Efficient Implementation of Spring WebFlux with Kotlin
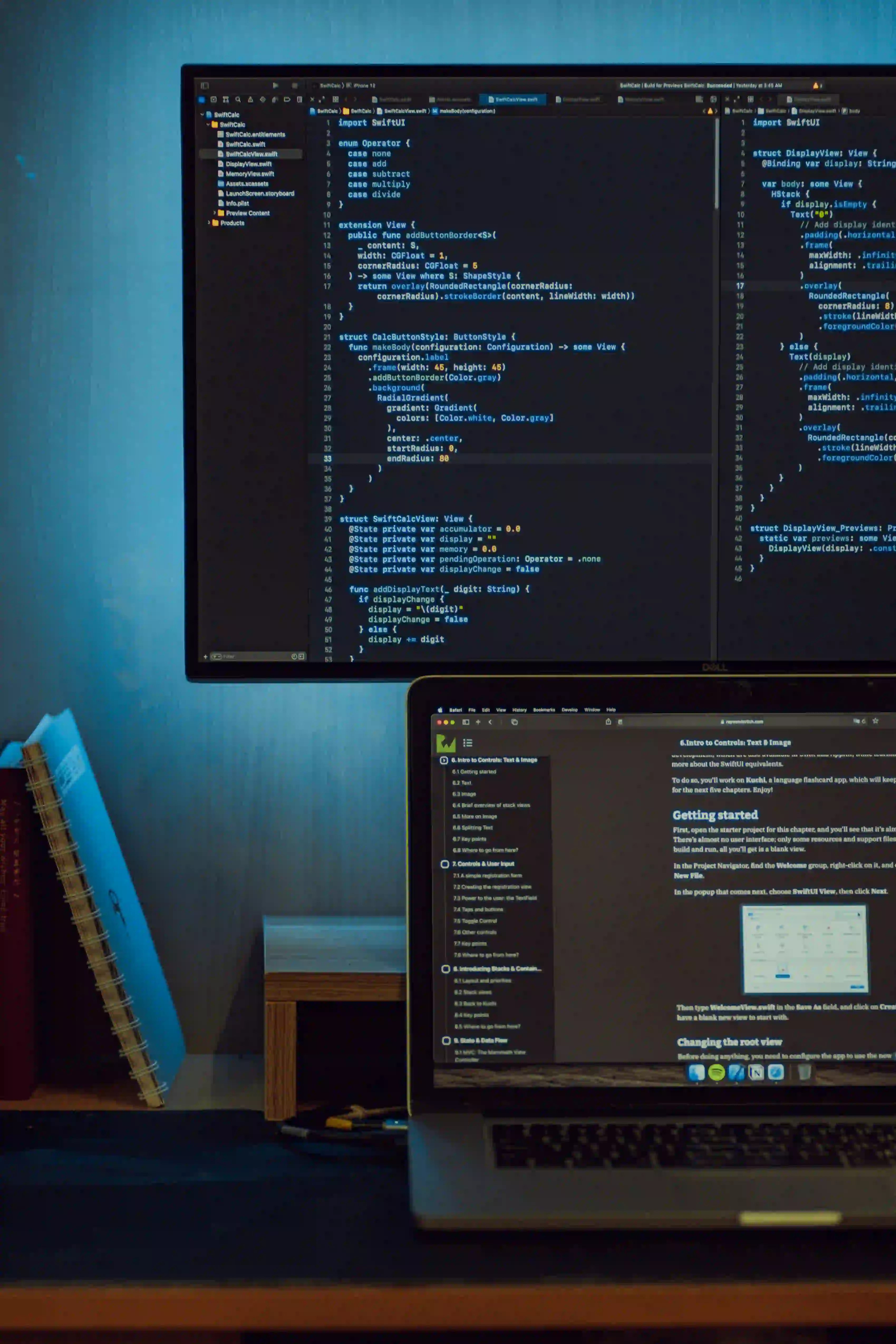
Efficient Implementation of Spring WebFlux with Kotlin
In recent years, there has been a significant shift towards reactive programming in the world of Java development. With the advent of non-blocking, asynchronous programming models, developers have been able to create highly scalable and efficient applications. One of the popular frameworks that has emerged in this context is Spring WebFlux, which provides reactive programming support for building web applications.
In this blog post, we will explore the efficient implementation of Spring WebFlux with Kotlin. We will delve into the key concepts of reactive programming, discuss the benefits of using Spring WebFlux, and provide practical examples to demonstrate its implementation with Kotlin.
Understanding Reactive Programming
Reactive programming is an asynchronous programming paradigm focused on data streams and the propagation of changes. It allows developers to build applications that can handle a large number of concurrent connections and process high volumes of data with low latency.
At the core of reactive programming are the following key components:
- Publisher: Emits data streams.
- Subscriber: Consumes the data emitted by the Publisher.
- Processor: Represents a processing stage, which can act as both a Publisher and a Subscriber.
With reactive programming, developers can define pipelines for processing data streams, where each step in the pipeline operates independently and asynchronously. This enables efficient resource utilization and improved responsiveness in applications.
Advantages of Spring WebFlux
Spring WebFlux is a part of the Spring Framework that provides support for reactive programming. It offers a non-blocking, reactive approach to building web applications, which is particularly well-suited for handling I/O-intensive operations.
Some of the key advantages of using Spring WebFlux include:
- Scalability: Asynchronous, non-blocking operations enable efficient handling of a large number of concurrent requests, making applications highly scalable.
- Performance: By leveraging reactive programming, Spring WebFlux can deliver better performance, particularly in scenarios involving I/O-bound operations.
- Functional Endpoints: Spring WebFlux supports functional programming style for defining web endpoints, providing a more concise and expressive way of handling HTTP requests.
Implementing Spring WebFlux with Kotlin
Now, let's dive into the actual implementation of Spring WebFlux with Kotlin. We will walk through the process of setting up a simple web application that exposes reactive endpoints using Spring WebFlux.
Setting up the Project
To get started, we need to initialize a new Spring Boot project with Kotlin and include the necessary dependencies for Spring WebFlux. We can use tools like Spring Initializr or manually configure the project with Gradle or Maven.
// build.gradle.kts
plugins {
id("org.springframework.boot") version "2.6.3"
id("io.spring.dependency-management") version "1.0.11.RELEASE"
kotlin("jvm") version "1.5.31"
kotlin("plugin.spring") version "1.5.31"
}
repositories {
mavenCentral()
}
dependencies {
implementation("org.springframework.boot:spring-boot-starter-webflux")
implementation("org.jetbrains.kotlin:kotlin-reflect")
implementation("org.jetbrains.kotlin:kotlin-stdlib-jdk8")
testImplementation("org.springframework.boot:spring-boot-starter-test")
}
In the build file, we have added the spring-boot-starter-webflux
dependency to enable Spring WebFlux support in our project. Additionally, we have included the Kotlin dependencies required for our Kotlin-based project.
Creating a Reactive Endpoint
Next, let's create a simple reactive endpoint that returns a stream of data. We can define a functional endpoint using Kotlin's concise syntax and leverage the reactive nature of Spring WebFlux.
// MainController.kt
import org.springframework.web.reactive.function.server.*
import org.springframework.context.annotation.Bean
import org.springframework.context.annotation.Configuration
import reactor.core.publisher.Flux
@Configuration
class MainRouter {
@Bean
fun route(): RouterFunction<ServerResponse> {
return RouterFunctions.route(RequestPredicates.GET("/data"), HandlerFunction {
ServerResponse.ok().body(
Flux.just("Hello", "World", "with", "Spring", "WebFlux"),
String::class.java
)
})
}
}
In the above example, we have defined a MainRouter
class as a configuration bean, where we have declared a route for handling GET requests to /data
. When a request is made to this endpoint, it returns a stream of strings as a reactive Flux
.
Running the Application
With the project set up and the reactive endpoint in place, we can run the Spring Boot application and test the reactive behavior of our endpoint. We can use tools like cURL, Postman, or simply a web browser to make a GET request to the /data
endpoint and observe the stream of data being returned.
$ ./gradlew bootRun
Once the application is up and running, we can make a GET request to http://localhost:8080/data
and see the reactive stream in action.
A Final Look
In this blog post, we have explored the efficient implementation of Spring WebFlux with Kotlin, leveraging the power of reactive programming to build scalable and efficient web applications. We discussed the key concepts of reactive programming, highlighted the advantages of using Spring WebFlux, and provided a practical example to demonstrate its implementation with Kotlin.
By embracing reactive programming paradigms and leveraging the capabilities of Spring WebFlux, developers can create highly responsive and performant web applications that are well-suited for modern use cases involving high concurrency and I/O-intensive operations.
Incorporating Spring WebFlux with Kotlin opens up a new realm of possibilities for building robust and efficient web applications, and it is definitely worth considering for developers looking to embrace the principles of reactive programming in their projects.
In conclusion, the combination of Spring WebFlux and Kotlin presents a compelling option for developing reactive, high-performance web applications, and understanding the intricacies of their efficient implementation is key to leveraging their full potential.
To delve deeper into the world of reactive programming and Spring WebFlux, I highly recommend checking out the official Spring WebFlux documentation and exploring the vast resources available for learning Kotlin's intricacies. Through further exploration and hands-on experience, one can truly master the art of building robust, reactive applications with Spring WebFlux and Kotlin.