Implementing Two-Way Data Binding in JavaFX
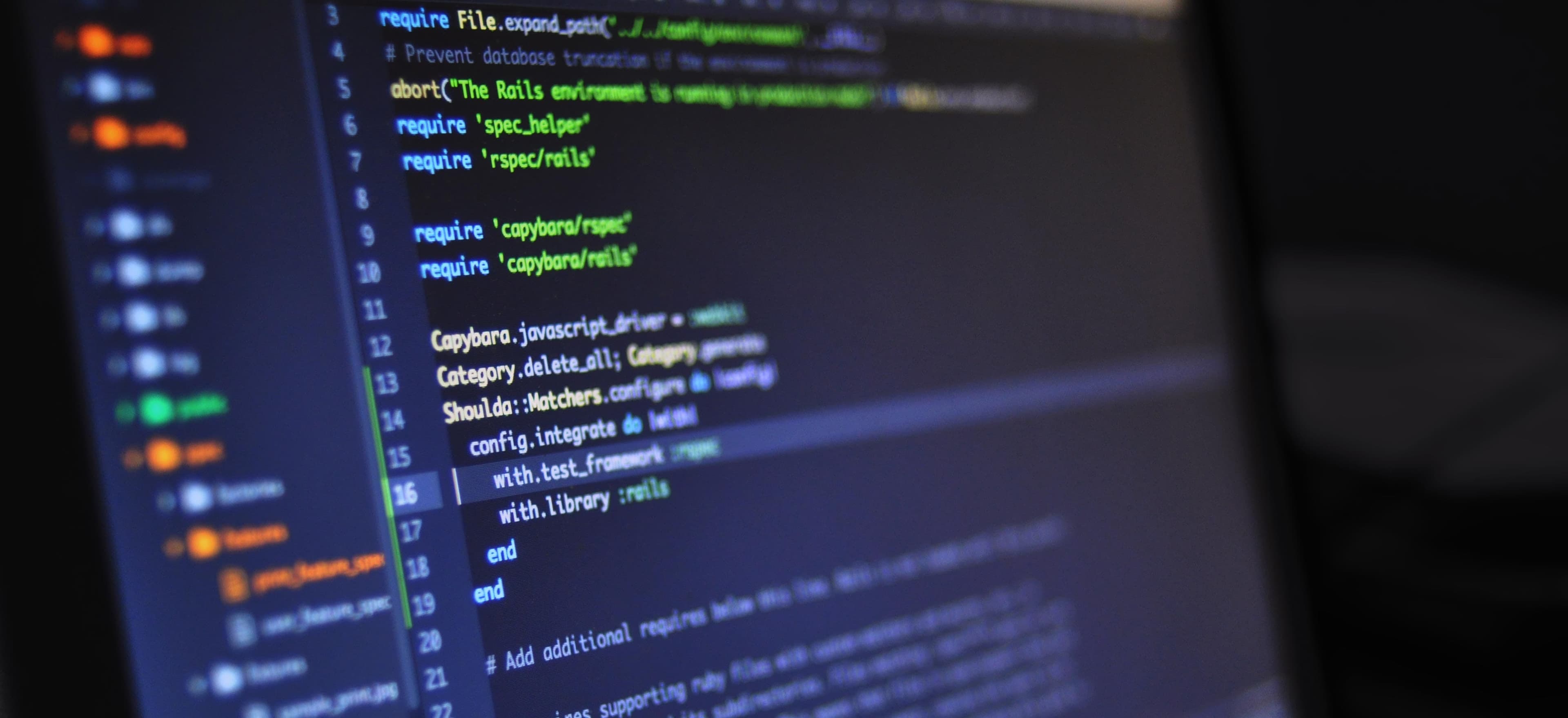
- Published on
In Java development, JavaFX is a powerful framework for building desktop and web applications with rich user interfaces. Two-way data binding is a critical feature in modern user interface frameworks where changes in the user interface elements are automatically reflected in the data model, and vice versa. In this article, we will explore how to implement two-way data binding in JavaFX, leveraging its powerful binding API.
What is Two-Way Data Binding?
Two-way data binding is a technique that establishes a synchronization relationship between the UI controls and the data model. When the data model changes, the UI controls are automatically updated, and when the user interacts with the UI controls, the data model is updated accordingly.
Setting Up the Project
Before diving into the implementation, ensure you have Java JDK installed. If not, download and install it. Additionally, you can leverage build tools like Maven or Gradle for managing dependencies and building the project. In this article, we will use Maven for managing the project.
Create a new Maven project and add the JavaFX dependency in the pom.xml
file:
<dependencies>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>16</version>
</dependency>
</dependencies>
Ensure your pom.xml
specifies the Java version to 11:
<properties>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
Implementing Two-Way Data Binding
In JavaFX, two-way data binding can be achieved using the Bindings
class and JavaFX properties. Let's illustrate this with an example of binding a TextField
to a StringProperty
.
Create a simple JavaFX application with a TextField
and a label to display the text entered in the TextField
.
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class TwoWayBindingExample extends Application {
@Override
public void start(Stage stage) {
TextField textField = new TextField();
Label label = new Label();
label.textProperty().bind(textField.textProperty());
VBox vBox = new VBox(10, textField, label);
vBox.setPadding(new Insets(10));
Scene scene = new Scene(vBox, 300, 200);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this example, the textProperty
of the TextField
is bound to the textProperty
of the Label
using the bind
method, establishing a two-way data binding. Now, when you type in the TextField
, the text will automatically update in the Label
, and vice versa, demonstrating two-way data binding in action.
Why Two-Way Data Binding in JavaFX?
Two-way data binding in JavaFX simplifies the code by automatically propagating changes between the UI controls and the data model. It eliminates the need for manual synchronization, reducing the potential for errors and making the code more maintainable.
Using JavaFX Properties
JavaFX provides various property types, such as StringProperty
, IntegerProperty
, BooleanProperty
, etc., that can be used to enable two-way binding. These properties encapsulate the value and provide mechanisms for notification of changes.
Advantages of Using JavaFX Properties for Two-Way Binding:
- Change Notification: The JavaFX properties provide built-in change notification mechanisms, notifying the binding when the value changes.
- Type Safety: The use of typed properties ensures type safety, avoiding runtime type errors.
- Integration with UI Controls: JavaFX properties seamlessly integrate with UI controls, enabling easy binding and synchronization.
Let's extend our example to use a StringProperty
to demonstrate how JavaFX properties facilitate two-way binding.
import javafx.beans.property.SimpleStringProperty;
import javafx.beans.property.StringProperty;
public class TwoWayBindingExample extends Application {
@Override
public void start(Stage stage) {
TextField textField = new TextField();
Label label = new Label();
StringProperty textProperty = new SimpleStringProperty();
textField.textProperty().bindBidirectional(textProperty);
label.textProperty().bind(textProperty);
VBox vBox = new VBox(10, textField, label);
vBox.setPadding(new Insets(10));
Scene scene = new Scene(vBox, 300, 200);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this extended example, a StringProperty
named textProperty
is created, and the bidirectional binding is established between the TextField
and the StringProperty
. Subsequently, the Label
is bound to the same textProperty
, enabling bidirectional synchronization between the TextField
and the Label
.
Key Takeaways
In conclusion, two-way data binding in JavaFX simplifies the development of user interfaces by automating the synchronization between UI controls and the data model. Leveraging JavaFX properties and the powerful binding API, developers can create responsive and maintainable applications with less boilerplate code. By implementing two-way data binding, JavaFX empowers developers to build intuitive and dynamic user interfaces seamlessly integrated with the underlying data model.
Implementing two-way data binding in JavaFX not only enhances the user experience but also streamlines the development process, making it a valuable technique for modern Java application development.
In this article, we have explored the fundamentals of two-way data binding in JavaFX, showcasing its implementation and the benefits it offers. By incorporating two-way data binding into your JavaFX applications, you can create more responsive, interactive, and maintainable user interfaces, ultimately enhancing the overall user experience.
Start incorporating two-way data binding in your JavaFX projects to elevate your application's user interface to the next level of responsiveness and user interaction!
Happy coding!