Fine-Grained Sorting in JDK 8
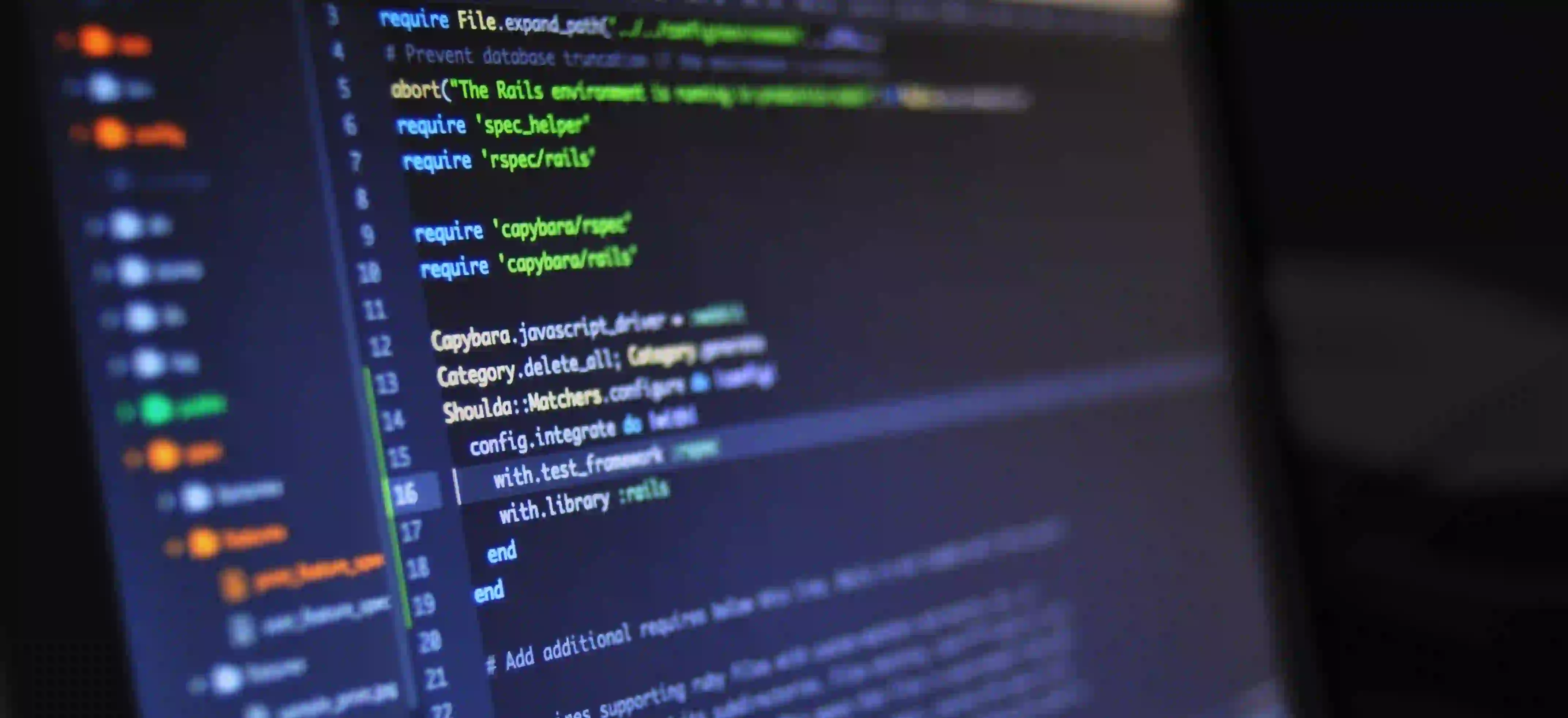
Unleashing the Power of Fine-Grained Sorting in JDK 8
In the world of programming, sorting is an essential operation that helps in organizing data efficiently. With the release of JDK 8, Java introduced a powerful feature known as fine-grained sorting, which has revolutionized the way developers sort data structures. In this article, we will delve into the concept of fine-grained sorting, understand its benefits, and explore practical examples of its implementation.
Understanding Fine-Grained Sorting
Fine-grained sorting in JDK 8 offers a more granular control over the sorting process, allowing developers to specify custom comparators for different elements within a collection. This means that instead of applying a single sorting criterion to the entire collection, you can now define multiple sorting criteria based on specific attributes of the elements.
Benefits of Fine-Grained Sorting
The introduction of fine-grained sorting brings about several benefits:
- Enhanced Flexibility: Fine-grained sorting provides developers with the flexibility to define unique sorting criteria for different attributes of the elements in a collection.
- Improved Readability: By specifying custom comparators for specific attributes, the code becomes more readable and intuitive, making it easier for other developers to understand the sorting logic.
- Precise Sorting Control: Developers can exercise precise control over the sorting process, ensuring that elements are ordered exactly as per the defined criteria.
Now, let's explore how fine-grained sorting can be leveraged in Java applications through practical examples.
Practical Implementation
Scenario 1: Sorting a Collection of Custom Objects
Consider a scenario where you have a collection of Person
objects, and you want to sort them based on their age and then by their name in case of a tie. Prior to JDK 8, achieving this level of fine-grained sorting would have been a daunting task. Let's look at how this can be easily accomplished using fine-grained sorting in JDK 8.
List<Person> people = // populate the list
people.sort(Comparator.comparing(Person::getAge).thenComparing(Person::getName));
In this example, we are sorting the list of Person
objects first by their age using Comparator.comparing
, and then by their name using thenComparing
. This concise and expressive code demonstrates the power of fine-grained sorting in JDK 8.
Scenario 2: Sorting Using Custom Rules
Suppose you have a list of employees, and you need to sort them based on a custom set of rules specific to your business domain. With fine-grained sorting, you can easily define custom comparators to cater to these specific requirements without compromising the readability of your code.
List<Employee> employees = // populate the list
employees.sort((emp1, emp2) -> {
if (emp1.getDepartment().equals(emp2.getDepartment())) {
return Integer.compare(emp1.getYearsOfService(), emp2.getYearsOfService());
} else {
return emp1.getDepartment().compareTo(emp2.getDepartment());
}
});
In this example, we are sorting the list of Employee
objects based on a custom set of rules. If the employees belong to the same department, we sort them by their years of service. Otherwise, we sort them based on their department names.
The Bottom Line
Fine-grained sorting in JDK 8 empowers Java developers with the ability to finely control the sorting process, resulting in more expressive, readable, and precise sorting operations. By leveraging custom comparators, developers can craft intricate sorting logic tailored to their specific requirements, thereby enhancing the overall quality of their code.
As you continue to explore the capabilities of JDK 8, consider integrating fine-grained sorting into your projects to take advantage of its robust and intuitive features.
To dive deeper into sorting in Java, you can explore the official Java documentation on Comparator Interface and Collections Class.
Happy coding!