Troubleshooting Common Scala Syntax Errors
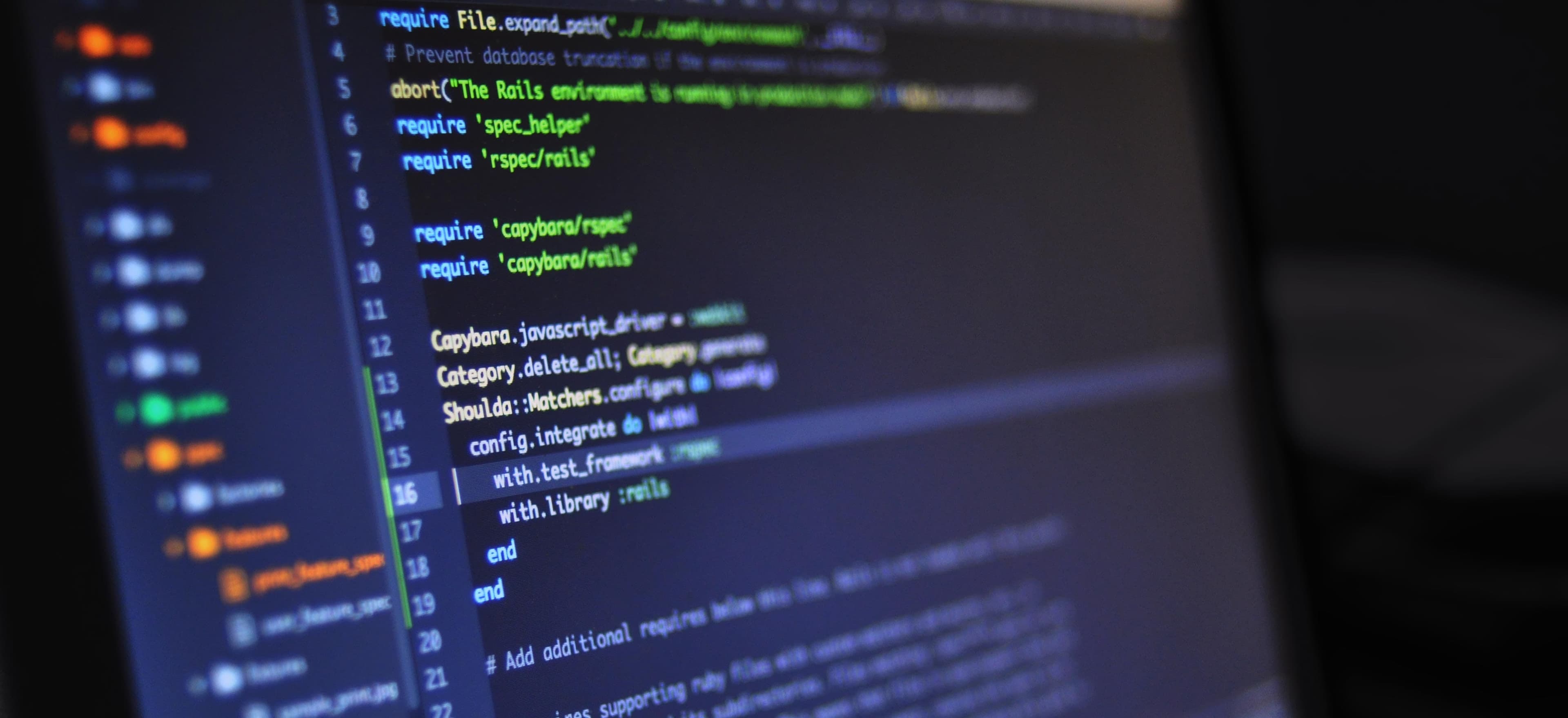
- Published on
Troubleshooting Common Scala Syntax Errors
Scala is a powerful programming language that runs on the Java Virtual Machine (JVM). It is known for its conciseness, expressiveness, and scalability. However, like any language, Scala is not free from syntax errors. In this post, we will explore some common Scala syntax errors and how to troubleshoot them effectively.
1. Missing Semicolon
One of the most common errors in Scala is the missing semicolon. Unlike Java, Scala does not require semicolons at the end of each statement. However, there are scenarios where the Scala parser might misinterpret the code due to missing semicolons.
For example, consider the following code snippet:
val x = 10
val y = 20
println(x + y)
The above code will result in a syntax error because the Scala parser will interpret it as val x = 10 val y = 20 println(x + y)
. To fix this, simply add semicolons after each statement:
val x = 10;
val y = 20;
println(x + y)
2. Incorrect Braces Usage
Another common syntax error in Scala is the incorrect usage of braces. In Scala, braces are used to define code blocks. If the braces are not used properly, it can lead to syntax errors.
Consider the following example:
if (x > 5)
println("x is greater than 5")
else
println("x is less than or equal to 5")
In this case, if you try to add another statement after the else
, you will encounter a syntax error. To fix this, enclose the if-else block within braces:
if (x > 5) {
println("x is greater than 5")
} else {
println("x is less than or equal to 5")
}
3. Uninitialized Variables
Scala requires all variables to be initialized at the time of declaration. Failure to do so will result in a syntax error.
Consider the following code snippet:
var x: Int
println(x)
The above code will result in a syntax error because the variable x
is not initialized. To fix this, simply initialize the variable x
:
var x: Int = 0
println(x)
4. Mismatched Types
Scala is a statically-typed language, which means that once a variable is declared with a certain type, it cannot be reassigned to a different type. Trying to reassign a variable to a different type will lead to a syntax error.
For example:
var x: Int = 10
x = "hello"
The above code will result in a syntax error because we are trying to assign a String
value to a variable declared as Int
. To fix this, ensure that the variable assignment matches the declared type:
var x: Int = 10
var y: String = "hello"
5. Incorrect Method Invocation
Scala allows the omission of dot and parentheses for method invocation in certain scenarios. However, this can lead to ambiguity and syntax errors.
Consider the following code:
val x = List(1, 2, 3)
x foreach println
In this case, the Scala parser will interpret println
as a function passed to foreach
, resulting in a syntax error. To fix this, use the appropriate syntax for method invocation:
val x = List(1, 2, 3)
x.foreach(println)
A Final Look
In conclusion, Scala, like any programming language, is prone to syntax errors. However, by being mindful of common pitfalls such as missing semicolons, incorrect braces usage, uninitialized variables, mismatched types, and incorrect method invocation, developers can effectively troubleshoot and address these issues. Mastering the nuances of Scala syntax is key to writing clean, error-free code.
Remember, practice makes perfect, and with time and experience, identifying and fixing syntax errors in Scala will become second nature.
I hope this guide has been helpful in highlighting some of the common Scala syntax errors and how to troubleshoot them. Happy coding!
For further reading about Scala syntax and best practices, check out official Scala documentation and Scala Style Guide.
Checkout our other articles