How to Ensure Interface Invariant Testing?
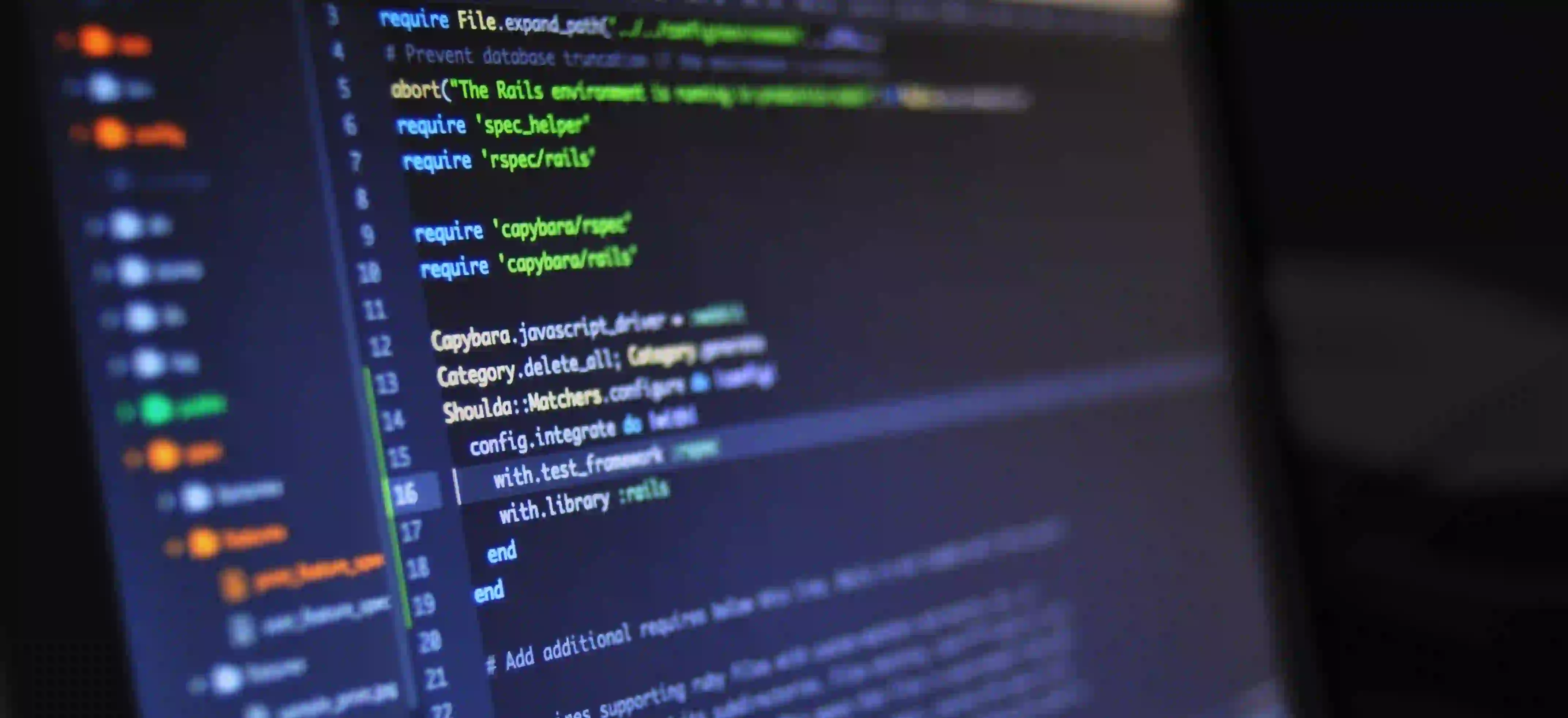
Ensuring Interface Invariant Testing in Java
When developing software in Java, it's crucial to ensure that the interfaces within the codebase are thoroughly tested. Interface invariants are the conditions that must be maintained upon entry and exit of every public method of a class that implements an interface. Testing these interface invariants helps to guarantee the correctness and stability of the code. In this article, we'll explore the concept of interface invariants and how to effectively test them in Java.
What are Interface Invariants?
In the context of Java, an interface defines a contract for a class, specifying a set of methods that the class must implement. Interface invariants, therefore, refer to the conditions that these methods must satisfy. When a class implements an interface, it commits to upholding the invariants defined by the interface. This ensures that the class behaves as expected and can be used interchangeably with other classes that implement the same interface.
For example, consider an interface List
with a method add
that appends an element to the list. One of the interface invariants could be that after calling add
, the size of the list should increase by 1. Testing this invariant ensures that any class implementing the List
interface maintains this behavior consistently.
Writing Test Cases for Interface Invariants
To ensure that interface invariants are upheld, it's essential to write comprehensive test cases. Test cases should cover a variety of scenarios to validate the behavior of the class implementing the interface under different conditions. When writing test cases for interface invariants, it's important to consider both the typical use cases and any edge cases that might arise.
Let's consider a simple example using JUnit to illustrate how test cases for interface invariants can be written in Java.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class ListTest {
@Test
public void testAddIncreasesSizeByOne() {
List<String> list = new ArrayList<>();
int initialSize = list.size();
list.add("element");
assertEquals(initialSize + 1, list.size());
}
}
In this example, the testAddIncreasesSizeByOne
method validates the interface invariant that adding an element to the list increases its size by one. It sets up the initial state, performs the operation, and then asserts that the invariant holds true. Writing test cases in this manner ensures that the interface invariants are rigorously tested.
It's important to note that testing interface invariants not only helps to verify the correctness of the implementation but also serves as documentation for the expected behavior of the class implementing the interface.
Using Mock Objects for Interface Invariant Testing
In some cases, testing interface invariants might require the use of mock objects to isolate the behavior of the class implementing the interface. Mock objects can be particularly useful when testing interactions with external dependencies or when certain conditions are hard to replicate in a real environment.
import static org.mockito.Mockito.*;
import org.junit.Test;
public class OrderProcessorTest {
@Test
public void testOrderProcessing() {
// Create a mock payment processor
PaymentProcessor mockPaymentProcessor = mock(PaymentProcessor.class);
// Create the order processor with the mock payment processor
OrderProcessor orderProcessor = new OrderProcessor(mockPaymentProcessor);
// Set up test conditions
Order order = new Order(/*...*/);
// Perform the operation
orderProcessor.processOrder(order);
// Verify that the invariant is upheld
verify(mockPaymentProcessor, times(1)).processPayment(order.getTotal());
}
}
In this example, we use Mockito to create a mock PaymentProcessor
. We set up the test conditions, perform the operation, and then verify that the processPayment
method of the PaymentProcessor
is called exactly once with the total amount of the order. This allows us to test the interface invariants related to payment processing without relying on a real payment processor.
Wrapping Up
Ensuring interface invariant testing is an integral part of Java software development. By thoroughly testing the behavior of classes that implement interfaces, we can guarantee that the defined invariants are consistently maintained. Writing comprehensive test cases, using mock objects when necessary, and leveraging testing frameworks such as JUnit and Mockito are essential practices for effective interface invariant testing in Java.
By upholding interface invariants through rigorous testing, developers can be confident in the reliability and consistency of their code, ultimately leading to higher quality software products.
In summary, interface invariant testing is a critical aspect of Java development, and by following best practices and leveraging the right tools, developers can ensure the correctness and stability of their code.