Mastering Application Instrumentation: Common Pitfalls to Avoid
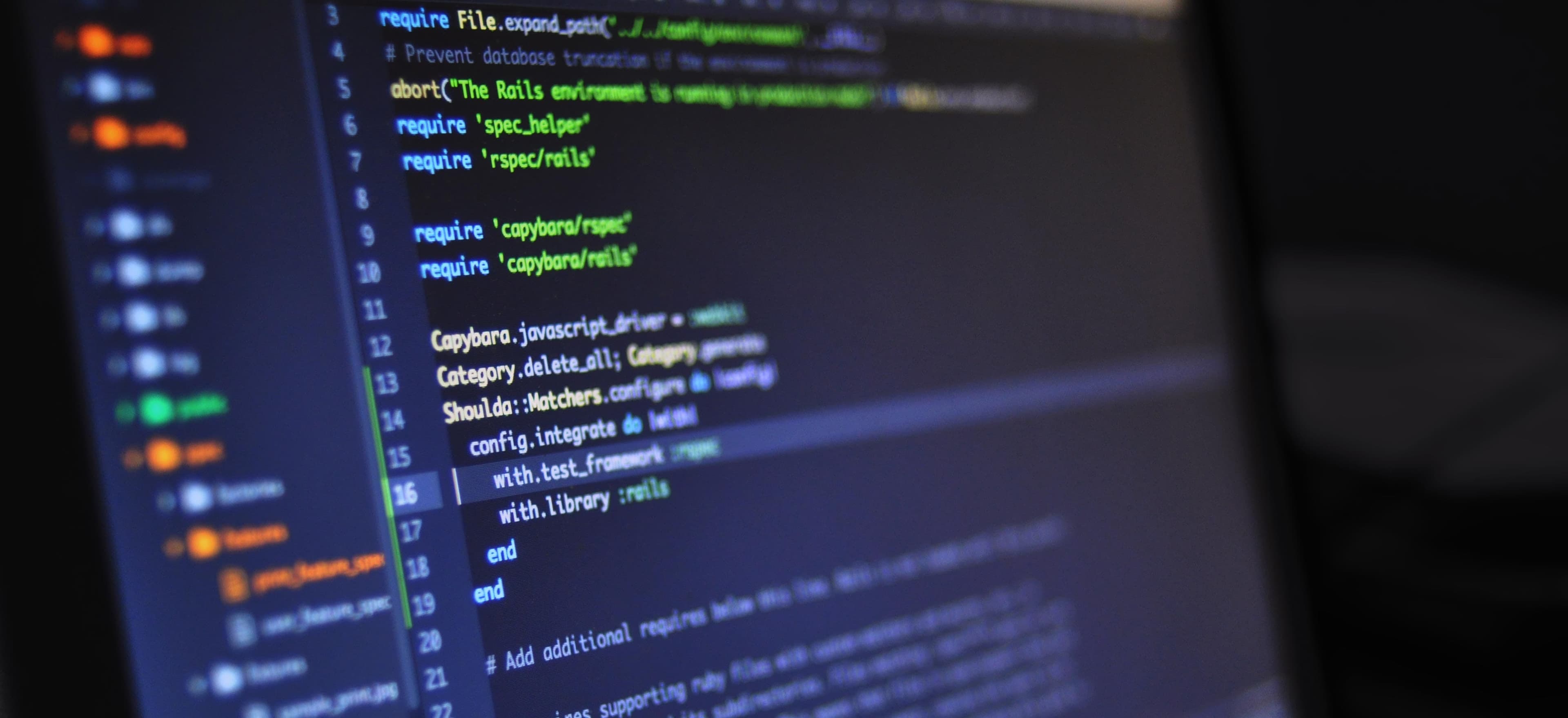
- Published on
Mastering Application Instrumentation: Common Pitfalls to Avoid
Application instrumentation is an essential part of software development that ensures efficient monitoring, debugging, and performance tuning. Whether you are building microservices, monoliths, or cloud-native applications, effective instrumentation allows you to gain insights into how your application behaves in production.
In this blog post, we will explore common pitfalls to avoid when it comes to application instrumentation. By addressing these traps, you can enhance your application’s performance, reliability, and maintainability. Let's dive in.
Understanding Application Instrumentation
Before we dive into the pitfalls, it’s crucial to understand what application instrumentation entails. Instrumentation refers to the process of adding monitoring and measurement capabilities to your code. It allows you to collect data, log events, and monitor system metrics.
In Java applications, this often involves using libraries or frameworks like Spring Boot Actuator, Micrometer, or Java Management Extensions (JMX). Each of these tools provides features that can help you capture performance data, health checks, and other vital application metrics.
- Spring Boot Actuator offers built-in endpoints that expose application metrics, health, and auditing information.
- Micrometer provides a flexible metrics facade, adding powerful telemetry features to your applications.
- JMX is a robust field instrument for monitoring Java applications and can be used for resource management.
Common Pitfalls in Application Instrumentation
1. Neglecting Contextual Information
One of the most common pitfalls in application instrumentation is the lack of contextual information in logs and metrics. Without context, it becomes difficult to analyze problems and correlate events.
Why it matters: Context helps identify the root cause of an issue. For example, if a log entry states "Error occurred," it does not give sufficient information. However, if the log also includes user ID, endpoint, request parameters, and timestamps, it becomes significantly useful.
Example of good logging:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserController {
private static final Logger logger = LoggerFactory.getLogger(UserController.class);
public void fetchUser(String userId) {
logger.info("Fetching user with ID: {}", userId);
// Implementation remains...
}
}
This example demonstrates how including user context in a log statement enhances its utility.
2. Over-Instrumentation
While instrumentation is critical, overdoing it can bog down your application. Adding too many metrics can lead to large volumes of data, making it harder to find valuable insights.
Why it matters: Excessive instrumentation can lead to increased overhead, affecting performance and storage. Choose metrics wisely and focus on business-critical use cases.
Best Practice: Use a MVP (Minimum Viable Product) approach to instrumentation. Start with essential metrics and assess their value before expanding your scope.
3. Ignoring Performance Impact
Every line of code has an associated cost. When it comes to instrumentation, the performance impact can be significant if not considered.
Why it matters: Instrumentation should not introduce latency into your application. If a metric collection takes too much time, it can affect user experience.
Example of non-blocking instrumentation:
import io.micrometer.core.instrument.MeterRegistry;
import io.micrometer.core.instrument.Counter;
public class OrderService {
private final Counter orderCounter;
public OrderService(MeterRegistry meterRegistry) {
this.orderCounter = meterRegistry.counter("orders.processed");
}
public void processOrder(Order order) {
// Non-blocking: Increment counter asynchronously
orderCounter.increment();
// Process the order here...
}
}
In this code snippet, the counter is incremented asynchronously, minimizing the impact on the processing time of an order.
4. Failing to Normalize Data
When collecting metrics, it's vital to ensure that the data is normalized. This means that similar events should be recorded in a consistent manner.
Why it matters: Normalized data is essential for accurate analysis and comparison over time. If metrics vary in naming or structure, it can lead to discrepancies in your reporting.
Example of normalized metrics:
import io.micrometer.core.instrument.MeterRegistry;
import io.micrometer.core.instrument.Tag;
public class PaymentService {
private final MeterRegistry meterRegistry;
public PaymentService(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
public void processPayment(Payment payment) {
meterRegistry.counter("payments.processed", "status", payment.getStatus().toString()).increment();
// Process the payment...
}
}
In this example, we consistently use the "payments.processed" metric, ensuring that all statuses are tracked uniformly.
5. Ignoring Security Concerns
Instrumentation can create security holes if sensitive data is logged. Careless handling of personal data can lead to serious compliance issues.
Why it matters: Data privacy regulations like GDPR or CCPA impose strict rules about data handling. Breaching these regulations can result in hefty fines.
Best Practice: Always avoid logging sensitive information. Here’s keeping sensitive data out of logs:
public class UserRegistrationService {
public void registerUser(User user) {
// Avoid logging sensitive user details
logger.info("User registration initiated for user: {}", user.getUsername());
// Implementation remains...
}
}
By logging only the username while avoiding sensitive information, the code adheres to best practices in security.
6. Not Leveraging Alerts and Dashboards
Instrumentation alone is not enough. Effective monitoring requires setting up alerts and dashboards that provide visibility into the health of your application.
Why it matters: Without a proper alerting system, you may miss critical issues, leading to outages or degraded user experiences.
Best Practice: Use tools like Grafana or Prometheus to visualize and alert. They can help you set thresholds and conditions for alerts based on the metrics collected.
Wrapping Up
Mastering application instrumentation is a powerful way to improve your software’s reliability, maintainability, and performance. By understanding the common pitfalls outlined in this article, you can ensure that your instrumentation strategy is effective and aligned with best practices.
- Neglecting Contextual Information can leave gaps in troubleshooting.
- Over-Instrumentation can lead to unnecessary complexity and performance degradation.
- Ignoring Performance Impact might affect user experience.
- Failing to Normalize Data hampers insightful analysis.
- Ignoring Security Concerns can lead to compliance issues.
- Not Leveraging Alerts and Dashboards can cause critical issues to go unnoticed.
With careful planning and execution of your instrumentation strategy, you can create a robust monitoring ecosystem that not only supports proactive maintenance but also enriches user experience.
By avoiding these common pitfalls, you can ensure that your application instrumentation strategy leads to meaningful insights and actionable data.
Further Resources
- For more details on solving performance issues, read about Optimizing Java Applications.
- Interested in practical metrics? Explore Micrometer Documentation.
By mastering application instrumentation, you lay down the groundwork for a high-performing, user-centric application. Happy coding!