Addressing Compatibility Issues with Java 17 and Spring Framework 6
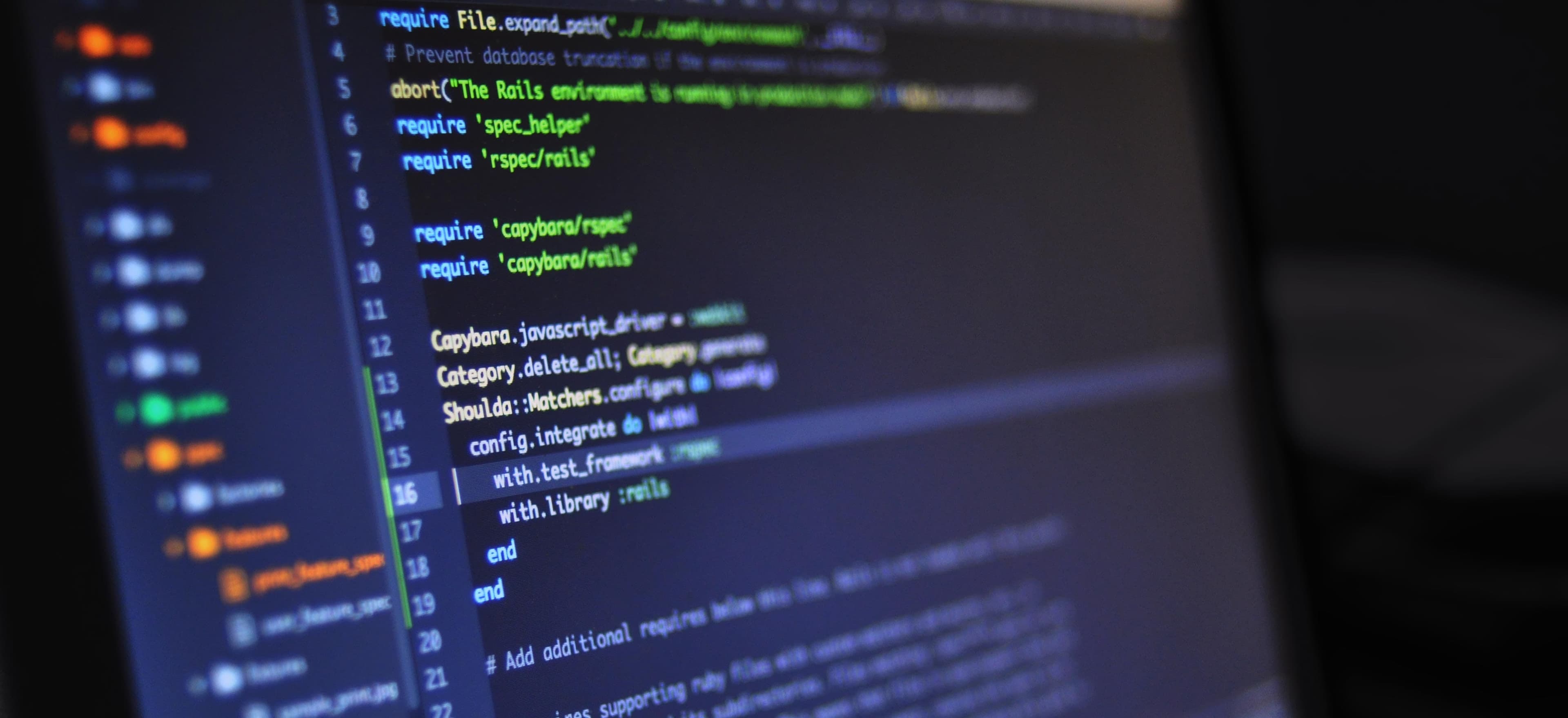
- Published on
Understanding Compatibility Issues with Java 17 and Spring Framework 6
When it comes to Java development, staying ahead with the latest language features and improvements is crucial. With the release of Java 17, developers gain access to new features, performance improvements, and enhanced security. However, adopting the latest Java version may introduce compatibility issues, particularly with popular frameworks like Spring.
In this article, we will explore the compatibility concerns between Java 17 and Spring Framework 6. We will also discuss potential solutions and best practices for addressing these issues to ensure a smooth transition to Java 17 while using Spring Framework 6.
What's New in Java 17?
Java 17, released in September 2021, is the latest long-term support (LTS) version of Java. It comes with a plethora of new features and enhancements, including sealed classes, pattern matching for switch expressions, and enhanced pseudo-random number generators, among others. These features aim to improve developer productivity, code readability, and performance.
Introducing Spring Framework 6
Spring Framework is one of the most widely used Java frameworks for building enterprise applications. It provides comprehensive infrastructure support, making it easier to develop robust and maintainable Java applications. With the release of Spring Framework 6, developers have access to new features and enhancements designed to align with the latest Java versions and industry best practices.
Compatibility Challenges with Java 17 and Spring Framework 6
While the advancements in Java 17 and Spring Framework 6 are beneficial, they also pose compatibility challenges. Upgrading to Java 17 may lead to incompatibilities with Spring Framework 6, potentially causing runtime errors, deprecated APIs, or behavioral inconsistencies.
Some of the key compatibility challenges include:
- Deprecation of APIs: Java 17 introduces deprecations and removals of certain APIs and features, which may be actively used within Spring Framework 6.
- Language Changes: New language features and enhancements in Java 17 may impact the way Spring Framework 6 code is interpreted and executed.
- Library Dependencies: Existing dependencies and libraries utilized by Spring Framework 6 may not be fully compatible with Java 17, leading to conflicts and runtime issues.
Solutions and Best Practices
To address compatibility issues between Java 17 and Spring Framework 6, consider the following solutions and best practices:
Upgrade to Spring Framework 6.1
Spring Framework 6.1 is specifically designed to align with the latest advancements in Java, including Java 17 compatibility. Upgrading to Spring Framework 6.1 ensures that your application leverages the new features of Java 17 seamlessly.
Review API Deprecations
Carefully review the deprecated APIs and features introduced in Java 17. Make necessary changes within your Spring Framework 6 codebase to replace deprecated APIs with their recommended alternatives. This ensures that your code remains compatible with the latest Java version.
Dependency Updates
Ensure that all dependencies and libraries used by Spring Framework 6 are updated to versions that are fully compatible with Java 17. This includes third-party libraries, plugins, and any internal dependencies within your application.
Quality Assurance and Testing
Conduct thorough testing of your Spring Framework 6 application on Java 17 to identify any compatibility issues, runtime errors, or behavioral inconsistencies. Automated testing suites and test-driven development practices can aid in identifying and addressing potential compatibility issues early in the development cycle.
Adopting Modularization
Consider modularization techniques to isolate and encapsulate code that may be affected by Java 17 updates. Modularization allows for targeted compatibility improvements and reduces the impact of broader language changes on your Spring Framework 6 application.
Community Support and Resources
Leverage the support of the Java and Spring community, including forums, discussion groups, and official documentation. Community insights, best practices, and shared experiences can provide valuable guidance in addressing compatibility challenges.
Sample Code for Compatibility Handling
Let's take a look at a simple example of how to handle compatibility with Java 17 within a Spring Framework 6 application.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyAppConfiguration {
// Bean configuration using sealed classes from Java 17
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
In this code snippet, we are utilizing the MyService
interface and its implemented class MyServiceImpl
within a Spring configuration. With the introduction of sealed classes in Java 17, we can leverage the benefits of enhanced type-checking and code security. This demonstrates how compatibility with new Java features can be seamlessly integrated within a Spring Framework 6 application.
Closing Remarks
As Java 17 continues to gain adoption, ensuring compatibility with frameworks like Spring Framework 6 becomes imperative for smooth application modernization. By understanding the compatibility challenges, adopting best practices, and leveraging community resources, developers can navigate the transition to Java 17 while maintaining the integrity of their Spring Framework 6 applications.
To conclude, it's crucial to stay informed about the latest updates, consult official documentation, and proactively address compatibility issues for a seamless and sustainable Java 17 and Spring Framework 6 integration.
In the rapidly evolving landscape of Java development, staying proactive and adaptable is key to harnessing the full potential of new language versions while maintaining the stability and performance of established frameworks.