Optimizing Swing Performance for Java Desktop Applications
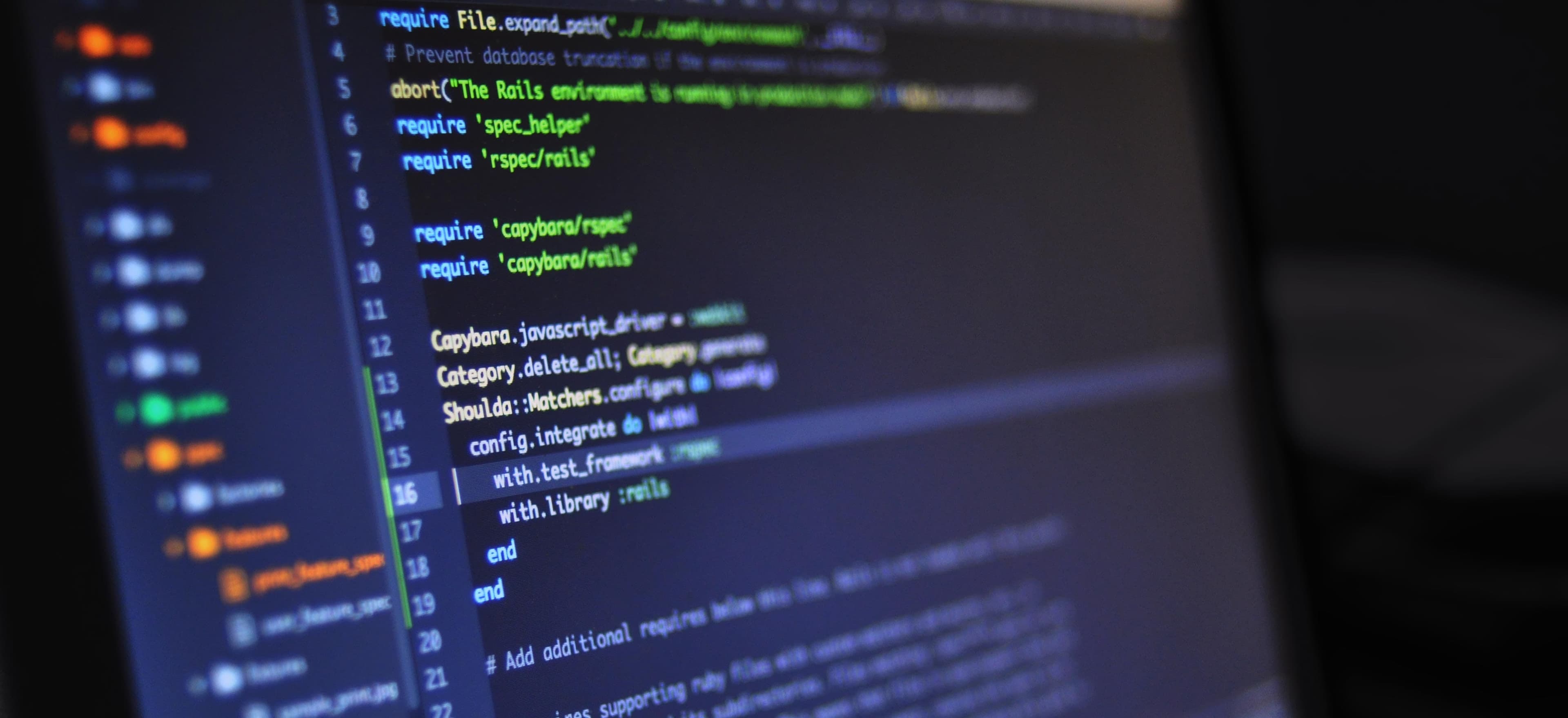
- Published on
Optimizing Swing Performance for Java Desktop Applications
Java desktop applications built using Swing provide a rich and flexible user interface. However, as the complexity of these applications grows, it's essential to ensure that they remain responsive and performant. In this article, we'll explore some best practices for optimizing the performance of Java desktop applications built with Swing.
Use Java Profiling Tools
Before diving into specific optimizations, it's crucial to identify performance bottlenecks in your Java desktop application. Java profiling tools like VisualVM, YourKit, or JProfiler can help pinpoint areas of your code that are causing slowdowns. These tools provide insights into memory usage, CPU utilization, and thread execution, allowing you to focus your optimization efforts where they are most needed.
Efficient Event Handling
One common source of performance degradation in Swing applications is inefficient event handling. When handling events such as mouse clicks or key presses, ensure that the event-handling code is optimized and responsive. Long-running event handlers can lead to unresponsive user interfaces. Consider offloading time-consuming tasks to background threads to keep the UI thread free to process user interactions.
button.addActionListener(e -> {
// Offload time-consuming task to background thread
SwingUtilities.invokeLater(() -> {
// Time-consuming task
});
});
Double Buffering for Smooth Rendering
Rendering performance is crucial for a responsive user interface. By default, Swing components use double buffering to reduce flickering and improve rendering performance. When custom painting components, ensure that double buffering is enabled to create smooth and flicker-free visual updates.
public class CustomComponent extends JComponent {
public CustomComponent() {
setDoubleBuffered(true); // Enable double buffering
}
}
Lightweight UI Components
Swing provides both heavyweight and lightweight components. Lightweight components generally offer better performance as they are rendered directly within the application window. When designing your user interface, prefer lightweight components such as JPanel
and JLabel
over heavyweight components like JScrollPane
whenever possible to improve rendering performance.
UI Threading Considerations
Swing applications are single-threaded by default, with all UI updates and event handling occurring on the Event Dispatch Thread (EDT). Lengthy tasks performed on the EDT can result in unresponsive UI. Utilize SwingWorker or CompletableFuture to execute long-running tasks asynchronously while keeping the UI responsive.
SwingWorker<Void, Void> worker = new SwingWorker<>() {
@Override
protected Void doInBackground() {
// Perform lengthy task in the background
return null;
}
};
worker.execute(); // Execute the SwingWorker
Layout Management
Effective layout management plays a significant role in the performance of Swing applications. Utilize layout managers such as FlowLayout
, BorderLayout
, or GridBagLayout
appropriately based on the components' positioning requirements. Avoid nesting multiple levels of complex layouts, as excessive nesting can lead to a degradation in rendering performance.
Efficient Resource Handling
Proper resource management is critical for the performance and stability of Java desktop applications. Be mindful of resource cleanup, especially when dealing with I/O operations, database connections, and native resources. Leaking resources can lead to memory leaks and degrade overall application performance over time.
Hardware Acceleration
Utilize hardware acceleration to offload graphical operations to the GPU where possible. This can be achieved by leveraging Java 2D's acceleration capabilities and using appropriate rendering hints. However, it's important to profile and test your application on a range of hardware configurations to ensure that accelerated rendering improves, rather than degrades, performance.
Key Takeaways
Optimizing the performance of Java desktop applications built with Swing is crucial for delivering a smooth and responsive user experience. By leveraging efficient event handling, double buffering, lightweight components, threaded execution, proper layout management, resource handling, and hardware acceleration, you can ensure that your Swing-based applications perform optimally. Remember to use Java profiling tools to identify performance bottlenecks and validate the impact of your optimizations.
Incorporating these best practices and continuously profiling and optimizing your Java desktop applications will result in a more responsive and efficient user interface, ultimately enhancing the overall user experience.
By implementing these techniques, your Java desktop applications built with Swing will not only look great but also perform exceptionally well, providing users with a seamless experience.
This article was brought to you by Oracle's official Java documentation.
Checkout our other articles