Simplifying JUnit 5 with Gradle: One Extra Line Needed!
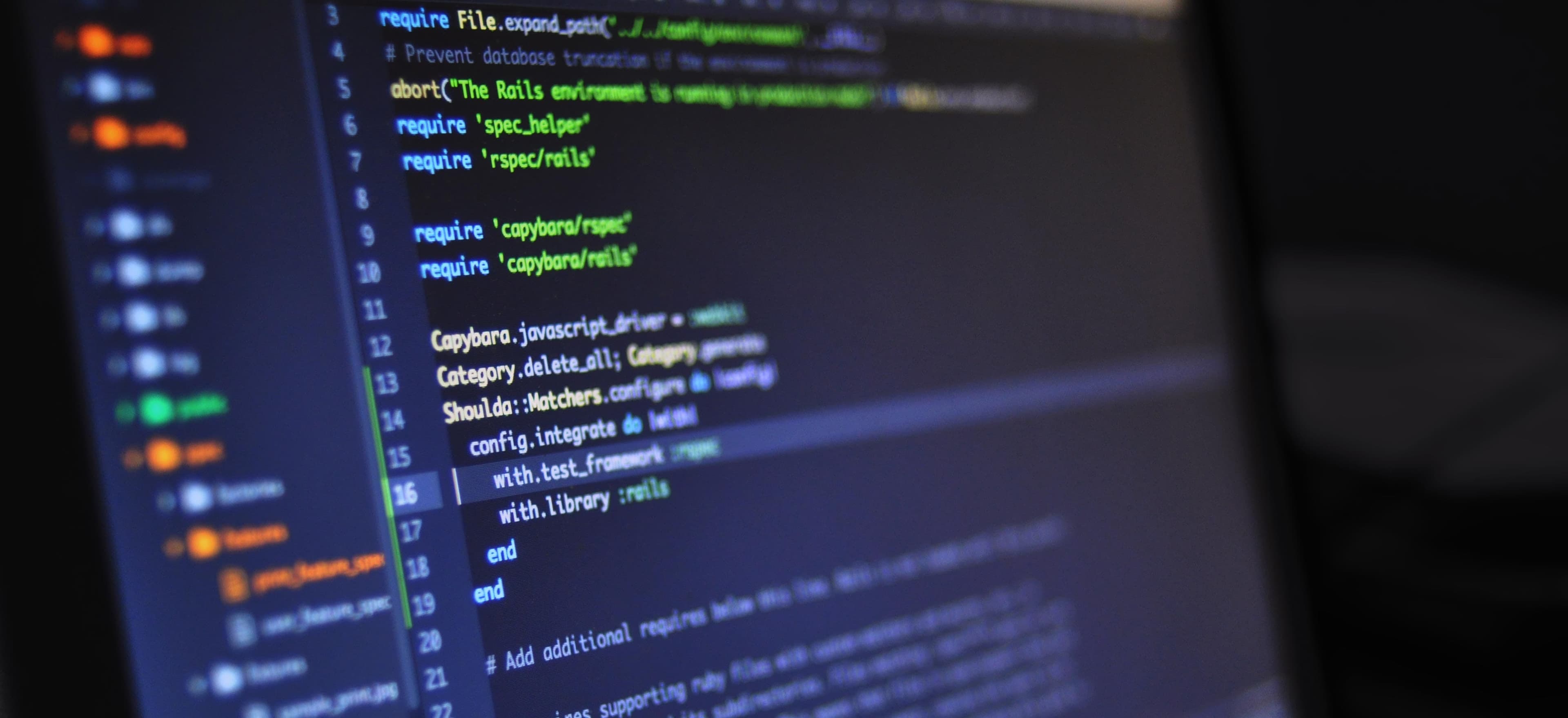
- Published on
Simplifying JUnit 5 with Gradle: One Extra Line Needed!
Testing is a critical part of software development, and JUnit 5 has emerged as one of the most popular testing frameworks in the Java ecosystem. When coupled with Gradle, JUnit 5 can streamline testing processes significantly. However, many developers experience setup confusion, often missing one crucial step. In this blog post, we will simplify JUnit 5 integration with Gradle, focusing on that “one extra line” needed in your configuration.
Understanding JUnit 5 and Gradle
JUnit 5, also known as Jupiter, supports both unit and integration testing. It provides a flexible architecture that allows you to write tests using annotations, assertions, and extension models.
Gradle, on the other hand, is a powerful build automation tool that manages dependencies, compiles code, and executes tests seamlessly. These two tools work together exceedingly well, but there are nuances in setting them up correctly.
Setting Up Your Project
To get started, you will need a basic Gradle project. If you haven’t created one yet, open your terminal and follow these simple steps:
-
Create a new directory for your project and navigate into it:
mkdir MyJUnitProject cd MyJUnitProject
-
Initialize a Gradle project:
gradle init --type java-application
-
Your project structure should look like this:
MyJUnitProject/ ├── build.gradle ├── settings.gradle └── src └── main └── java └── (Your application code here)
Adding JUnit 5 Dependency
To perform tests with JUnit 5, you need to add it to your dependencies in build.gradle
. This is where the “one extra line” comes into play.
Step 1: Modify your build.gradle
File
Open your build.gradle
file and modify it to include the JUnit dependency. Here’s how you should structure it:
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
// JUnit 5 Dependency
testImplementation 'org.junit.jupiter:junit-jupiter:5.8.2'
}
test {
// This is the 'one extra line' you need!
useJUnitPlatform()
}
Explanation
-
testImplementation
: This line adds the JUnit 5 library as a dependency for your testing environment. -
useJUnitPlatform()
: This extra line is essential. Without it, Gradle won’t know to look for JUnit 5 tests. It defaults to JUnit 4, which can lead to frustrating issues where tests are discovered but not executed because they aren't recognized as JUnit 4 tests.
Creating a Sample Test Class
Now that your configuration is set up, it’s time to write a sample test. Create a new directory for your test cases:
mkdir -p src/test/java/com/example
Next, create a file named CalculatorTest.java
inside src/test/java/com/example
:
package com.example;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
Why This Code?
-
@Test
Annotation: This annotation indicates that the method is a test method. JUnit will recognize and run this method when executing tests. -
assertEquals
Method: This method checks whether the actual result matches the expected result. If they do not match, the test fails, and you can clearly see what went wrong.
Implementing the Calculator Class
Next, we need to implement a class called Calculator
. Create a new file named Calculator.java
in the src/main/java/com/example
directory:
package com.example;
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Rationale Behind the Code
- Simple Addition Method: In a real-world context, the logic can be much more complex. However, we keep it simple to focus on JUnit setup.
Running Your Tests
To run the tests you've just created, execute the following command in the terminal:
./gradlew test
Gradle will compile your project, run the tests, and display the results. If everything is set up correctly, you should see an output indicating that your test passed successfully.
The Closing Argument
Integrating JUnit 5 with Gradle can simplify your testing setup, but it requires careful attention to detail. The missing “one extra line” of useJUnitPlatform()
in the test {}
block is essential for telling Gradle to recognize JUnit 5 tests.
Additional Resources
For further reading on JUnit 5, check JUnit 5 Documentation and for advanced Gradle features, take a look at the Gradle User Manual.
By ensuring you have this simple setup in place, you can focus more on writing meaningful tests without the overhead of configuration issues, making your development process smoother and more efficient. Happy testing!
Checkout our other articles