Optimizing Built-In Serialization for Efficient Data Storage
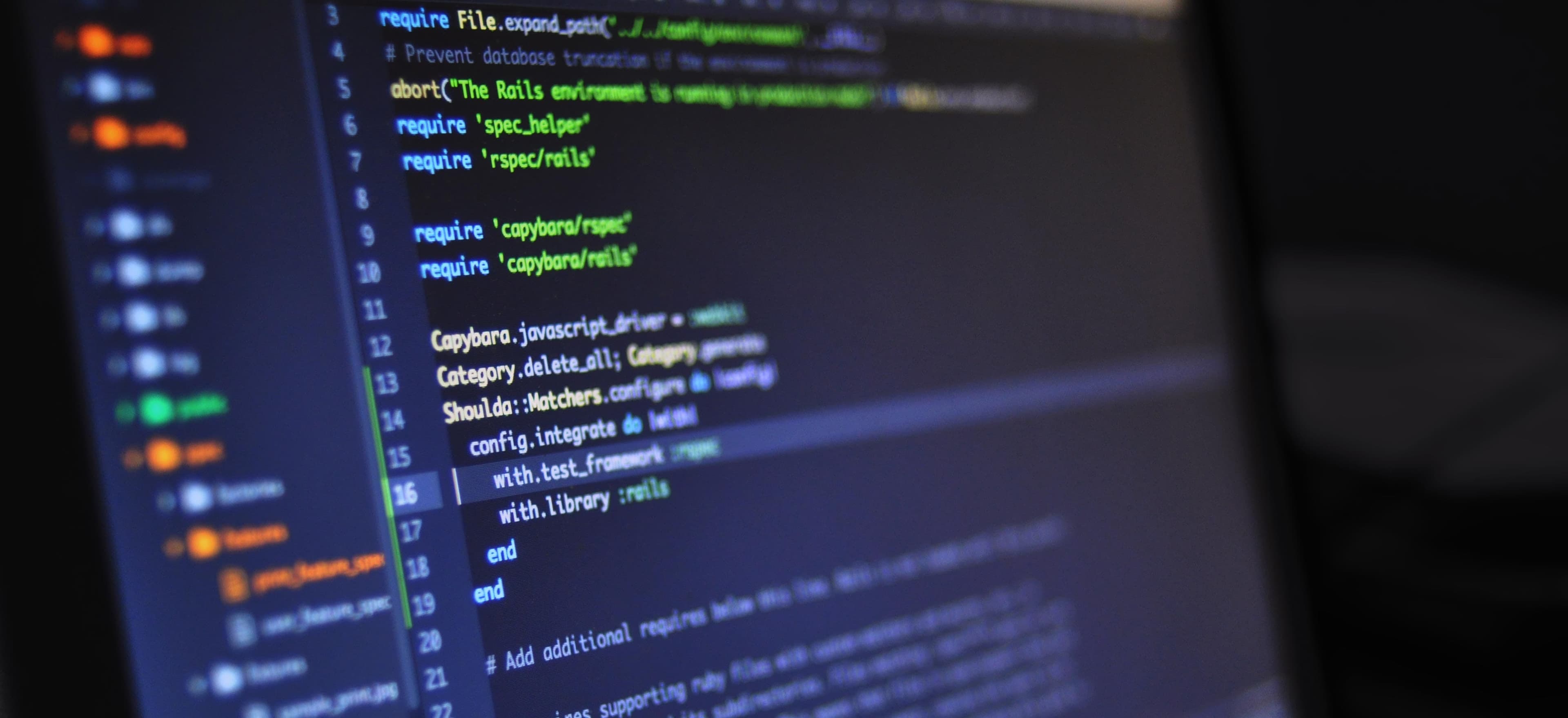
- Published on
Optimizing Built-In Serialization for Efficient Data Storage
In Java, serialization is the process of converting an object into a stream of bytes to store the object or transmit it to memory, a database, or a file. Serialization is an essential part of Java programming as it allows the state of an object to be converted into a format that can be easily stored and later reconstructed. While Java provides built-in serialization mechanisms through the java.io.Serializable
interface, it's crucial to optimize this process for efficient data storage and retrieval.
Understanding Built-In Serialization in Java
Java provides built-in support for serialization and deserialization through the java.io.Serializable
interface. By implementing this interface, a class indicates that its non-transient fields can be written to an ObjectOutputStream
for serialization. During deserialization, the non-transient fields of the class can be restored from the ObjectInputStream
.
Optimizing Serialization for Efficient Data Storage
1. Implementing Custom Serialization
While built-in serialization provides a straightforward way to serialize and deserialize objects, it may not always be the most efficient option. Implementing custom serialization using the writeObject
and readObject
methods allows for more control over the serialization process. By customizing the serialization logic, unnecessary data can be excluded, leading to smaller serialized object sizes and improved storage efficiency.
private void writeObject(ObjectOutputStream out) throws IOException {
// Custom serialization logic to write only necessary data
out.writeObject(this.field1);
out.writeObject(this.field2);
// Additional serialization logic
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
// Custom deserialization logic to read only necessary data
this.field1 = (Type1) in.readObject();
this.field2 = (Type2) in.readObject();
// Additional deserialization logic
}
2. Using Externalizable Interface
Another way to optimize serialization in Java is by implementing the java.io.Externalizable
interface. Unlike the Serializable
interface, Externalizable
gives complete control over the serialization and deserialization process. This approach can be more efficient when dealing with large or complex objects, as it allows for fine-grained control over the serialized format.
public class CustomObject implements Externalizable {
@Override
public void writeExternal(ObjectOutput out) throws IOException {
// Custom serialization logic
}
@Override
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
// Custom deserialization logic
}
}
3. Using Transient and Static Fields
In Java serialization, marking fields as transient
excludes them from the serialization process. This can be particularly useful when dealing with fields that do not need to be persisted or can be reconstructed based on other data. Additionally, marking fields as static
excludes them from serialization as well, as static fields belong to the class rather than individual objects.
public class TransientExample implements Serializable {
private int nonTransientField;
private transient String transientField;
private static int staticField;
}
4. Choosing the Right Serialization Format
Java's built-in serialization uses a binary format, which is not human-readable and can be inefficient in terms of storage. Alternatively, using JSON or XML for serialization can provide a more compact and readable representation of the data. Libraries like Jackson for JSON or JAXB for XML can be used to serialize Java objects into these formats, offering more flexibility and interoperability across different systems.
// Serialization to JSON using Jackson library
ObjectMapper objectMapper = new ObjectMapper();
String json = objectMapper.writeValueAsString(object);
5. Performance Considerations
Optimizing serialization for efficient data storage should also consider performance implications. Serialization and deserialization can be resource-intensive operations, especially for large objects or complex object graphs. It's important to measure the performance impact of serialization in terms of memory usage and CPU time, and balance it with the storage efficiency gained through optimization.
My Closing Thoughts on the Matter
Optimizing built-in serialization in Java is essential for efficient data storage and retrieval. By implementing custom serialization, using the Externalizable
interface, managing transient and static fields, choosing the right serialization format, and considering performance implications, developers can enhance the efficiency of data storage while maintaining seamless object serialization and deserialization.
With these optimization techniques, Java applications can effectively manage and store data in a compact and efficient manner, ultimately improving the overall performance and resource utilization.
For further exploration of Java serialization optimization, check out the official Oracle documentation on serialization and the Jackson library for JSON serialization.
Remember, optimizing serialization is a critical aspect of efficient data storage, and understanding these techniques can greatly benefit the performance of Java applications.
Checkout our other articles