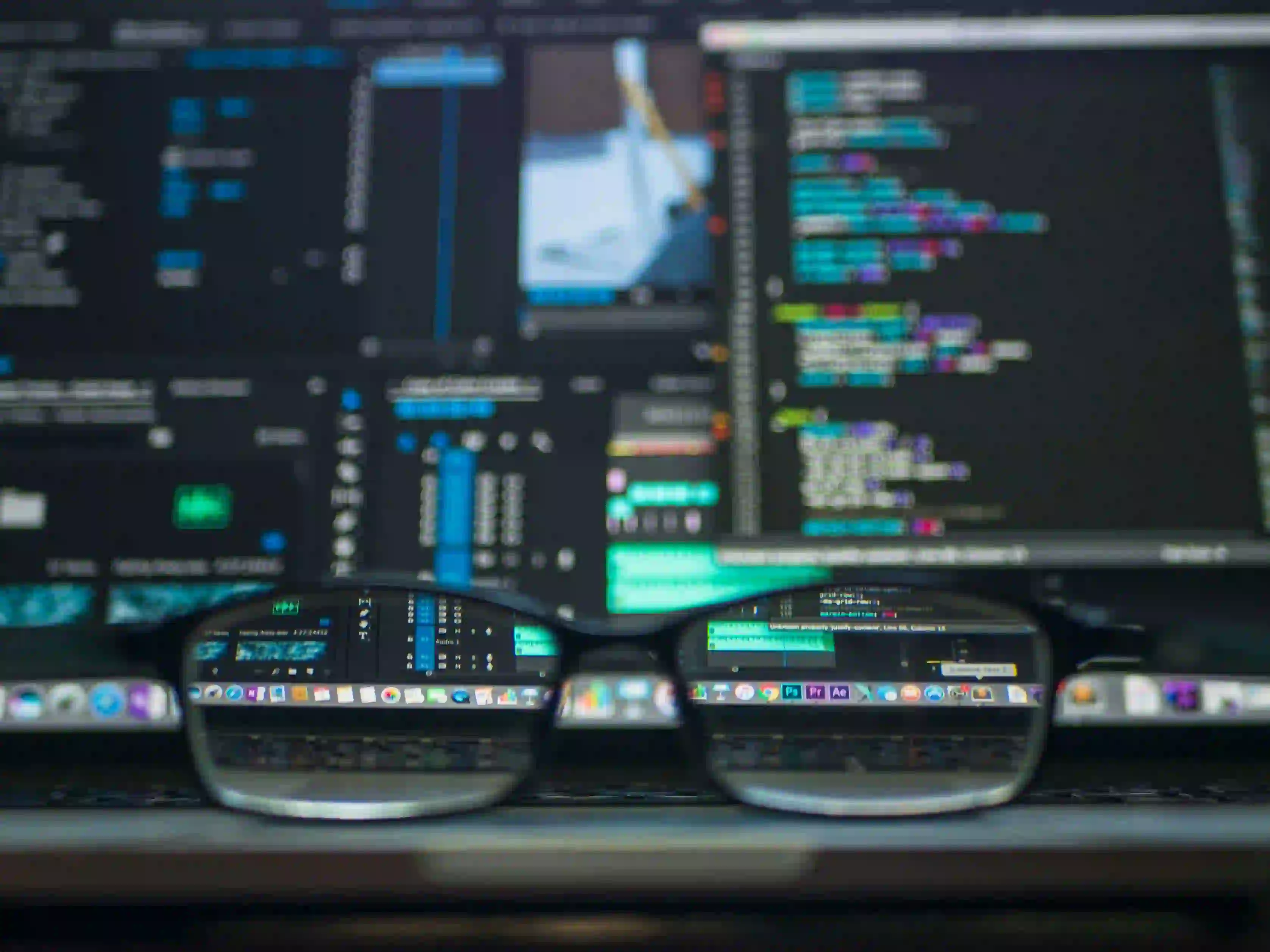
Storing JSON Data in DynamoDB using AWS SDK 2
When working with AWS's DynamoDB, it's essential to have a clear understanding of the various methods available for interacting with the service. In this blog post, we're going to delve into storing JSON data in DynamoDB using the AWS SDK 2 for Java. We'll explore the process step by step and provide concrete examples to help you implement this functionality into your own projects.
Prerequisites
Before we begin, make sure you have the following prerequisites in place:
- An AWS account
- AWS SDK for Java
- Basic understanding of DynamoDB and its operations
- Java development environment (JDK)
Setting Up AWS SDK 2 in Your Project
To start, you need to include the AWS SDK 2 for Java in your project. You can do this by adding the following dependencies to your pom.xml
file if you're using Maven:
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>dynamodb</artifactId>
<version>2.13.48</version> <!-- Replace with the latest version -->
</dependency>
If you're using Gradle, add the following to your build.gradle
file:
implementation 'software.amazon.awssdk:dynamodb:2.13.48' // Replace with the latest version
Once you've added the dependencies, make sure to refresh your project to fetch the new dependencies.
Creating a DynamoDB Table
Before storing JSON data in DynamoDB, you need to create a table. For this example, let's assume we're storing information about users in a table called UsersTable
. We'll define a userId
as the primary key for the table.
Here's a simple definition of the table using the AWS SDK 2:
import software.amazon.awssdk.services.dynamodb.DynamoDbClient;
import software.amazon.awssdk.services.dynamodb.model.*;
public class DynamoDbExample {
public static void createUsersTable() {
DynamoDbClient ddb = DynamoDbClient.create();
CreateTableRequest request = CreateTableRequest.builder()
.attributeDefinitions(
AttributeDefinition.builder()
.attributeName("userId")
.attributeType("N")
.build()
)
.keySchema(
KeySchemaElement.builder()
.attributeName("userId")
.keyType(KeyType.HASH)
.build()
)
.provisionedThroughput(
ProvisionedThroughput.builder()
.readCapacityUnits(5L)
.writeCapacityUnits(5L)
.build()
)
.tableName("UsersTable")
.build();
ddb.createTable(request);
}
}
In the above code, we're using the DynamoDbClient
to create a new table called UsersTable
with userId
as the primary key.
Storing JSON Data in DynamoDB
Now that we have our table set up, let's focus on storing JSON data in DynamoDB. To do this, we'll create a method to handle the process of converting JSON data into DynamoDB's Item
format and then store it in the table.
import software.amazon.awssdk.services.dynamodb.model.AttributeValue;
import java.util.HashMap;
import java.util.Map;
public class DynamoDbExample {
public static void storeJsonDataInDynamoDB() {
DynamoDbClient ddb = DynamoDbClient.create();
String jsonData = "{\"userId\": \"123\", \"name\": \"John Doe\", \"age\": 30}";
Map<String, AttributeValue> itemValues = new HashMap<>();
itemValues.put("userId", AttributeValue.builder().n("123").build());
itemValues.put("userData", AttributeValue.builder().s(jsonData).build());
PutItemRequest request = PutItemRequest.builder()
.tableName("UsersTable")
.item(itemValues)
.build();
ddb.putItem(request);
}
}
In the above code, we first define a JSON string representing user data. We then create a Map
of AttributeValue
pairs, where the key is the attribute name in the DynamoDB table, and the value is the corresponding AttributeValue
object. We use the PutItemRequest
to specify the table name and the item to be stored, and then we call putItem
on the DynamoDbClient
to store the JSON data in DynamoDB.
Retrieving JSON Data from DynamoDB
Conversely, if you need to retrieve JSON data from DynamoDB, you can do so by fetching the item from the table and converting the attributes back into JSON format. Here's an example of how you might achieve this:
import software.amazon.awssdk.services.dynamodb.model.GetItemRequest;
import software.amazon.awssdk.services.dynamodb.model.GetItemResponse;
public class DynamoDbExample {
public static String retrieveJsonDataFromDynamoDB() {
DynamoDbClient ddb = DynamoDbClient.create();
GetItemRequest request = GetItemRequest.builder()
.tableName("UsersTable")
.key(Map.of("userId", AttributeValue.builder().n("123").build()))
.build();
GetItemResponse response = ddb.getItem(request);
return response.item().get("userData").s();
}
}
In this code, we use the GetItemRequest
to specify the table name and the key of the item we want to retrieve. We then use the DynamoDbClient
to execute the request and obtain a GetItemResponse
. From the response, we extract the userData
attribute and convert it back to a JSON string.
The Bottom Line
In this blog post, we've covered the process of storing and retrieving JSON data in DynamoDB using the AWS SDK 2 for Java. We began by setting up the AWS SDK 2 in our project, then went on to create a DynamoDB table for storing user data. We demonstrated how to store JSON data in DynamoDB and provided an example of how to retrieve that data from the table as well.
By following these steps and code examples, you can seamlessly integrate JSON data storage into your DynamoDB workflow, enabling you to efficiently manage and retrieve complex data structures within your applications.
For more in-depth information on the AWS SDK 2 for Java and DynamoDB, be sure to check out the official AWS SDK for Java documentation and DynamoDB Developer Guide.