Seamlessly integrating JNDI and JPA in Java applications
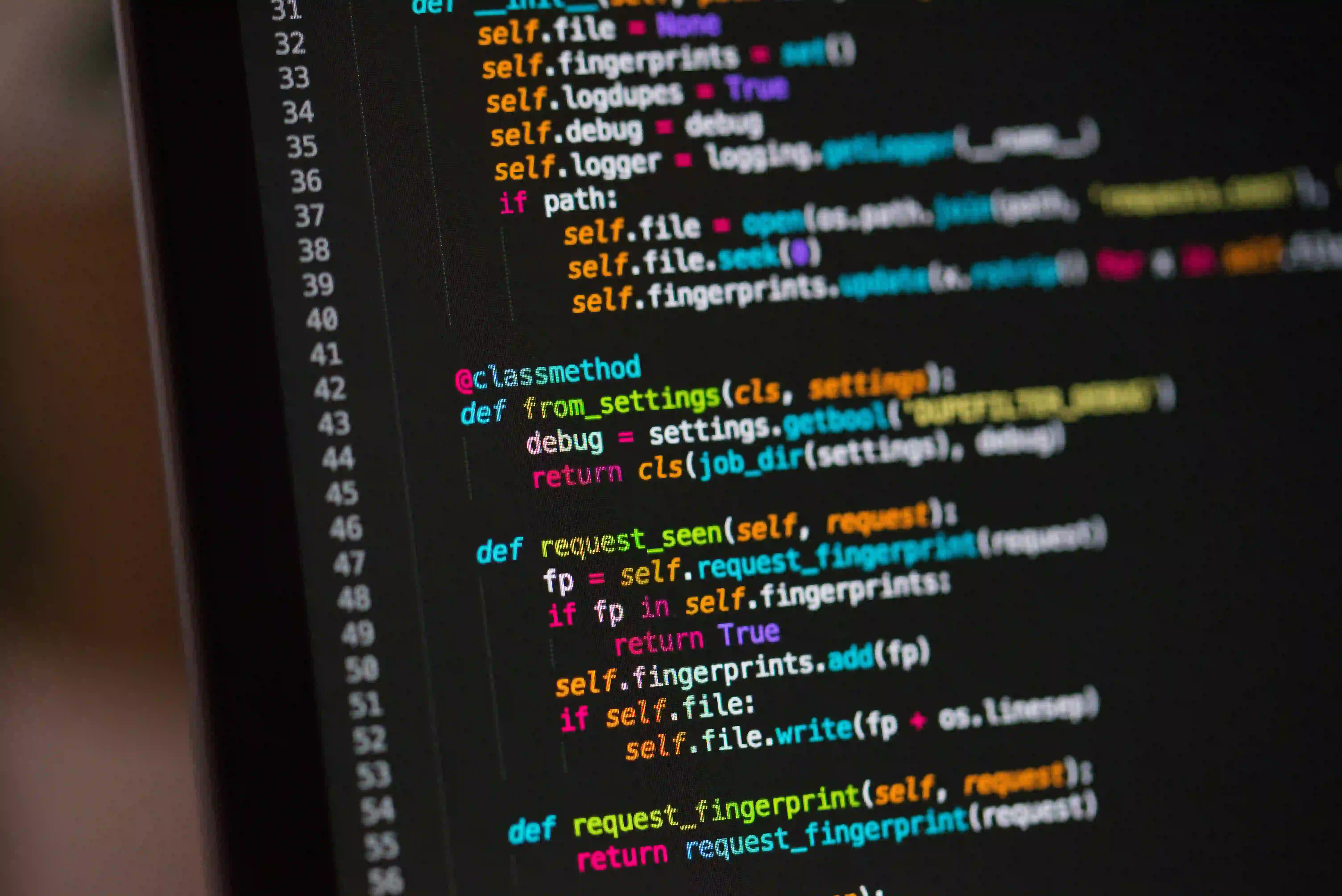
Seamlessly integrating JNDI and JPA in Java applications
In Java enterprise applications, it's common to use Java Naming and Directory Interface (JNDI) for accessing and managing resources like databases, message queues, and other services. On the other hand, Java Persistence API (JPA) is used for managing relational data in applications. In this blog post, we will explore how to seamlessly integrate JNDI and JPA in Java applications to efficiently manage database connections and perform data operations.
Setting up JNDI for database connection
JNDI allows us to decouple the resource lookup from the actual implementation, providing flexibility and ease of configuration. Let's start by setting up a JNDI resource for the database connection. First, we need to define the JNDI resource in the application server or servlet container. For example, in Tomcat, we can define a JNDI resource in the context.xml
file:
<Context>
<Resource name="jdbc/myDB" auth="Container" type="javax.sql.DataSource"
maxTotal="100" maxIdle="30" maxWaitMillis="10000"
username="db_username" password="db_password"
driverClassName="com.mysql.cj.jdbc.Driver"
url="jdbc:mysql://localhost:3306/myDB"/>
</Context>
In this example, we define a JNDI resource called jdbc/myDB
of type javax.sql.DataSource
for the MySQL database. The resource includes essential configuration properties such as maxTotal
, maxIdle
, username
, password
, driverClassName
, and url
.
Once the JNDI resource is defined, we can look it up in our Java application to obtain a database connection.
Accessing JNDI resource in Java application
In a Java application, we can use JNDI to access the database connection defined in the JNDI resource. Here's an example of how we can obtain a database connection using JNDI in a servlet:
Context initContext = new InitialContext();
Context envContext = (Context) initContext.lookup("java:comp/env");
DataSource dataSource = (DataSource) envContext.lookup("jdbc/myDB");
Connection connection = dataSource.getConnection();
// Use the connection for database operations
In this code snippet, we use the InitialContext
to obtain the initial context and then perform a lookup for the environment context. Finally, we lookup the jdbc/myDB
JNDI resource to obtain a javax.sql.DataSource
which provides us with a database connection.
Integrating JPA with JNDI
Now that we have successfully obtained a database connection using JNDI, let's integrate Java Persistence API (JPA) to manage relational data in our Java application.
First, we need to configure the JPA persistence unit to use the JNDI-bound DataSource
for database operations. We can do this by specifying the JNDI name of the DataSource
in the persistence.xml
file:
<persistence-unit name="myPersistenceUnit" transaction-type="JTA">
<jta-data-source>java:comp/env/jdbc/myDB</jta-data-source>
<!-- Other JPA configuration -->
</persistence-unit>
In this configuration, we specify the JNDI name of the DataSource
within the jta-data-source
element. This tells the JPA provider to use the JNDI-bound DataSource
for managing database connections.
Using JPA for database operations
With the JPA persistence unit configured to use the JNDI-bound DataSource
, we can now use JPA for performing database operations in our Java application. Here's an example of how we can use JPA to retrieve entities from the database:
EntityManagerFactory entityManagerFactory = Persistence.createEntityManagerFactory("myPersistenceUnit");
EntityManager entityManager = entityManagerFactory.createEntityManager();
List<MyEntity> entities = entityManager.createQuery("SELECT e FROM MyEntity e", MyEntity.class).getResultList();
// Use the retrieved entities
In this code snippet, we create an EntityManagerFactory
using the persistence unit name and then create an EntityManager
to interact with the database. We use JPA's query capabilities to retrieve entities from the database and perform operations on them.
A Final Look
Integrating JNDI and JPA in Java applications allows for efficient management of database connections and relational data operations. By leveraging JNDI for obtaining database connections and JPA for managing relational data, developers can achieve a seamless integration that enhances the scalability and maintainability of their applications.
In this blog post, we've explored the process of setting up JNDI for database connections, accessing JNDI resources in Java applications, integrating JPA with JNDI, and using JPA for database operations. This integration not only simplifies the configuration and management of database resources but also provides a standardized approach to working with relational data in Java applications.
To delve deeper into JNDI and JPA, check out the official Oracle documentation and Hibernate's JPA documentation.
Start seamlessly integrating JNDI and JPA in your Java applications to streamline database operations and enhance the overall performance of your enterprise applications. Happy coding!