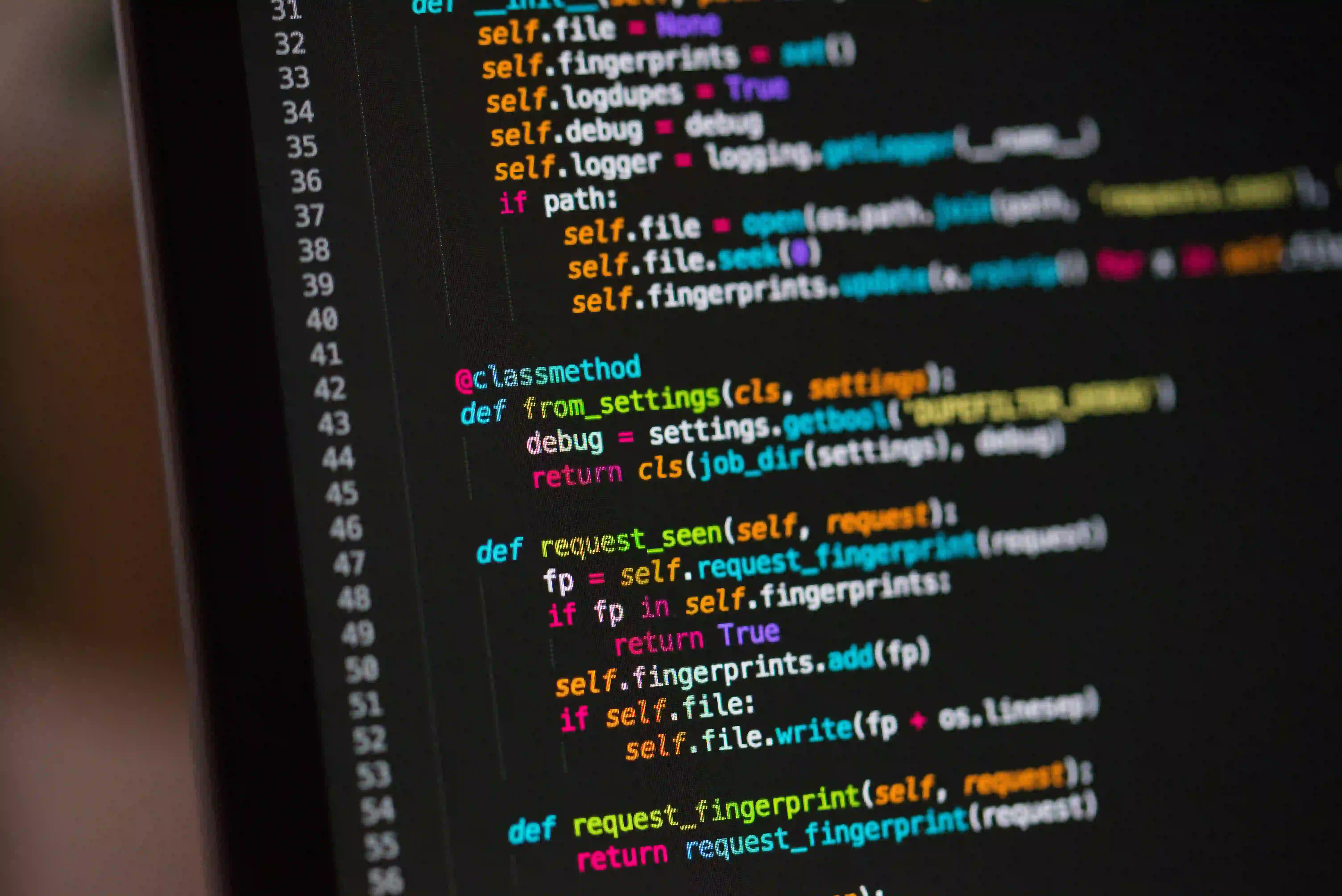
Visualizing Test Coverage in Eclipse: Identifying Uncovered Code
In software development, ensuring adequate test coverage is crucial for identifying potential bugs and ensuring the reliability and stability of the codebase. While writing unit tests is essential, it's equally important to ascertain how much of the code is actually exercised by these tests. This is where test coverage tools come into play, providing developers with insights into which parts of the code are covered by tests and which remain untested.
In this blog post, we'll explore how to visualize test coverage in Eclipse using the EclEmma plugin, and specifically focus on identifying uncovered code to prioritize test writing efforts.
Why Test Coverage Matters
Before we delve into the practical aspects, let's briefly discuss why test coverage is important. Test coverage analysis helps in:
- Identifying Untested Code: It reveals areas of the codebase that are not covered by tests, signaling potential blind spots where bugs might lurk.
- Improving Code Quality: By highlighting uncovered code, developers can focus their testing efforts on the most critical and error-prone parts of the software.
- Maintaining and Refactoring Code: When making changes to the codebase, test coverage analysis ensures that existing functionality is not inadvertently broken.
Now, let's jump into Eclipse and see how we can use EclEmma to visualize test coverage and pinpoint uncovered code.
Setting Up EclEmma in Eclipse
First, ensure that you have Eclipse IDE installed. If not, you can download Eclipse from the official website and follow the installation instructions.
Once Eclipse is up and running, follow these steps to set up EclEmma:
-
Install EclEmma Plugin: In Eclipse, navigate to
Help -> Eclipse Marketplace
and search for "EclEmma". Click on the "Install" button to add the plugin to your IDE. -
Restart Eclipse: After the installation is complete, restart Eclipse to activate the EclEmma plugin.
With EclEmma successfully installed, we can now proceed to visualize the test coverage of our Java code.
Visualizing Test Coverage with EclEmma
Let's consider a simple Java class representing a mathematical function - MathUtils.java
. We'll write a few unit tests for this class and then use EclEmma to visualize the test coverage.
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
public static int subtract(int a, int b) {
return a - b;
}
}
For the sake of demonstration, here are the corresponding JUnit tests:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class MathUtilsTest {
@Test
public void testAdd() {
assertEquals(4, MathUtils.add(2, 2));
}
@Test
public void testSubtract() {
assertEquals(3, MathUtils.subtract(5, 2));
}
}
Now, let's run the tests and visualize the coverage using EclEmma:
-
Run JUnit Tests: Right-click on the project containing
MathUtils
and selectRun As -> JUnit Test
to execute the unit tests. -
View Coverage Report: After the tests have run, right-click on the project again and choose
Coverage As -> Coverage
. This will generate a coverage report using EclEmma.
The visual representation provided by EclEmma will highlight the covered and uncovered lines of code in the MathUtils
class. Any lines not exercised by the tests will be clearly identified, allowing us to prioritize our testing efforts on these areas.
Identifying Uncovered Code
EclEmma's coverage report not only gives an overview of the test coverage but also specifically pinpoints the uncovered lines of code. Uncovered code is marked distinctly, making it easy for developers to identify and focus on these areas when writing additional tests.
Here's an example of how uncovered code might be highlighted in EclEmma's coverage report:
public class MathUtils {
public static int add(int a, int b) {
return a + b; // Covered
}
public static int subtract(int a, int b) {
return a - b; // Uncovered
}
}
In this example, the line containing the subtraction operation in the subtract
method is identified as uncovered. This directs our attention to the fact that this particular line of code is not being tested and needs to be addressed to achieve comprehensive test coverage.
By focusing on these uncovered areas, developers can systematically improve test coverage and fortify the robustness of the codebase.
To Wrap Things Up
Test coverage visualization plays a fundamental role in understanding the effectiveness of our testing efforts and identifying gaps in the coverage. EclEmma, with its seamless integration into Eclipse, provides developers with a powerful tool to gain insights into their codebase's test coverage and, more importantly, to pinpoint uncovered code for targeted testing.
In this blog post, we've walked through the process of setting up EclEmma in Eclipse, visualizing test coverage, and identifying uncovered code. By incorporating test coverage analysis into the development workflow, teams can enhance the overall quality and reliability of their software.
With the ability to visualize and identify uncovered code, developers are empowered to write targeted tests, resulting in more comprehensive test coverage and ultimately delivering more robust and stable software.
So, next time you're working on a Java project in Eclipse, consider leveraging the EclEmma plugin to gain invaluable insights into your test coverage and uncover those critical areas of untested code.
Happy testing!