One of the key principles of writing clean and maintainable code is readability. It not only makes it easier for others to understand your code but also helps you as the developer to quickly grasp the functionality of your own code when you revisit it after some time. In this post, we'll explore the concept of improving code readability by using a single return statement in Java methods.
The Problem with Multiple Return Statements
Consider the following method:
public int calculateTotalPrice(int price, int quantity) {
int totalPrice = 0;
if (price > 0) {
totalPrice = price * quantity;
return totalPrice;
} else {
return -1;
}
}
In this method, we have multiple return statements based on certain conditions. While this code works perfectly fine, having multiple return statements can make the code harder to understand at a glance. It requires the reader to trace through the different return paths to understand the entire flow of the method.
The Solution: Single Return Statement
By using a single return statement at the end of a method, you can improve the readability of your code significantly. Let's refactor the previous method to use a single return statement:
public int calculateTotalPrice(int price, int quantity) {
if (price > 0) {
return price * quantity;
}
return -1;
}
The refactored code is more concise and easier to understand. The single return statement at the end makes it clear that either the calculated total price is returned or -1
if the price is not valid. This reduces cognitive load and makes the code flow more linear and intuitive.
Maintaining Consistency
When using a single return statement, it's important to be consistent in your codebase. If some methods use a single return statement while others use multiple return statements, it can lead to inconsistency and confusion. Therefore, strive to maintain a uniform approach throughout your codebase to improve overall readability and maintainability.
Handling Early Exit Conditions
One common concern with single return statements is how to handle early exit conditions. For example, if a method needs to perform some validations at the beginning and return early if the conditions are not met. Let's consider an example:
public boolean isInputValid(String input) {
if (input == null || input.isEmpty()) {
return false;
}
// Perform additional validations
return true;
}
In this case, the method returns early if the input is null or empty, and then continues with additional validations only if the initial conditions are met. This approach enhances the readability by clearly indicating the early exit conditions before proceeding with the main logic.
Reducing Nesting Levels
Using a single return statement can also help in reducing the levels of nesting in your code. When you have multiple conditional checks leading to different return statements, the nesting levels can increase, making the code harder to follow. By consolidating the return statements into a single one, you can flatten the structure of your code and make it more linear.
Key Takeaways
In conclusion, using a single return statement in Java methods can significantly improve the readability of your code. It reduces cognitive load, maintains a linear flow, handles early exit conditions effectively, and reduces nesting levels. By striving for consistency in your codebase, you can enhance overall maintainability and make it easier for others to understand and work with your code.
Remember, the goal is not just to make the code work, but to make it easy to understand and maintain. Utilizing a single return statement is a simple yet powerful technique in achieving this goal.
Now that you understand the benefits of using a single return statement, I encourage you to revisit your existing codebase and consider where this technique can be applied for improved readability and maintainability.
For further reading on best practices in Java, you may find the Java Coding Standards guide by Oracle valuable.
Happy coding!