Avoiding Getter Bloat in JavaBeans
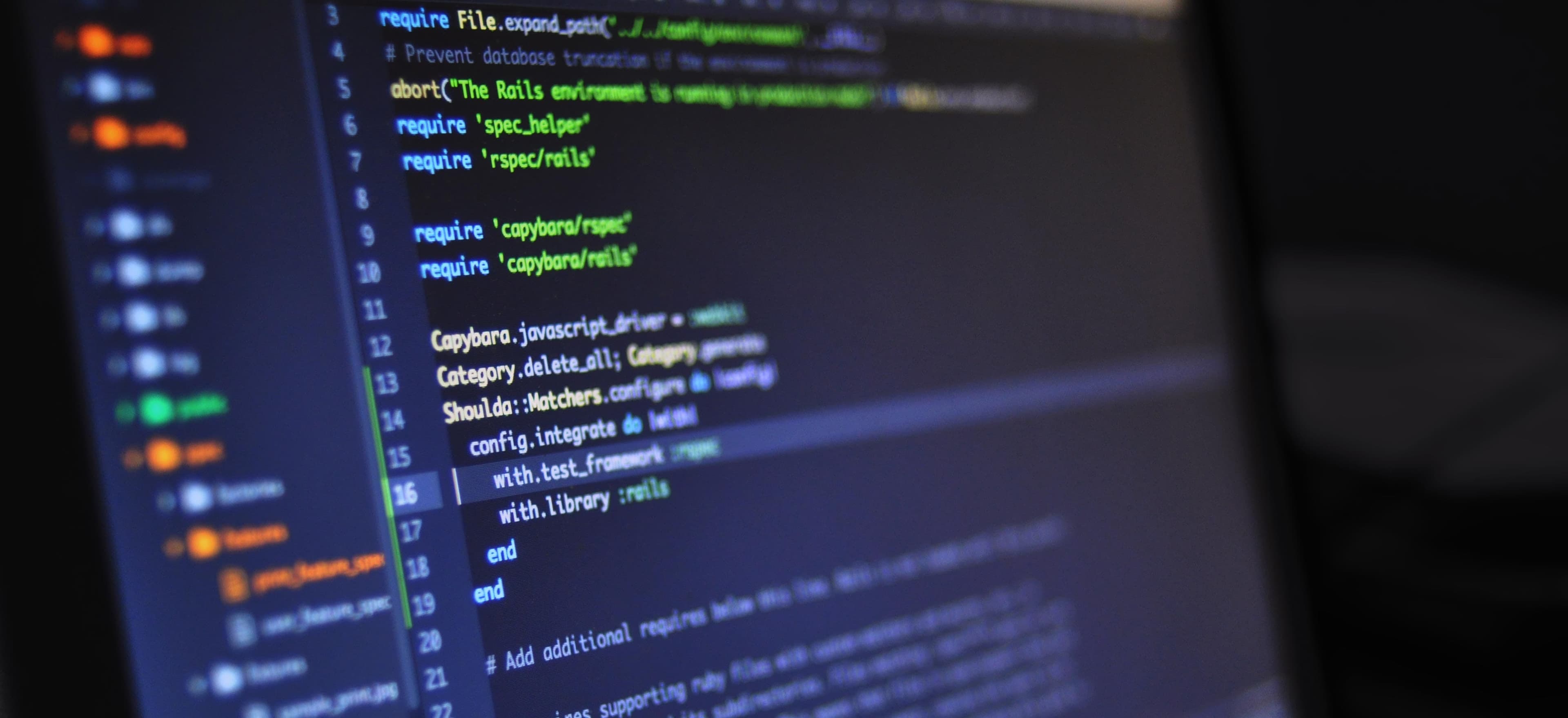
- Published on
In the world of Java programming, JavaBeans are a fundamental part of writing Java applications. JavaBeans are reusable software components that are manipulated visually in a builder tool. These components are defined by the JavaBeans specification, which outlines conventions for creating Java classes that follow specific naming patterns, have a default (no-argument) constructor, and are serializable.
When creating JavaBeans, it's common to use getters and setters to access and modify the properties of the bean. While getters and setters provide a clean way to access and update the fields of a Java class, they can lead to what is known as "getter bloat" when overused. Getter bloat refers to an excessive number of getters in a class, which can lead to bloated, difficult-to-maintain code.
In this article, we will explore the concept of getter bloat in JavaBeans, discuss its implications, and present strategies for avoiding it. We'll consider various techniques and patterns that can help streamline the process of working with JavaBeans while maintaining clean, concise code.
Understanding Getter Bloat
Getter bloat occurs when a JavaBean class has an excessive number of getters, often corresponding to each individual property of the bean. This can lead to verbose and cluttered code, making it harder to read, understand, and maintain. Consider a simple example:
public class Person {
private String firstName;
private String lastName;
private int age;
// Getters
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public int getAge() {
return age;
}
// Setters
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public void setAge(int age) {
this.age = age;
}
}
In this example, the Person
class has a separate getter method for each property (firstName
, lastName
, and age
). While this approach adheres to JavaBeans conventions, it can lead to repetitive code and decreased maintainability as the number of properties grows.
The Impact of Getter Bloat
Getter bloat can have several negative impacts on code quality and maintenance:
1. Code Verbosity
Excessive getters can make the codebase more verbose and harder to navigate, especially in classes with a large number of properties.
2. Readability
A high number of getters can reduce the overall readability of the code, making it more challenging for developers to understand the class interface quickly.
3. Maintenance
As the number of properties in a class increases, so does the amount of boilerplate code involved in creating and maintaining individual getters and setters.
4. Code Duplication
Boilerplate code for getters and setters can lead to duplication, potentially violating the DRY (Don't Repeat Yourself) principle.
Given these implications, it's crucial to explore strategies for mitigating getter bloat in JavaBeans. Let's examine some effective approaches to tackle this issue.
Strategies to Avoid Getter Bloat
1. Encapsulate Related Functionality with Custom Methods
Rather than exposing every internal property via a getter, consider encapsulating related functionality into cohesive methods. This approach can improve the clarity and intention of the class while reducing the number of individual getters.
For instance, instead of having separate getters for firstName
and lastName
, one can create a getFullName
method:
public String getFullName() {
return firstName + " " + lastName;
}
This way, the class interface remains concise, and the logic for combining first and last names is encapsulated within a single method.
2. Use Property Accessors (Lombok, Kotlin, etc.)
Leverage libraries such as Lombok or languages like Kotlin that provide concise syntax for defining properties. Lombok's @Data
annotation, for example, automatically generates getters and setters for all fields in a class, significantly reducing boilerplate code.
import lombok.Data;
@Data
public class Person {
private String firstName;
private String lastName;
private int age;
}
Using such libraries or languages can drastically reduce getter bloat by eliminating the need for manually defining individual getters and setters.
3. Consider the Builder Pattern
For classes with a large number of properties, especially those requiring complex instantiation, consider using the Builder pattern. This pattern allows for fluent construction of objects while minimizing the need for excessive getters and setters.
public class PersonBuilder {
private String firstName;
private String lastName;
private int age;
public PersonBuilder withFirstName(String firstName) {
this.firstName = firstName;
return this;
}
public PersonBuilder withLastName(String lastName) {
this.lastName = lastName;
return this;
}
public PersonBuilder withAge(int age) {
this.age = age;
return this;
}
public Person build() {
return new Person(firstName, lastName, age);
}
}
By employing the Builder pattern, the class interface remains clean, and the construction of objects becomes more expressive and less cluttered.
4. Embrace Functional Programming Constructs
Incorporate functional programming concepts where appropriate, such as using java.util.function.Function
to represent transformation operations on object properties. This approach can help reduce the number of individual getters and provide a more declarative style for working with properties.
5. Evaluate the Need for Every Property
Before adding a new property and its corresponding getter, carefully evaluate whether it's essential for external access. In many cases, not every internal property needs to be exposed via a getter, and an internal representation may suffice.
The Closing Argument
In conclusion, while getters and setters are integral parts of JavaBeans, excessive getter bloat can lead to code verbosity, reduced maintainability, and decreased readability. By adopting strategies such as encapsulating related functionality, leveraging libraries like Lombok, embracing the Builder pattern, and evaluating the necessity of each property, developers can effectively mitigate getter bloat in JavaBeans.
It's important to strike a balance between adhering to JavaBeans conventions and maintaining clean, concise code. With careful consideration and thoughtful design, it's possible to create JavaBeans that are both compliant and maintainable, enhancing the overall quality of Java applications.
In the ever-evolving landscape of Java development, staying mindful of getter bloat and employing effective strategies to mitigate its impact is crucial for building robust, maintainable codebases and fostering a positive developer experience.
Happy coding!