Handling Message Loss in Akka Systems
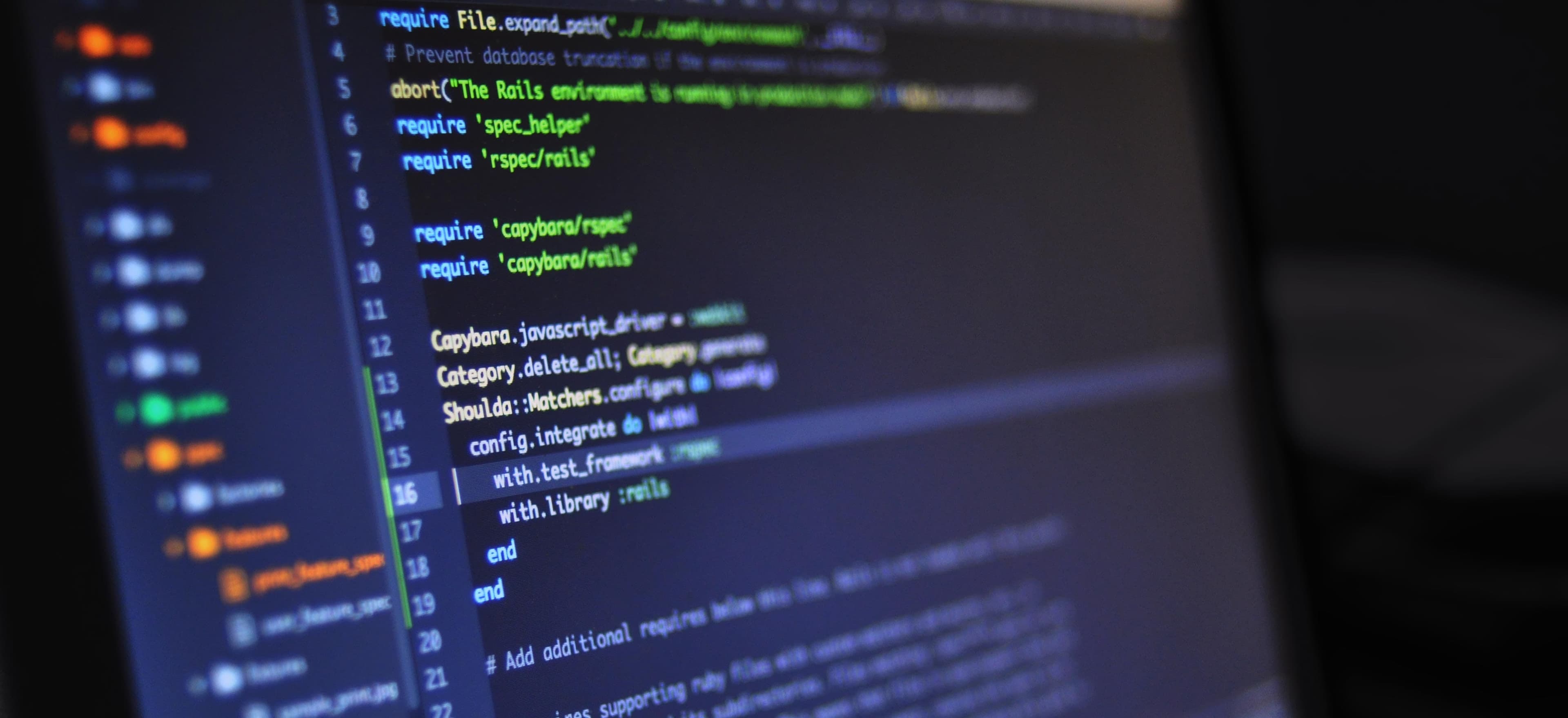
- Published on
Dealing with Message Loss in Akka Systems
When designing distributed systems using Akka, one of the critical challenges is ensuring that messages are not lost during communication between actors. Message loss can lead to inconsistencies in the system and affect its reliability and integrity. In this article, we will explore various strategies to handle message loss in Akka systems and ensure the robustness of the communication mechanism.
Understanding Message Loss
Message loss in distributed systems can occur due to various reasons such as network failures, actor crashes, or system overload. When an actor sends a message to another actor, there is a possibility that the message may not reach its intended destination. This could be due to network issues, actor failures, or other transient faults in the system.
In Akka, actors communicate through message passing, and ensuring the delivery of these messages is crucial for maintaining the consistency and reliability of the system. Therefore, it is imperative to address the potential for message loss and implement strategies to mitigate its impact.
At-Least-Once Delivery
A common approach to handling message loss is to implement an at-least-once delivery mechanism. This ensures that messages are eventually delivered, even if they are lost or duplicated during transmission. By acknowledging the receipt of a message and re-sending it if no acknowledgment is received, we can guarantee that the message will eventually reach its destination.
public class AtLeastOnceDeliveryActor extends AbstractActor {
private final ActorRef destination;
public AtLeastOnceDeliveryActor(ActorRef destination) {
this.destination = destination;
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(Message.class, this::deliverMessage)
.match(Confirm.class, this::handleConfirmation)
.build();
}
private void deliverMessage(Message message) {
// Persist the message and track the delivery
// Send the message to the destination
}
private void handleConfirmation(Confirm confirm) {
// Handle the acknowledgment from the destination
}
}
In the above code snippet, the AtLeastOnceDeliveryActor
class demonstrates how to implement at-least-once message delivery in Akka. When a message is sent, it is tracked and re-sent until it receives confirmation from the destination.
Idempotent Handling
Another approach to mitigate the impact of message loss is to ensure that the processing of messages is idempotent. Idempotent operations produce the same result regardless of the number of times they are executed. By designing message processing logic to be idempotent, we can safely handle potential retransmissions of lost messages without causing inconsistencies in the system.
public class IdempotentMessageProcessor extends AbstractActor {
private Set<String> processedIds = new HashSet<>();
@Override
public Receive createReceive() {
return receiveBuilder()
.match(Message.class, this::processMessage)
.build();
}
private void processMessage(Message message) {
if (!processedIds.contains(message.getId())) {
// Process the message
processedIds.add(message.getId());
}
}
}
In the above example, the IdempotentMessageProcessor
class employs a set to keep track of processed message IDs. When a new message arrives, it checks if the message ID has already been processed and only proceeds with the processing if it's a new message.
Supervision Strategies
Akka provides mechanisms for supervision strategies to handle failures within the actor system. By defining how parent actors should react to the failures of their children, we can implement strategies to recover from message loss due to actor crashes or failures.
public class SupervisorActor extends AbstractActor {
private final ActorRef child = getContext().actorOf(Props.create(ChildActor.class));
@Override
public SupervisorStrategy supervisorStrategy() {
return new OneForOneStrategy(
3, Duration.ofMinutes(1), throwable -> {
if (throwable instanceof MessageLossException) {
return resume();
} else {
return escalate();
}
}
);
}
}
In the above example, the SupervisorActor
defines a supervision strategy using OneForOneStrategy
. If the child actor encounters a MessageLossException
, the strategy is configured to resume the actor, thereby providing a way to recover from message loss scenarios.
Backpressure and Flow Control
In highly concurrent and distributed systems, it is essential to incorporate backpressure and flow control mechanisms to prevent overwhelming actors with a large volume of incoming messages. Backpressure helps in regulating the rate of message delivery, thus reducing the likelihood of message loss due to overload or congestion.
Akka provides support for implementing backpressure and flow control using the akka.stream
package, which allows for the creation of reactive streams that enable efficient handling of asynchronous message processing.
Source<Message, NotUsed> source = // Define the message source
Flow<Message, ProcessedMessage, NotUsed> processor = // Define the message processing flow
Sink<ProcessedMessage, CompletionStage<Done>> sink = // Define the message sink
RunnableGraph<NotUsed> graph = source.via(processor).to(sink);
graph.run(materializer);
The above code snippet illustrates the construction of a reactive stream in Akka using the Source
, Flow
, and Sink
components. By utilizing reactive streams, we can implement backpressure and flow control to manage the delivery of messages and alleviate the risk of message loss.
Closing the Chapter
In the context of Akka systems, addressing message loss is crucial for ensuring the reliability and consistency of distributed communication. By implementing strategies such as at-least-once delivery, idempotent message processing, supervision strategies, and backpressure mechanisms, we can effectively mitigate the impact of message loss and enhance the robustness of Akka-based distributed systems.
Dealing with message loss in Akka requires a comprehensive understanding of the underlying communication mechanisms and fault-tolerance strategies. By adopting the principles and techniques discussed in this article, developers can build resilient and reliable distributed systems using Akka, thereby enabling the seamless exchange of messages between actors while ensuring the integrity of the system as a whole.
In conclusion, by integrating the discussed strategies into Akka systems, developers can bolster the fault tolerance and reliability of message exchange, thereby enhancing the overall robustness of distributed systems.