Utilizing Java 8 Stream FlatMap for Effective Data Transformation
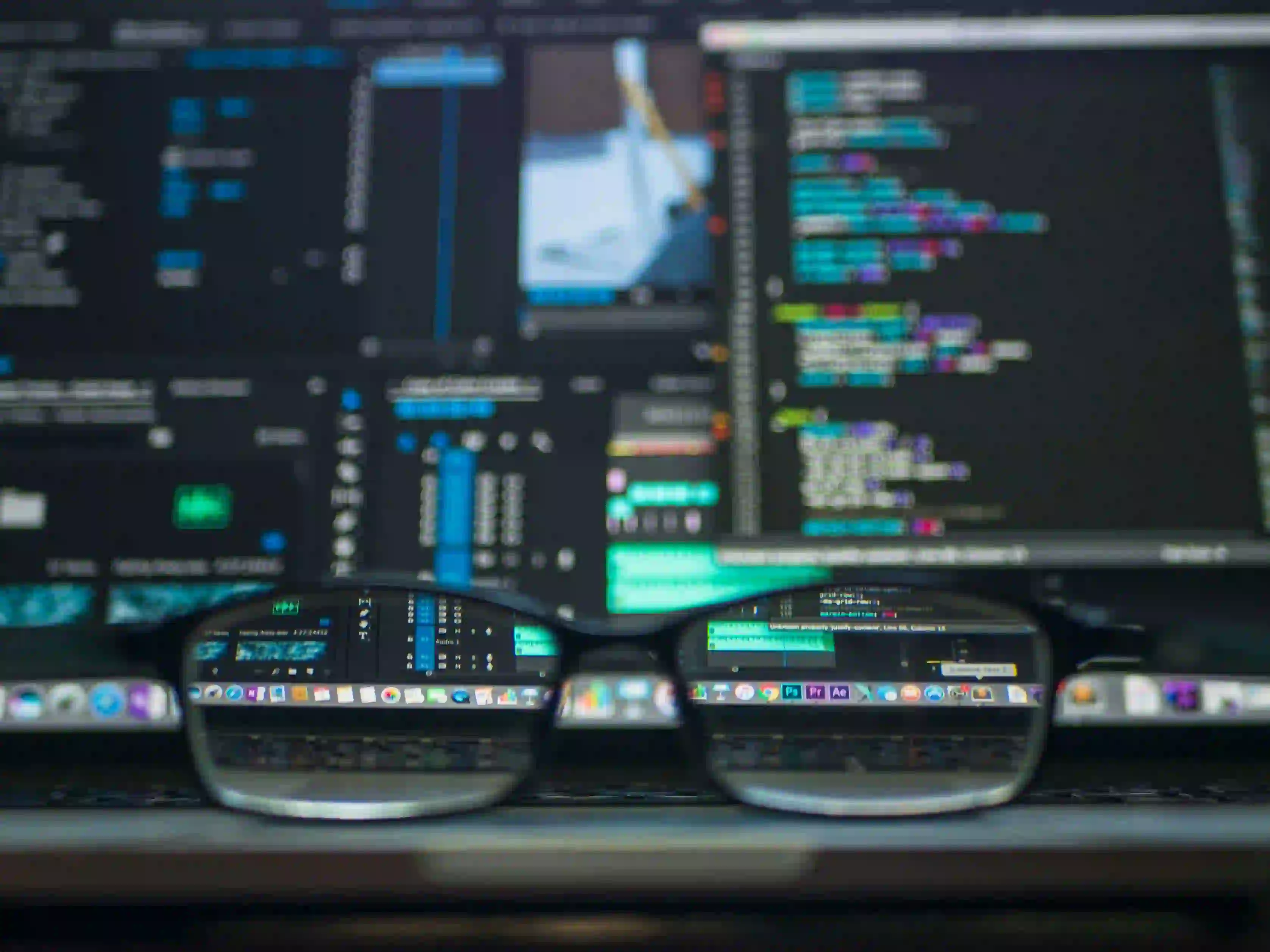
Leveraging Java 8 Stream flatMap
for Enhanced Data Transformation
In the realm of Java programming, the introduction of Streams in Java 8 revolutionized the way we handle collections. Streams offer a fluent and expressive way to perform operations on sequences of elements. Among the myriad of functions available in Streams, flatMap
stands out as a powerful tool for transforming and manipulating data structures. In this post, we will delve into the intricacies of flatMap
and explore its applications for effective data transformation.
Understanding flatMap
At its core, the flatMap
function is an intermediate operation in the Stream API that performs a one-to-many transformation on the elements of the stream. It takes a function as an argument, which itself returns a Stream, and then flattens the resulting streams into a single stream. This allows for a seamless transformation of complex data structures, such as nested collections or maps, into a single stream of elements.
Practical Examples
Let's dive into some practical examples to illustrate the versatility and utility of flatMap
.
Example 1: Flattening Nested Collections
Consider a scenario where we have a list of lists, and we want to flatten it into a single list. Prior to Java 8, achieving this operation would require cumbersome nested loops. However, with flatMap
, this task becomes succinct and comprehensible.
List<List<Integer>> nestedList = Arrays.asList(
Arrays.asList(1, 2),
Arrays.asList(3, 4),
Arrays.asList(5, 6)
);
List<Integer> flattenedList = nestedList.stream()
.flatMap(List::stream)
.collect(Collectors.toList());
System.out.println(flattenedList); // Output: [1, 2, 3, 4, 5, 6]
In this example, the flatMap
operation seamlessly transforms the nested structure of List<List<Integer>>
into a single stream, which is then collected into a flattened list.
Why This Works
The flatMap
operation, in this case, applies the List::stream
function to each element of the original stream. This produces individual streams for each nested list, which are then flattened into a single stream, preserving the order of elements.
Example 2: Parsing and Flattening JSON Data
Another compelling use case for flatMap
is the parsing and flattening of JSON data. When working with JSON arrays containing nested objects or arrays, flatMap
facilitates the extraction of specific elements by flattening the nested structure.
JSONArray jsonArray = new JSONArray("[{'name': 'Alice', 'languages': ['Java', 'Python']}, {'name': 'Bob', 'languages': ['C++', 'JavaScript']}]");
List<String> allLanguages = IntStream.range(0, jsonArray.length())
.mapToObj(jsonArray::getJSONObject)
.flatMap(jsonObject -> jsonObject.getJSONArray("languages").toList().stream())
.map(Object::toString)
.collect(Collectors.toList());
System.out.println(allLanguages); // Output: [Java, Python, C++, JavaScript]
In this example, the flatMap
operation navigates through the JSON array, extracts the nested languages
arrays, and flattens them into a single stream of languages.
Why This Works
The functional flow within the flatMap
allows for seamless extraction and flattening of the nested languages
arrays. By returning a stream of languages for each JSON object, the flatMap
operation culminates in a unified stream of all languages.
Advanced Applications
Beyond the aforementioned examples, flatMap
can be employed in more advanced scenarios, such as asynchronous processing, database querying, or even hierarchical data manipulation. Its versatility lies in its ability to gracefully handle intricate data structures and streamline the transformation process.
Example 3: Asynchronous Data Processing
Consider a situation where data processing tasks need to be executed asynchronously, and the results need to be aggregated. flatMap
can be utilized to parallelize these tasks and merge the results into a cohesive stream.
List<CompletableFuture<String>> asyncResults = fetchDataAsynchronously()
.stream()
.map(this::processDataAsync)
.collect(Collectors.toList());
List<String> mergedResults = asyncResults.stream()
.map(CompletableFuture::join)
.collect(Collectors.toList());
In this example, the flatMap
operation could be integrated to parallelize the asynchronous processing of data and then seamlessly combine the individual results into a single stream.
Why flatMap
Excels Here
By using flatMap
in an async context, each asynchronous data processing task can generate a stream of results. These streams can then be flattened into one collective stream, ensuring a unified aggregation of results.
The Bottom Line
In conclusion, the Java 8 Stream flatMap
function serves as a formidable tool for transforming and manipulating complex data structures. Its ability to streamline one-to-many transformations, flatten nested collections, and facilitate advanced processing makes it a valuable asset in the Java developer's toolkit. By harnessing the power of flatMap
, developers can enhance their code's readability, maintainability, and performance, ultimately leading to more robust and efficient applications.
With its remarkable versatility and utility, flatMap
continues to be a cornerstone of modern Java programming, enabling developers to tackle data transformation challenges with elegance and precision.
Incorporating flatMap
into your codebase can significantly enhance its expressiveness and maintainability. Embrace the power of flatMap
today and elevate your data transformation capabilities in Java.
To delve deeper into Java 8 Streams and flatMap
, I recommend exploring the official documentation on Oracle's Java Platform, Standard Edition and the insightful resources provided by Baeldung.