The Deployment Process: Handling Inconsistent Environments
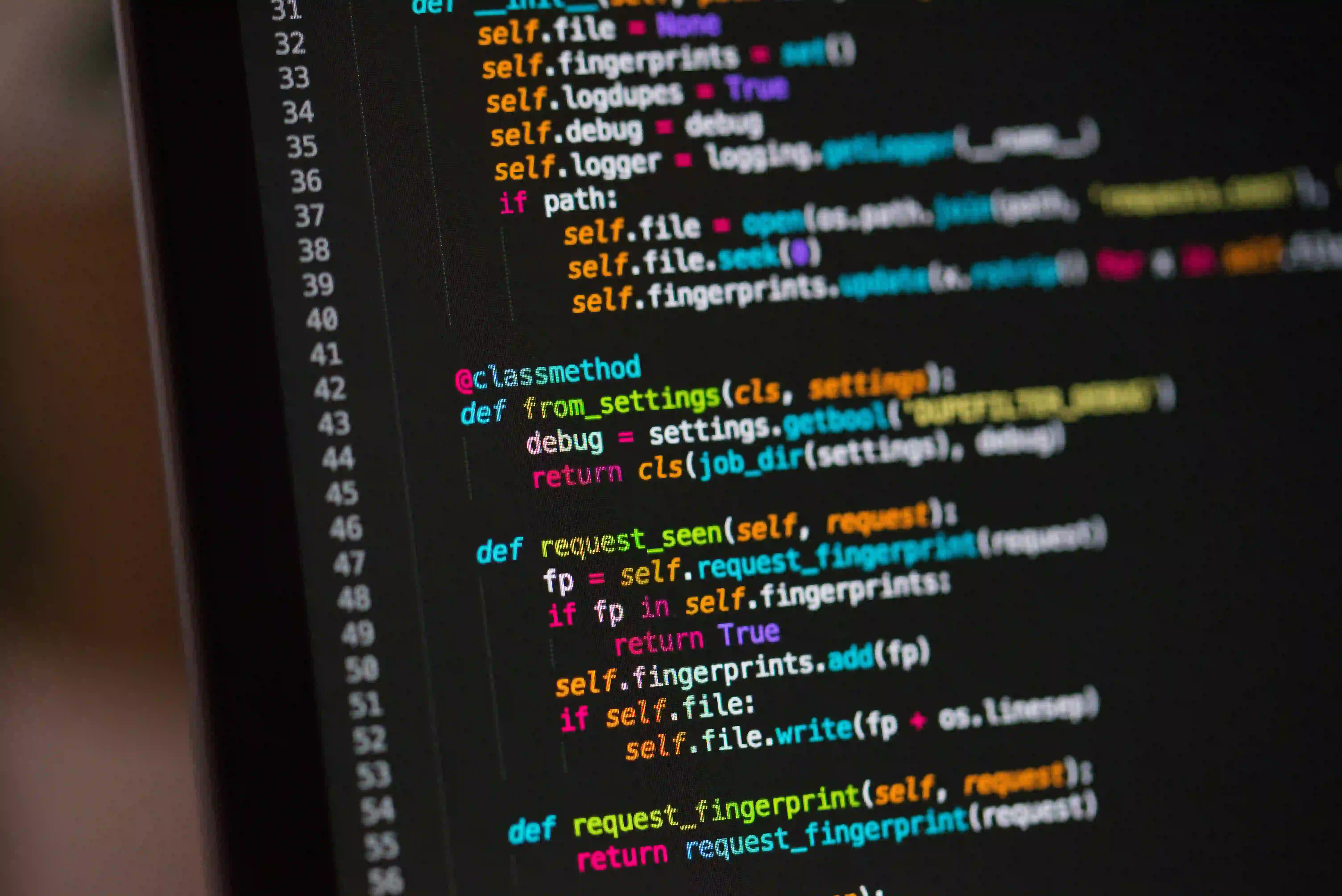
The Deployment Process: Handling Inconsistent Environments
Deploying a Java application can be a challenging task, especially when dealing with inconsistencies across various environments such as development, testing, and production. In this post, we will explore strategies and best practices for handling these inconsistencies in the deployment process.
Understanding Inconsistent Environments
Inconsistent environments arise due to differences in hardware, software configurations, network settings, and other variables. These variations can lead to unexpected behavior in deployed applications. To mitigate these issues, it is crucial to identify and address the inconsistencies during the deployment process.
Using Environment-specific Configuration
One effective approach to handling inconsistent environments is to use environment-specific configuration. By externalizing configuration settings such as database URLs, API endpoints, and logging levels, the application can adapt to different environments without requiring code changes.
public class AppConfig {
private String databaseUrl;
private String apiUrl;
// Other configuration properties
// Getters and Setters
}
In the above code snippet, the AppConfig
class encapsulates environment-specific configuration settings. These settings can be loaded from external properties files, environment variables, or configuration servers.
Leveraging Dependency Injection
Dependency injection frameworks such as Spring facilitate the management of environment-specific components. By injecting different implementations of a component based on the environment, the application's behavior can be tailored accordingly.
@Service
public class UserService {
private UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
In this example, the UserService
class relies on the UserRepository
interface. Depending on the environment, different implementations of the UserRepository
interface can be injected, ensuring seamless integration with diverse databases or external systems.
Automating Environment-specific Builds
Automating the build process for different environments can streamline the deployment workflow. Tools like Apache Maven and Gradle allow for the creation of environment-specific build profiles, enabling the generation of artifacts tailored to each environment's requirements.
<profiles>
<profile>
<id>dev</id>
<activation>
<activeByDefault>true</activeByDefault>
</activation>
<properties>
<environment>dev</environment>
</properties>
</profile>
<profile>
<id>prod</id>
<properties>
<environment>prod</environment>
</properties>
</profile>
</profiles>
The above Maven configuration demonstrates the activation of different build profiles based on the target environment. Utilizing such profiles, environment-specific resources, dependencies, and configurations can be incorporated into the build process.
Testing for Environment Compatibility
Comprehensive testing is essential to ensure that the application behaves as expected across diverse environments. This includes unit testing, integration testing, and end-to-end testing to validate the application's compatibility with varying configurations.
Additionally, tools like Docker and Kubernetes provide mechanisms for creating consistent runtime environments, enabling thorough testing of the application in environments mirroring production settings.
Continuous Integration and Continuous Delivery (CI/CD)
Embracing CI/CD practices can further alleviate the challenges posed by inconsistent environments. Automated pipelines that encompass build, test, and deployment stages facilitate the rapid and reliable dissemination of the application across different environments.
By utilizing tools such as Jenkins, Travis CI, or GitLab CI/CD, developers can establish workflows that promote robustness and consistency in deployment processes.
Final Considerations
Dealing with inconsistent environments in the deployment process requires a combination of meticulous configuration management, automation, and thorough testing. By leveraging environment-specific configuration, dependency injection, automated builds, comprehensive testing, and CI/CD practices, developers can navigate the complexities of inconsistent environments with confidence.
As the deployment landscape continues to evolve, it is imperative for development teams to adapt and refine their deployment strategies to accommodate the ever-changing ecosystem of environments in which their applications operate.
For more in-depth information on Java deployment best practices, you can refer to the official Java documentation.
Feel free to share your thoughts and experiences with handling inconsistent environments in Java deployment!