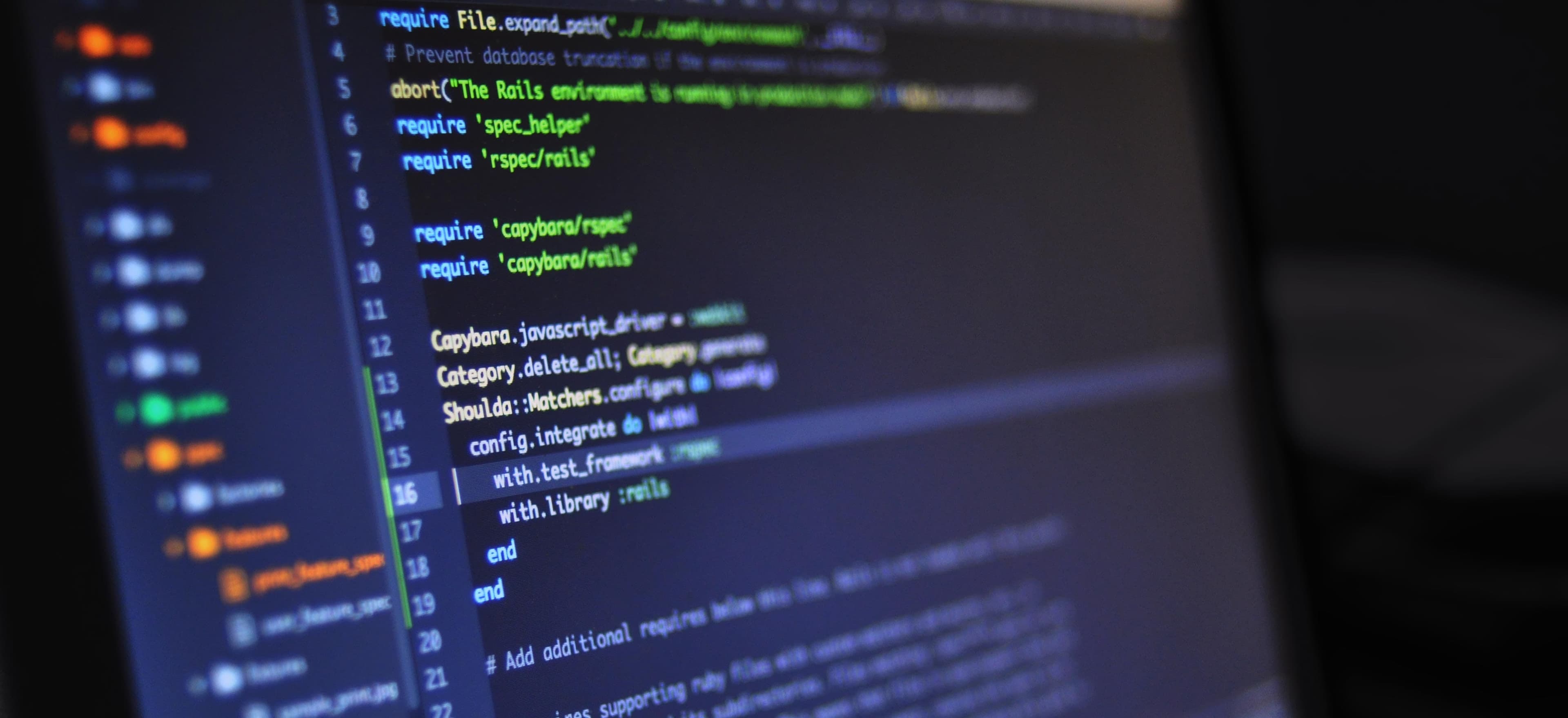
- Published on
Integrating Apache Camel with Spring Boot
In this blog post, we will explore the seamless integration of Apache Camel with Spring Boot. Apache Camel is a powerful open-source integration framework that provides a simple and easy-to-understand approach to integration architecture. Spring Boot, on the other hand, is a popular and widely used framework for building enterprise-ready applications.
Integrating Apache Camel with Spring Boot can bring a new level of flexibility and extensibility to your application, allowing you to easily implement complex integration patterns and handle various message formats. Let's dive into how we can achieve this integration and leverage the capabilities of both frameworks.
Setting Up the Project
To get started, let's set up a new Spring Boot project using either the Spring Initializr or your favorite IDE. Ensure that you include the necessary dependencies for Apache Camel and Spring Boot. You can add the Camel and Spring Boot dependencies in your pom.xml
file if you're using Maven or build.gradle
for Gradle.
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-spring-boot-starter</artifactId>
<version>3.11.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Creating a Simple Camel Route
Now, let's create a simple Camel route to understand the basic integration. Create a new class, for example, SampleCamelRoute
, and annotate it with @Component
to let Spring Boot find and load it.
import org.apache.camel.builder.RouteBuilder;
import org.springframework.stereotype.Component;
@Component
public class SampleCamelRoute extends RouteBuilder {
@Override
public void configure() {
from("direct:start")
.log("Message received: ${body}");
}
}
In this simple example, we've created a Camel route that listens to the direct:start
endpoint and logs the message received. This showcases the simplicity and readability of Camel routes, making it easy to understand the message flow and processing logic.
Running the Application
With the Camel route in place, you can run the Spring Boot application. Upon startup, the Camel context will be automatically created and started, and the Camel route we defined will be deployed and ready to handle incoming messages.
@SpringBootApplication
public class CamelSpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(CamelSpringBootApplication.class, args);
}
}
Adapting to Enterprise Integration Patterns
Apache Camel supports a wide range of enterprise integration patterns (EIP), allowing you to implement complex routing, transformation, and mediation logic. Let's take a look at a more advanced example where we integrate multiple systems using different EIP.
@Component
public class OrderIntegrationRoute extends RouteBuilder {
@Override
public void configure() {
from("direct:receiveOrder")
.choice()
.when(header("orderType").isEqualTo("online"))
.to("direct:processOnlineOrder")
.when(header("orderType").isEqualTo("inStore"))
.to("direct:processInStoreOrder")
.otherwise()
.to("direct:processDefaultOrder");
from("direct:processOnlineOrder")
.log("Processing online order: ${body}");
from("direct:processInStoreOrder")
.log("Processing in-store order: ${body}");
from("direct:processDefaultOrder")
.log("Processing default order: ${body}");
}
}
In this example, we've utilized EIP such as choice()
, when()
, to()
, and otherwise()
to route messages based on their content. This demonstrates how Apache Camel simplifies the implementation of complex integration logic while maintaining readability and maintainability.
Externalizing Configuration
An important aspect of enterprise applications is the ability to externalize configuration, allowing for easier deployment and management. Spring Boot provides excellent support for externalized configuration using property files, YAML files, environment variables, and command-line arguments.
camel:
component:
direct:
properties:
block=true
In the application.yaml
file, you can configure Camel components and endpoints, such as the direct
component properties. This externalized configuration allows the Camel routes and components to be fine-tuned without modifying the application code, providing greater flexibility and reusability.
Error Handling and Dead Letter Channel
Handling errors gracefully is essential in integration scenarios. Apache Camel provides robust error handling capabilities, including the Dead Letter Channel (DLC) pattern, which allows you to redirect failed messages to a separate endpoint for further processing or analysis.
@Component
public class ErrorHandlingRoute extends RouteBuilder {
@Override
public void configure() {
errorHandler(deadLetterChannel("log:deadLetter")
.maximumRedeliveries(3)
.redeliveryDelay(1000));
from("direct:processOrder")
.throwException(new RuntimeException("Failed to process order"));
}
}
In this example, we've configured a Dead Letter Channel to log failed messages after a maximum of 3 redelivery attempts with a delay of 1 second between attempts. This level of control over error handling ensures that your integration processes remain resilient and dependable.
Testing Camel Routes
Testing Camel routes is a critical aspect of ensuring their correctness and robustness. With Spring Boot's support for testing, you can easily write unit tests for your Camel routes using tools like @RunWith
and @MockEndpoints
.
@RunWith(CamelSpringBootRunner.class)
@SpringBootTest
@MockEndpoints("log:*")
public class SampleCamelRouteTest {
@Autowired
private CamelContext camelContext;
@Produce("direct:start")
private ProducerTemplate producerTemplate;
@Test
public void testRoute() throws InterruptedException {
MockEndpoint mock = camelContext.getEndpoint("mock:log:output", MockEndpoint.class);
mock.expectedMessageCount(1);
producerTemplate.sendBody("Test Message");
mock.assertIsSatisfied();
}
}
This test demonstrates how to use @RunWith
to integrate Camel into the Spring Boot test framework, along with @MockEndpoints
to mock the log
endpoint. By employing unit tests, you can verify the behavior of your Camel routes and ensure they meet the expected criteria.
Final Thoughts
Integrating Apache Camel with Spring Boot offers a potent solution for developing robust, extensible, and maintainable integration applications. With the seamless integration of these frameworks, you can leverage the power of Camel's routing and mediation capabilities while benefiting from Spring Boot's simplicity and convention-over-configuration approach.
By combining the strengths of Apache Camel and Spring Boot, you can build enterprise-grade integration solutions that meet the most demanding integration requirements. Whether you're implementing simple message routing or complex enterprise integration patterns, the combination of Apache Camel and Spring Boot provides an exceptional platform for integration development.
In future posts, we'll explore more advanced features and integrations with Apache Camel and Spring Boot, so stay tuned for more insights into mastering enterprise integration.
Checkout our other articles