Optimizing DevOps Configuration for Flexibility
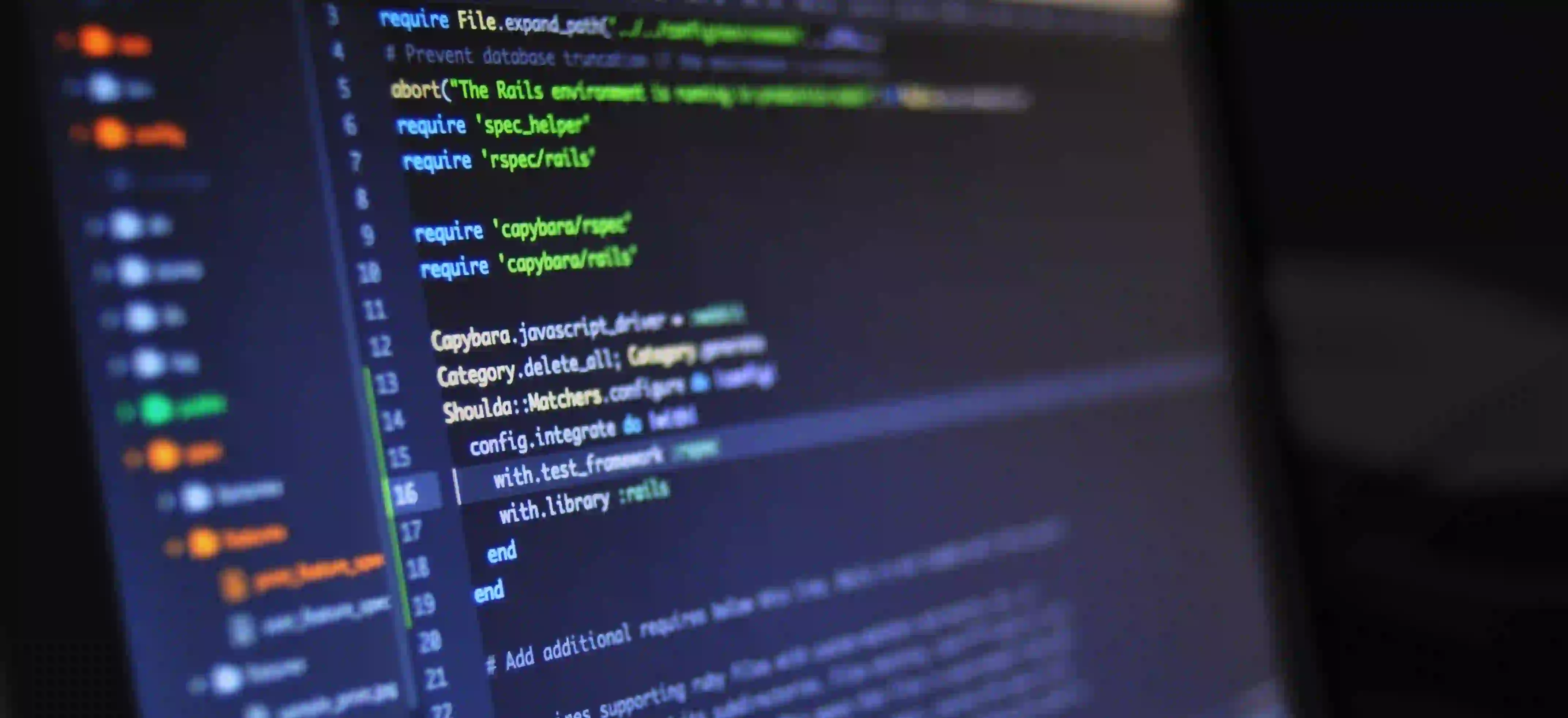
Optimizing DevOps Configuration for Flexibility
In the world of DevOps, flexibility is key. The ability to adapt to changing requirements and environments is crucial for successful software delivery. One way to achieve this flexibility is through the optimization of DevOps configuration. In this article, we will explore the importance of optimizing DevOps configuration for flexibility and demonstrate how Java can be utilized to achieve this goal.
The Role of Configuration in DevOps
Configuration in DevOps refers to the setup and customization of tools, environments, and processes to facilitate the seamless delivery of software. This includes configuration management, infrastructure as code, continuous integration/continuous deployment (CI/CD) pipelines, and more. An optimized configuration enables teams to respond to changes quickly, maintain consistency across environments, and minimize errors in the software delivery process.
Challenges in DevOps Configuration
DevOps configurations often face numerous challenges, including the need to support different environments (development, testing, production), manage dependencies, handle dynamic scalability, and incorporate security measures. These challenges necessitate a flexible and robust configuration approach that can adapt to diverse requirements without introducing complexity.
Using Java for Configuration Flexibility
Java, with its strong ecosystem and versatile features, can play a significant role in optimizing DevOps configuration for flexibility. Let's delve into some key areas where Java can be leveraged for this purpose.
Configuration Management with Java Properties
In Java, properties files provide a simple and flexible way to manage configuration. By using key-value pairs, properties files can encapsulate environment-specific settings, such as database connection details, API endpoints, feature toggles, and more. Here's an example of a Java properties file:
# config.properties
db.url=jdbc:mysql://localhost:3306/mydb
db.username=admin
db.password=secret
Using Java's Properties
class, we can load and access these configurations within our code:
Properties properties = new Properties();
try (InputStream input = new FileInputStream("config.properties")) {
properties.load(input);
String dbUrl = properties.getProperty("db.url");
String dbUsername = properties.getProperty("db.username");
String dbPassword = properties.getProperty("db.password");
} catch (IOException ex) {
// Handle configuration loading failure
}
By utilizing properties files and the Properties
class, we can easily adapt configuration settings based on the target environment without modifying the code, thus enhancing flexibility.
Externalizing Configuration with Spring Boot
Spring Boot, a popular Java framework, offers robust support for externalized configuration. Through property files, YAML files, environment variables, and command-line arguments, Spring Boot allows for flexible setup of application properties. Additionally, Spring Cloud Config provides centralized external configuration management for distributed systems, further enhancing flexibility and scalability.
Here's an example of a Spring Boot application.properties
file:
# application.properties
server.port=8080
logging.level.org.springframework=DEBUG
Spring Boot's auto-configuration and property binding capabilities enable seamless adaptation of configurations, making it an ideal choice for flexible DevOps setups.
Templating Configuration with Apache FreeMarker
Apache FreeMarker is a powerful Java template engine that allows for the generation of configuration files from templates. By using FreeMarker, we can define reusable configuration templates with placeholders and dynamically populate them based on environment-specific variables.
Consider the following FreeMarker template for an Nginx configuration:
# nginx.conf.ftl
server {
listen ${nginx.port};
server_name ${nginx.serverName};
location / {
proxy_pass ${nginx.upstream};
}
}
With FreeMarker, we can substitute placeholders with actual values based on the deployment environment, enabling flexible and consistent configuration management.
Leveraging Configuration Libraries
Java offers a myriad of configuration libraries and frameworks, such as Apache Commons Config, Typesafe Config, and JOOQ. These libraries provide advanced features for handling configuration files, environment variables, hierarchical configurations, and more. By integrating such libraries into DevOps workflows, teams can achieve greater flexibility in managing configurations across diverse environments and deployment scenarios.
Final Thoughts
Optimizing DevOps configuration for flexibility is pivotal in enabling agile and resilient software delivery pipelines. By harnessing the capabilities of Java, including properties files, Spring Boot, FreeMarker, and configuration libraries, teams can achieve a high degree of adaptability in their DevOps setups. Embracing flexible configuration practices empowers organizations to respond to changing requirements, mitigate operational complexities, and deliver high-quality software with confidence.
In conclusion, Java serves as a formidable ally in the quest for flexible DevOps configuration, propelling software delivery towards greater adaptability and efficiency.
By embracing flexible configuration practices, organizations can respond to changing requirements, mitigate operational complexities, and deliver high-quality software with confidence (link to DevOps flexibility article). If you are interested in digging deeper into the Java framework, you can explore additional resources on Java documentation.
Start optimizing your DevOps configuration for flexibility with Java today!