Optimizing Apache Camel Quarkus for 100-Camel Workflow
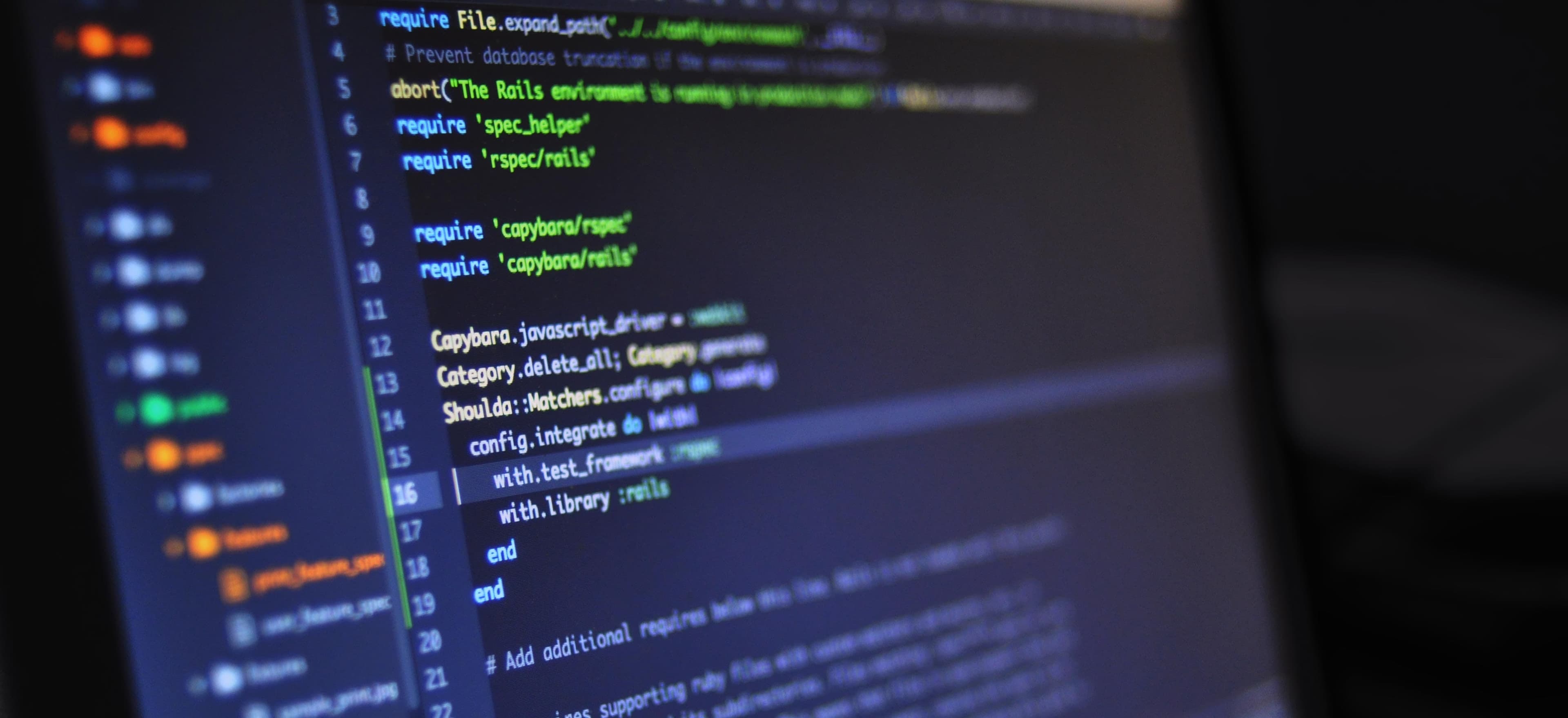
- Published on
Optimizing Apache Camel Quarkus for 100-Camel Workflow
Apache Camel Quarkus is an integration framework that provides a lightweight, fast, and efficient platform for building microservices and serverless applications. In this blog post, we will explore how to optimize Apache Camel Quarkus for a 100-Camel workflow, discussing various strategies, best practices, and code examples to achieve optimal performance.
Understanding the 100-Camel Workflow
Before diving into optimization, let's first understand what we mean by a 100-Camel workflow. In this context, the term "100-Camel" signifies a scenario where a large number of Apache Camel routes are coexisting within an application. Such a scenario can pose challenges related to memory consumption, startup time, and overall performance.
Optimizing Startup Time and Memory Consumption
One of the primary concerns when dealing with a 100-Camel workflow is the startup time of the application and its memory consumption. Apache Camel Quarkus provides several features and optimization techniques to address these concerns.
Using Static Configuration
One way to optimize startup time is by using static configuration wherever possible. This can be achieved by configuring Camel routes using properties or YAML files instead of dynamically loading configuration from external sources. By doing so, the application can initialize faster as it does not need to retrieve configuration dynamically.
from("timer:foo?period=5000")
.setBody().constant("Static Data")
.to("log:foo");
In the above Camel route, the configuration for the timer endpoint is static, thereby reducing the startup time.
Minimizing Dependencies
Another optimization technique is to minimize the number of dependencies used by the application. This can be achieved by carefully selecting and including only the required Camel components and libraries. Unnecessary dependencies can contribute to increased memory consumption and longer startup times.
Implementing Lazy Initialization
Apache Camel Quarkus supports lazy initialization of routes and components, allowing them to be initialized only when they are first used. This can significantly reduce the startup time, especially in scenarios with a large number of Camel routes.
from("direct:start")
.routeId("lazyRoute")
.startupOrder(5)
.to("log:lazyRoute");
In the above example, the lazyRoute
is configured to initialize lazily, improving the startup performance of the application.
Performance Tuning for 100-Camel Workflow
In addition to optimizing startup time and memory consumption, performance tuning is crucial for ensuring efficient execution of a 100-Camel workflow.
Parallel Processing
When dealing with a large number of Camel routes, leveraging parallel processing can greatly improve the overall performance. Apache Camel provides the multicast
EIP (Enterprise Integration Pattern) for parallel processing of messages.
from("direct:start")
.multicast()
.to("direct:route1", "direct:route2", "direct:route3");
In the above example, messages sent to the direct:start
endpoint will be processed in parallel by route1
, route2
, and route3
, leading to improved throughput.
Throttling
Throttling is a technique used to limit the rate of message processing to prevent overloading downstream systems. In a 100-Camel workflow, it's essential to implement throttling to ensure optimal utilization of resources and prevent resource exhaustion.
from("direct:start")
.throttle(10)
.timePeriodMillis(1000)
.asyncDelayed()
.to("direct:throttledRoute");
In the above example, the throttle
EIP is used to limit the rate of message processing to 10 messages per second, preventing excessive load on the downstream throttledRoute
.
Error Handling Strategies
Efficient error handling is critical in a 100-Camel workflow to ensure fault tolerance and graceful recovery from failures. Apache Camel provides various error handling strategies, such as redelivery and exception clauses, to handle errors effectively.
onException(Exception.class)
.maximumRedeliveries(3)
.redeliveryDelay(500)
.handled(true)
.to("log:error");
from("direct:start")
.doTry()
.to("mock:validate")
.doCatch(Exception.class)
.to("mock:errorHandler");
In the above example, an error handling strategy is defined to handle exceptions and redeliver messages with a delay, ensuring robust error recovery.
Lessons Learned
Optimizing Apache Camel Quarkus for a 100-Camel workflow involves a combination of startup time optimization, memory consumption reduction, and performance tuning techniques. By leveraging static configuration, lazy initialization, parallel processing, throttling, and effective error handling, developers can ensure the efficient execution of a large number of Camel routes within their applications.
In conclusion, the performance and scalability of a 100-Camel workflow can be significantly enhanced through thoughtful optimization and adherence to best practices.
For further understanding, consider exploring the official Apache Camel documentation and the Quarkus optimization guide.