Understanding Custom Object Creation in JSON Binding
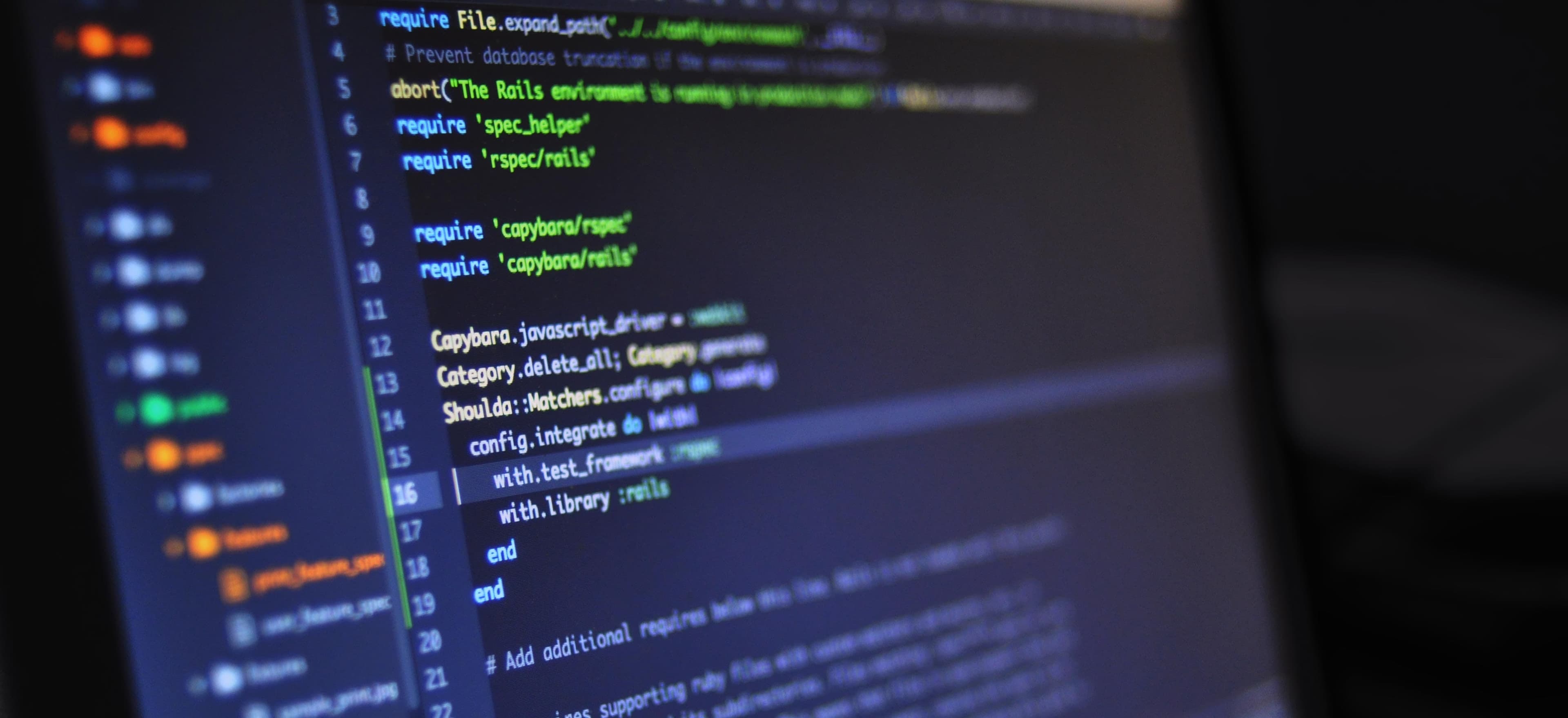
- Published on
Understanding Custom Object Creation in JSON Binding
In Java programming, handling JSON data is a common requirement. With the advent of JSON-B (JSON Binding) API in Java EE 8, developers have been provided with a convenient way to serialize Java objects into JSON and vice versa. While JSON-B provides default mapping strategies for Java objects, there are scenarios where custom object creation becomes necessary for more complex use cases.
This article delves into the concept of custom object creation in JSON binding, exploring how developers can define their custom strategies for mapping Java objects to JSON and JSON to Java.
Overview of JSON Binding API
The JSON Binding API, commonly referred to as JSON-B, is a part of the Java EE platform and provides a standardized mechanism for converting Java objects to and from JSON data. This API offers annotations and a runtime to handle the serialization and deserialization of Java objects to and from JSON format.
Default Mapping in JSON-B
By default, JSON-B uses a set of rules to map Java objects to JSON and vice versa. For example, basic data types like String
, int
, boolean
, etc., are mapped straightforwardly to their JSON equivalents. Additionally, collections and arrays in Java are mapped to JSON arrays.
For instance, consider the following Java class:
public class Person {
public String name;
public int age;
}
When serialized to JSON using JSON-B, an instance of the Person
class would be represented as:
{
"name": "John Doe",
"age": 25
}
Custom Object Creation
In some cases, the default mapping strategy of JSON-B might not be sufficient for complex Java objects. This is where custom object creation comes into play. Developers can define their own strategies for the serialization and deserialization of objects.
Customizing Serialization
To customize object creation during serialization, developers can use the JsonbSerializer
interface. This interface allows us to define our own logic for converting Java objects to JSON format.
Let's take an example where we want to customize the serialization of a Date
field in a Java class.
import javax.json.bind.serializer.JsonbSerializer;
import javax.json.bind.serializer.SerializationContext;
import javax.json.stream.JsonGenerator;
import java.lang.reflect.Type;
import java.text.SimpleDateFormat;
import java.util.Date;
public class CustomDateSerializer implements JsonbSerializer<Date> {
private SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
@Override
public void serialize(Date date, JsonGenerator generator, SerializationContext ctx) {
String formattedDate = dateFormat.format(date);
generator.write(formattedDate);
}
}
In this example, we have created a custom serializer CustomDateSerializer
that implements the JsonbSerializer
interface. Within the serialize
method, we define our custom logic for formatting the Date
object.
Customizing Deserialization
Similarly, to customize object creation during deserialization, developers can use the JsonbDeserializer
interface. This interface allows us to define our own logic for converting JSON data to Java objects.
Continuing from the previous example, let's define a custom deserializer for the Date
field.
import javax.json.bind.serializer.JsonbDeserializer;
import javax.json.bind.serializer.DeserializationContext;
import javax.json.stream.JsonParser;
import java.lang.reflect.Type;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class CustomDateDeserializer implements JsonbDeserializer<Date> {
private SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
@Override
public Date deserialize(JsonParser parser, DeserializationContext ctx, Type rtType) {
String dateValue = parser.getString();
try {
return dateFormat.parse(dateValue);
} catch (ParseException e) {
// Handle the exception
return null;
}
}
}
In this example, we have created a custom deserializer CustomDateDeserializer
that implements the JsonbDeserializer
interface. Within the deserialize
method, we define our custom logic for parsing the JSON string into a Date
object.
Registering Custom Object Creation
After defining custom serializers and deserializers, developers need to register them with the JSON-B runtime to utilize them during serialization and deserialization processes.
import javax.json.bind.Jsonb;
import javax.json.bind.JsonbBuilder;
public class PersonService {
private Jsonb jsonb = JsonbBuilder.create();
// Defining custom date serializer and deserializer
private CustomDateSerializer customDateSerializer = new CustomDateSerializer();
private CustomDateDeserializer customDateDeserializer = new CustomDateDeserializer();
public String serializePerson(Person person) {
// Registering the custom serializer and deserializer
jsonb.config(SerializationFeatures.INSERT_DATE_IN_MILLISECONDS, false)
.withAdapters(customDateSerializer, customDateDeserializer);
return jsonb.toJson(person);
}
public Person deserializePerson(String json) {
// Registering the custom serializer and deserializer
jsonb.config(SerializationFeatures.INSERT_DATE_IN_MILLISECONDS, false)
.withAdapters(customDateSerializer, customDateDeserializer);
return jsonb.fromJson(json, Person.class);
}
}
In this snippet, we create a PersonService
class responsible for serializing and deserializing Person
objects. Here, we obtain the Jsonb
instance using JsonbBuilder.create()
. We then register the custom serializer and deserializer for the Date
field using the withAdapters
method to include custom object creation during serialization and deserialization.
By following these steps, developers can leverage custom object creation in JSON-B to tailor the serialization and deserialization processes according to their specific requirements.
The Closing Argument
Custom object creation in JSON binding provides developers with the flexibility to define their strategies for mapping Java objects to JSON and JSON to Java, catering to diverse and complex use cases. By implementing custom serializers and deserializers and registering them with the JSON-B runtime, developers can tailor the serialization and deserialization processes to suit their specific needs.
For further understanding, refer to the Java EE documentation on JSON-B and explore the various features and functionalities offered by the API.
In conclusion, understanding and harnessing custom object creation capabilities in JSON-B empowers developers to efficiently handle complex data structures and ensure seamless interoperability between Java objects and JSON data.