Creating Custom Buttons in GWT using UIBinder
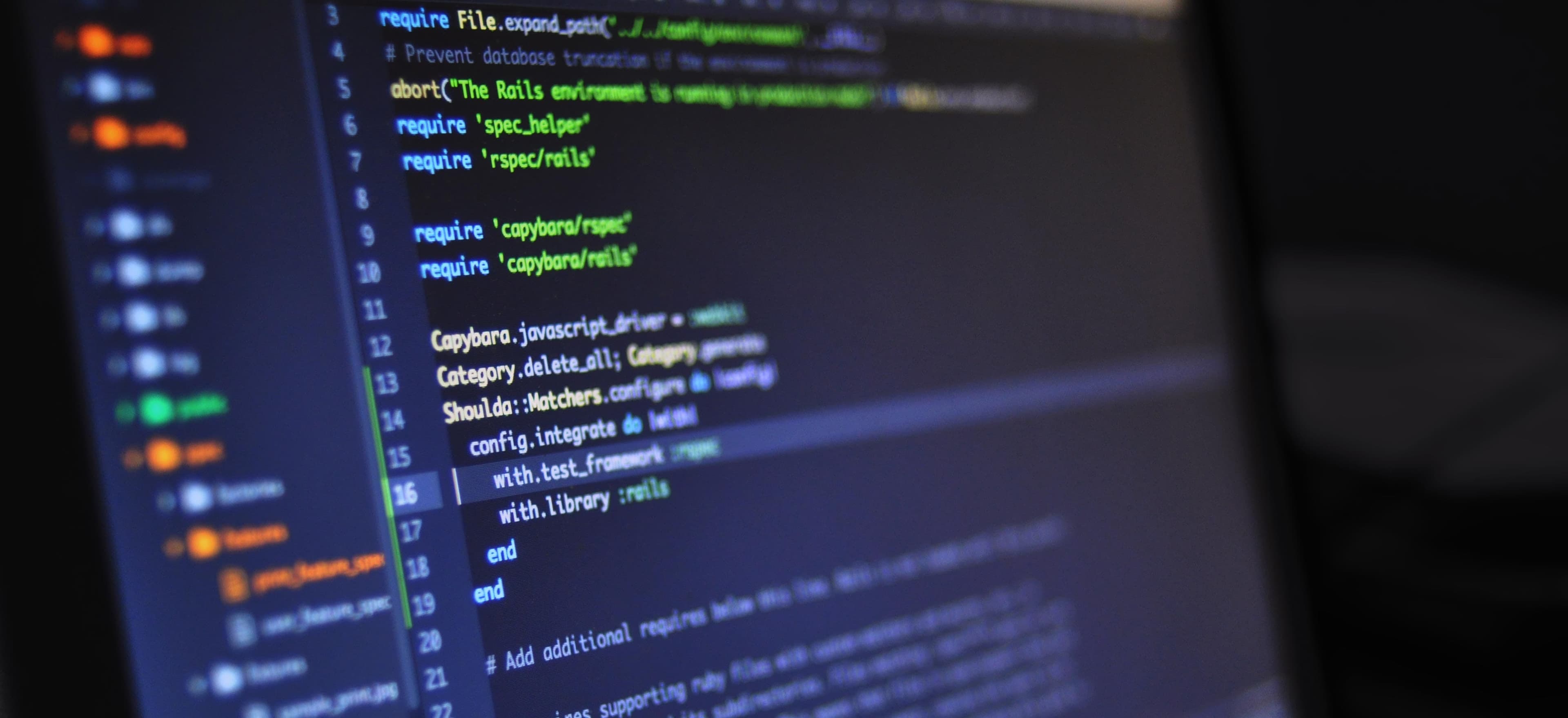
- Published on
Creating Custom Buttons in GWT using UIBinder
In this post, we'll explore how to create custom buttons in GWT (Google Web Toolkit) using UIBinder. GWT is a powerful web toolkit for building high-performance, complex web applications. UIBinder is a declarative XML-based language that allows you to define the layout and structure of your GWT components.
Why Create Custom Buttons?
While GWT provides a set of pre-defined button widgets, there are times when you may need a custom button with a specific design or functionality that is not available out-of-the-box. Creating custom buttons allows you to tailor the look and behavior of the buttons to meet your application's specific requirements.
Getting Started
Before we dive into creating custom buttons, make sure you have GWT set up in your development environment. If you haven't done so already, you can follow the instructions on the official GWT website to get started.
Creating the Custom Button Component
Let's start by creating a custom button component using UIBinder. We'll define the button's layout and appearance in a UIBinder XML file and then bind it to a Java class that handles its behavior.
Step 1: Define the UIBinder XML File
Create a new UIBinder XML file for the custom button component. Here's an example of how you can define the XML layout for the custom button:
<!-- CustomButton.ui.xml -->
<ui:UiBinder xmlns:ui='urn:ui:com.google.gwt.uibinder'
xmlns:g='urn:import:com.google.gwt.user.client.ui'>
<g:Button ui:field='customButton' addStyleNames='{style.customButton}' text='Custom Button'/>
</ui:UiBinder>
In this XML file, we define a GWT Button
widget with a custom style and text. We also assign it a field name using ui:field
for referencing it in the associated Java class.
Step 2: Create the Java Component
Next, create a Java class that represents the custom button component and binds it to the UIBinder XML file. Here's an example of how the Java class can look like:
// CustomButton.java
public class CustomButton extends Composite {
interface CustomButtonUiBinder extends UiBinder<Widget, CustomButton> {}
private static CustomButtonUiBinder uiBinder = GWT.create(CustomButtonUiBinder.class);
@UiField
Button customButton;
public CustomButton() {
initWidget(uiBinder.createAndBindUi(this));
}
// Add methods to handle button behavior
public void addClickHandler(ClickHandler handler) {
customButton.addClickHandler(handler);
}
}
In this Java class, we use the UiBinder
interface to link the Java class with the UIBinder XML file. We also define the Button
field and any methods for handling the button's behavior, such as adding click handlers.
Step 3: Using the Custom Button
Once the custom button component is created, you can use it in your GWT application by instantiating it and adding it to your UI. Here's an example of how you can use the custom button in your GWT application:
// Example usage
CustomButton customButton = new CustomButton();
customButton.addClickHandler(event -> Window.alert("Custom button clicked"));
RootPanel.get().add(customButton);
Styling the Custom Button
To enhance the visual appeal of the custom button, you can apply custom styles to it using CSS. You can define the styles in a separate CSS file and apply them to the custom button using the addStyleNames
method in the UIBinder XML file.
Step 1: Define Custom Styles
Create a CSS file and define custom styles for the button. For example:
/* custom-button.css */
.customButton {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
border-radius: 5px;
}
Step 2: Apply Custom Styles
In the UIBinder XML file, apply the custom styles to the button using the addStyleNames
attribute:
<!-- CustomButton.ui.xml -->
<ui:UiBinder xmlns:ui='urn:ui:com.google.gwt.uibinder'
xmlns:g='urn:import:com.google.gwt.user.client.ui'
xmlns:res='urn:with:com.example.resources'>
<ui:style src='custom-button.css'/>
<g:Button ui:field='customButton' addStyleNames='{res.myResources.customButton}' text='Custom Button'/>
</ui:UiBinder>
By referencing the custom style in the addStyleNames
attribute, the custom button will inherit the specified styles.
Closing Remarks
In this post, we've explored the process of creating custom buttons in GWT using UIBinder. By leveraging UIBinder's declarative approach, you can easily define the layout and styling of custom buttons, which can then be bound to Java classes to handle their behavior. Custom buttons provide flexibility and customization options that go beyond the standard button widgets provided by GWT, allowing you to create tailored user interface components for your GWT applications. By following these steps, you can create custom buttons that seamlessly integrate with your GWT projects and enhance the user experience.