Common Pitfalls When Using Google Gson
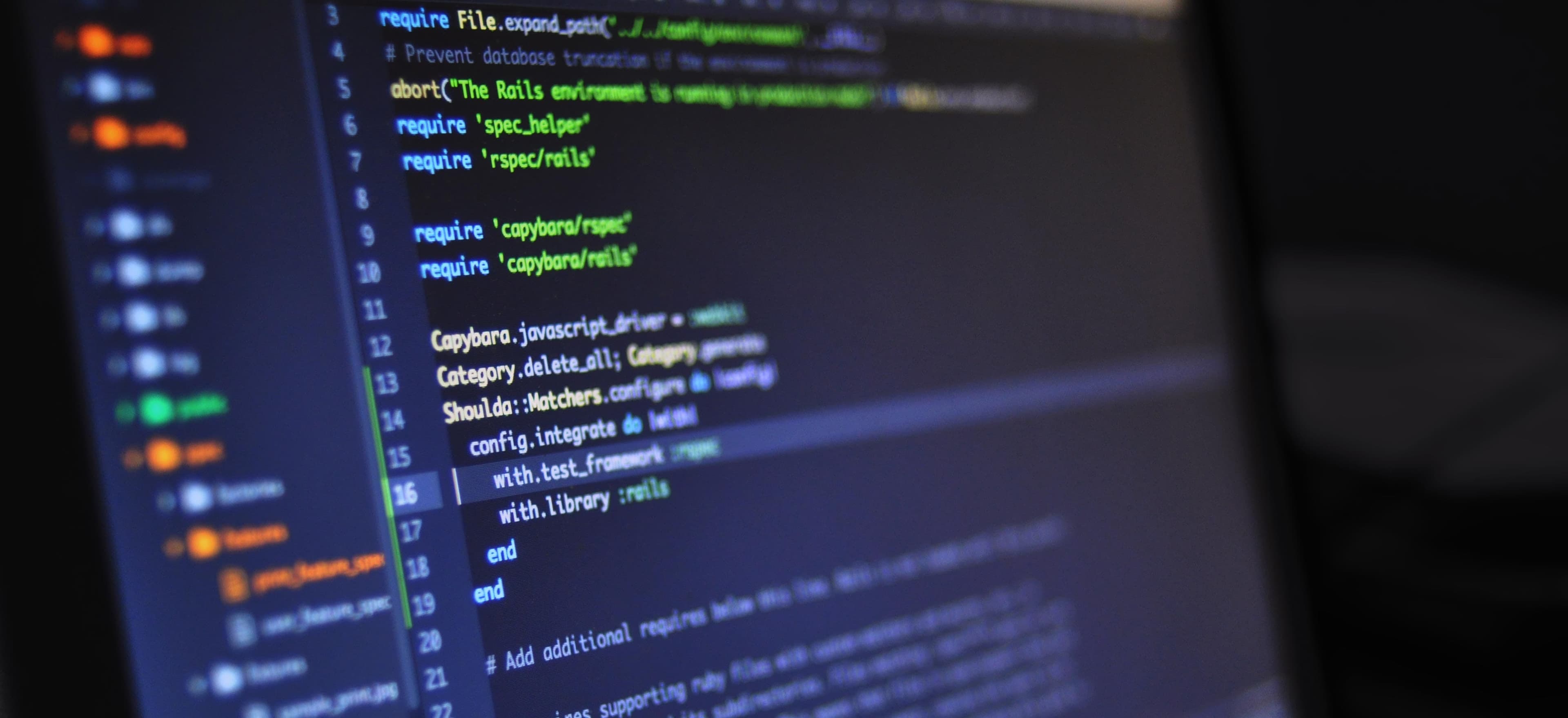
- Published on
Common Pitfalls When Using Google Gson
When working with Java, you may often come across the need to serialize and deserialize objects to and from JSON. One popular library for this task is Google Gson. While Gson is a powerful and convenient tool, there are several common pitfalls that developers may encounter when using it. In this post, we'll discuss some of these pitfalls and how to avoid them.
Pitfall 1: Handling Nested Objects
One common mistake when using Gson is not properly handling nested objects. Consider the following JSON:
{
"name": "John Doe",
"age": 30,
"address": {
"street": "123 Main St",
"city": "Anytown"
}
}
To deserialize this JSON into a Java object, you might have classes like:
class Person {
private String name;
private int age;
private Address address;
}
class Address {
private String street;
private String city;
}
When deserializing the JSON using Gson, you need to ensure that the Address
object within the Person
object is correctly populated. This is achieved by using @SerializedName
annotation or custom deserialization with TypeAdapter
.
class Person {
private String name;
private int age;
@SerializedName("address")
private Address personAddress;
// getter and setter methods
}
class Address {
private String street;
private String city;
// getter and setter methods
}
Pitfall 2: Dealing with Collections
Another pitfall is handling collections of objects. Let's say you have JSON like this:
{
"name": "John Doe",
"hobbies": ["hiking", "reading", "swimming"]
}
To deserialize this JSON, you might have a Person
class with a list of hobbies:
class Person {
private String name;
private List<String> hobbies;
// getter and setter methods
}
When using Gson to deserialize this JSON, ensure that you properly handle the type of the collection by specifying the type when using TypeToken
.
Type listType = new TypeToken<List<String>>(){}.getType();
List<String> hobbies= gson.fromJson(json, listType);
Pitfall 3: Dealing with Default Values
When deserializing JSON into Java objects, it's essential to consider default values. If a field in the JSON is missing, Gson will set the corresponding field in the Java object to its default value (e.g., null
for objects, 0
for integers, false
for booleans). To handle this, you can use the @SerializedName
annotation and set a default value using the @SerializedName
annotation.
class Person {
private String name;
private int age;
@SerializedName("address")
private Address personAddress;
@SerializedName("isStudent")
private boolean isStudent = true; //default value
// getter and setter methods
}
Pitfall 4: Dealing with Unnecessary Fields
Sometimes, JSON may contain fields that you don't want to include in your Java object. To handle this, you can use @Expose
annotation to include or exclude fields during serialization and deserialization. This helps to maintain the integrity of your Java object's representation.
class Person {
@Expose
private String name;
@Expose(serialize = false)
private int age; //exclude age during serialization
// getter and setter methods
}
Pitfall 5: Handling Circular References
One often overlooked pitfall is dealing with circular references. If your JSON contains circular references (e.g., A referencing B, and B referencing A), Gson may throw a StackOverflowError
during deserialization. To address this, you can use @Expose(deserialize = false)
to break the circular reference during deserialization.
class Person {
@Expose
private String name;
@Expose
private List<Person> friends;
// getter and setter methods
}
Wrapping Up
While Google Gson is a powerful library for JSON serialization and deserialization in Java, it's essential to be mindful of these common pitfalls. By understanding and addressing these pitfalls, you can maximize the efficiency and robustness of your JSON handling in Java.
To learn more about Gson and JSON processing in Java, check out the official Gson documentation and the Java JSON Processing API.
Remember, with great power (of Gson) comes great responsibility (for handling JSON in Java)!