Java Methods: Simplifying with Parameters Object
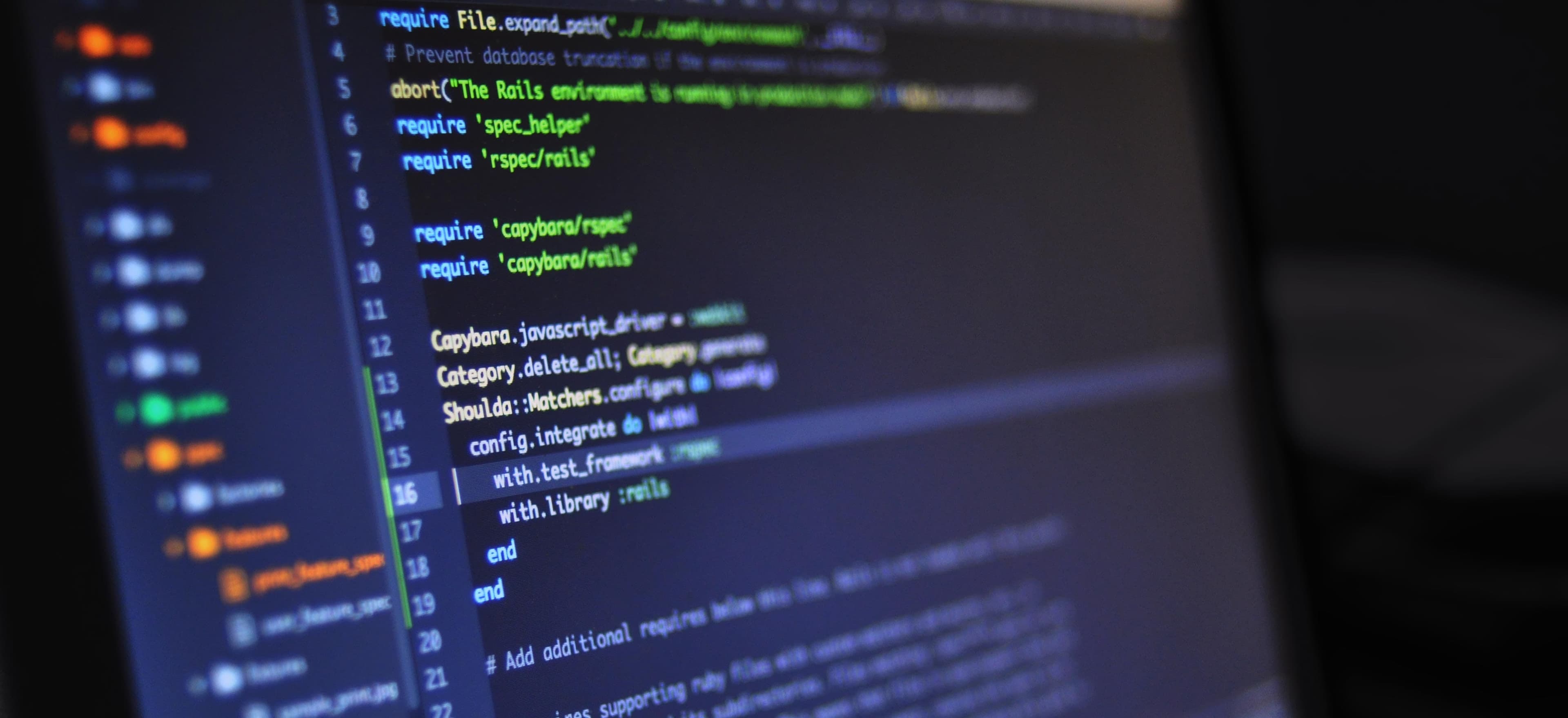
- Published on
Simplifying Java Methods with Parameters Object
When it comes to writing efficient and maintainable Java code, utilizing the right set of parameters can make a world of difference. Excessive use of parameters can clutter the method signature and make the code difficult to read, understand, and maintain. In this post, we will delve into the concept of using a Parameters Object to simplify our Java methods, leading to cleaner and more manageable code.
The Problem with Too Many Parameters
Consider a scenario where you have a method that requires a long list of parameters. For example:
public void createOrder(String customerName, String customerEmail, String productName, int quantity, double price, boolean isExpressDelivery) {
// Method implementation
}
As the number of parameters grows, managing them becomes increasingly cumbersome. It becomes challenging to remember the order of parameters and their respective meanings, which can lead to errors and confusion. Moreover, if the method signature needs to be changed or extended in the future, it would require updating the method itself and all the calls to that method.
Introducing the Parameters Object
To address the issues associated with an excessive number of parameters, we can employ the Parameters Object pattern. This pattern entails encapsulating related parameters into a single object, thus simplifying the method signature and improving code readability.
Let's refactor the createOrder
method using a Parameters Object:
public class OrderParams {
private String customerName;
private String customerEmail;
private String productName;
private int quantity;
private double price;
private boolean isExpressDelivery;
// Getters and setters for the parameters
}
public void createOrder(OrderParams orderParams) {
// Method implementation using orderParams object
}
By introducing the OrderParam
class, we have consolidated all the parameters related to order creation into a single object. This not only declutters the method signature but also makes it easier to comprehend the inputs required for the createOrder
method.
Benefits of Using a Parameters Object
Improved Readability
The use of a Parameters Object enhances the readability of the method signature by providing a clear, self-descriptive parameter object instead of a lengthy list of individual parameters.
Simplified Maintenance
In case the structure of the parameters needs to be modified or extended, making changes to a single Parameters Object class is more straightforward than updating multiple methods and their respective calls.
Enhanced Flexibility
With a Parameters Object, it becomes easier to introduce new parameters without affecting the existing method signature, as the object can be easily extended to accommodate additional attributes.
Enhanced Testability
When writing unit tests, passing a single Parameters Object containing all the required inputs simplifies the test setup and improves the overall test readability.
Common Pitfalls and Best Practices
While leveraging a Parameters Object offers numerous advantages, there are certain pitfalls to be mindful of. It's crucial to follow best practices to maximize the benefits of this approach.
Designing Cohesive Parameter Objects
Ensure that the parameters encapsulated within the Parameters Object are cohesive and related to the functionality being performed by the method. Avoid creating overly general parameter objects that encompass disparate concerns.
Avoiding Object Proliferation
While using Parameters Objects can streamline method signatures, it's essential to strike a balance and avoid creating an excessive number of small, specialized parameter classes. Aim for a cohesive and concise design.
Managing Object State
Be cautious about the state of the Parameters Object. If the same Parameters Object instance is used across multiple method calls, changes to its state could lead to unexpected behavior. Consider immutability or defensive copying where applicable.
Providing Defaults and Validation
When using a Parameters Object, consider providing default values for optional parameters and incorporating validation logic within the Parameters Object to ensure the integrity of the input data.
When to Use Parameters Objects
The decision to employ Parameters Objects should be guided by the complexity and cohesion of the parameters being passed to a method. It is particularly beneficial in the following scenarios:
- Methods with multiple parameters, especially when some of them are optional
- Enhancing readability and maintainability of code
- Promoting a cohesive and expressive API for complex operations
On the other hand, for simple methods with a small, fixed number of closely related parameters, the use of a Parameters Object may introduce unnecessary complexity.
Bringing It All Together
In conclusion, leveraging Parameters Objects in Java methods can significantly contribute to code simplicity, maintainability, and readability. By encapsulating related parameters within a single object, we can streamline method signatures, reduce parameter clutter, and enhance the overall codebase. However, it's important to apply this pattern judiciously, keeping the cohesion of parameters and the overall codebase in mind.
So, the next time you find yourself dealing with a method signature that resembles a daunting list of parameters, consider employing the Parameters Object pattern to declutter and simplify your code.
Don't forget to share your thoughts or any experiences you've had with Parameters Objects in Java! And if you're looking to explore more about Java best practices, this article delves into various Java best practices for performance improvement.