Minimizing Security Risks When Using Third-Party Code
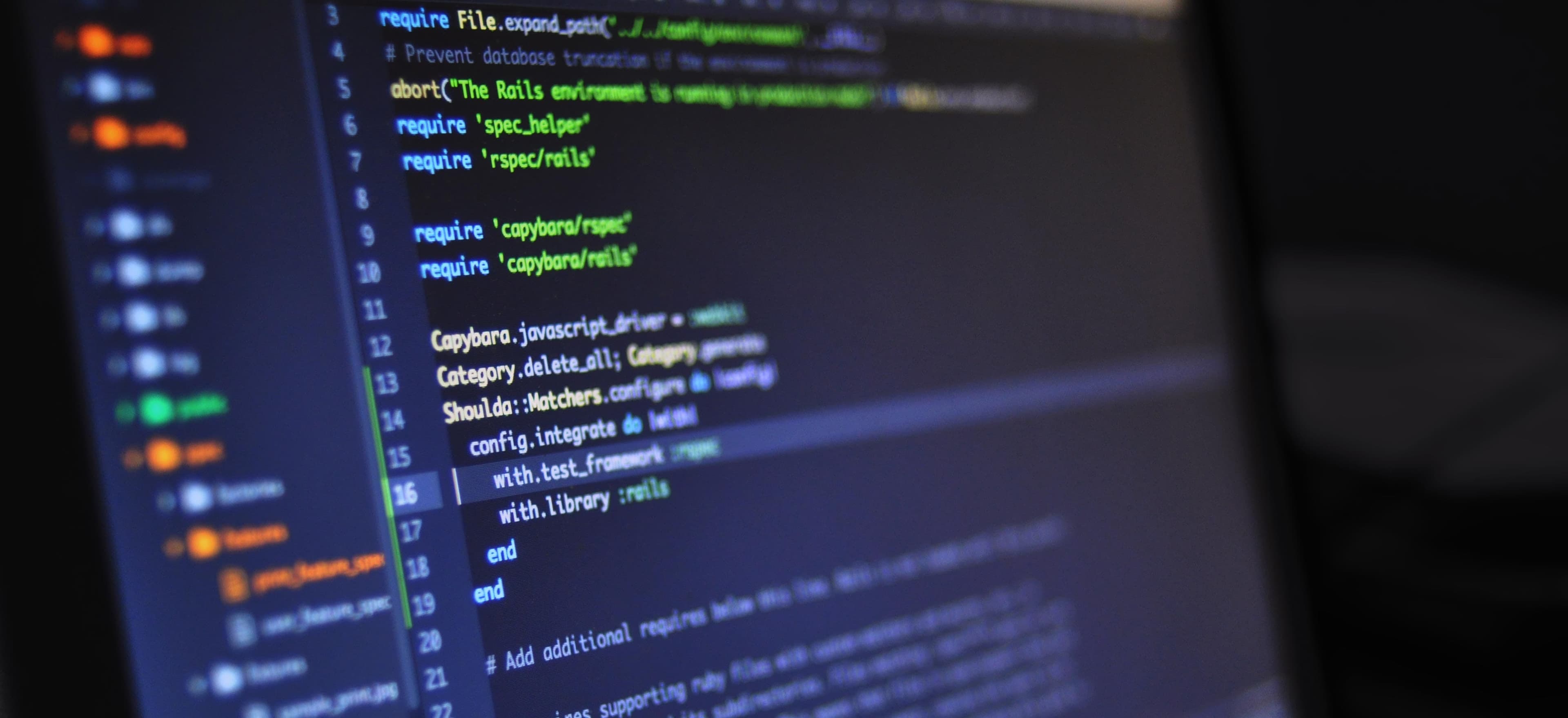
- Published on
Minimizing Security Risks When Using Third-Party Code
In today's software development landscape, it's almost inevitable to build an application without depending on third-party code. While leveraging existing libraries and frameworks can significantly expedite the development process, it also introduces potential security vulnerabilities. Therefore, it's imperative to employ best practices to minimize security risks when using third-party code.
1. Vet the Dependencies
Before integrating any third-party library into your project, ensure that you thoroughly vet it. This involves evaluating its reputation, understanding its maintenance status, and examining its security history. Reliable sources such as the GitHub repository and official documentation often provide valuable insights into these aspects.
2. Regularly Update Dependencies
Outdated dependencies can pose severe security risks. Hackers constantly look for vulnerabilities in popular libraries, and keeping your dependencies up to date can help mitigate these risks. Automating dependency updates using tools like Dependabot or Renovate can streamline this process.
3. Establish Code Review Practices
Integrating third-party code should always undergo a rigorous code review process. By involving experienced developers, you can identify potential security vulnerabilities and architectural pitfalls before they manifest in production.
4. Employ Static Code Analysis Tools
Leveraging static code analysis tools such as FindBugs and SpotBugs can help detect security vulnerabilities and coding errors within the third-party code. These tools examine the codebase without actually executing the code and provide insights into potential security risks.
Example: Using FindBugs for Static Analysis
// Sample Java code
public class Example {
public void demonstrateFindBugs() {
// Perform some operations
}
}
In this snippet, FindBugs can analyze the Example
class and provide insights into potential security risks and coding errors.
5. Implement Security Headers
When utilizing third-party code, it's essential to implement security headers to mitigate potential attacks such as Cross-Site Scripting (XSS) and Clickjacking. Frameworks like Spring Security provide robust mechanisms for incorporating security headers.
6. Monitor Security Advisories
Subscribe to security advisories for the libraries and frameworks you use. Platforms like NVD Database and OSS Index offer detailed information about reported vulnerabilities, enabling you to promptly address any security issues in your dependencies.
7. Utilize Sandboxing Techniques
Incorporate sandboxing techniques, such as Java SecurityManager, to confine the capabilities of third-party code. By defining fine-grained permissions, you can limit the potential impact of security breaches within the third-party components.
8. Validate Input Data
When interacting with third-party libraries, validate all input data rigorously. Proper input validation can thwart a plethora of security vulnerabilities, including SQL injection and command injection.
Closing Remarks
Integrating third-party code into your project can significantly enhance productivity, but it also introduces potential security vulnerabilities. By adhering to best practices such as vetting dependencies, regular updates, code reviews, and employing security headers, developers can effectively minimize security risks when using third-party code in Java applications. Additionally, leveraging static code analysis tools, monitoring security advisories, and employing sandboxing techniques are instrumental in enhancing the overall security posture of your application. Ultimately, a proactive and vigilant approach is paramount in ensuring the security and integrity of your software projects.