Optimizing Java Logging for Efficient Development
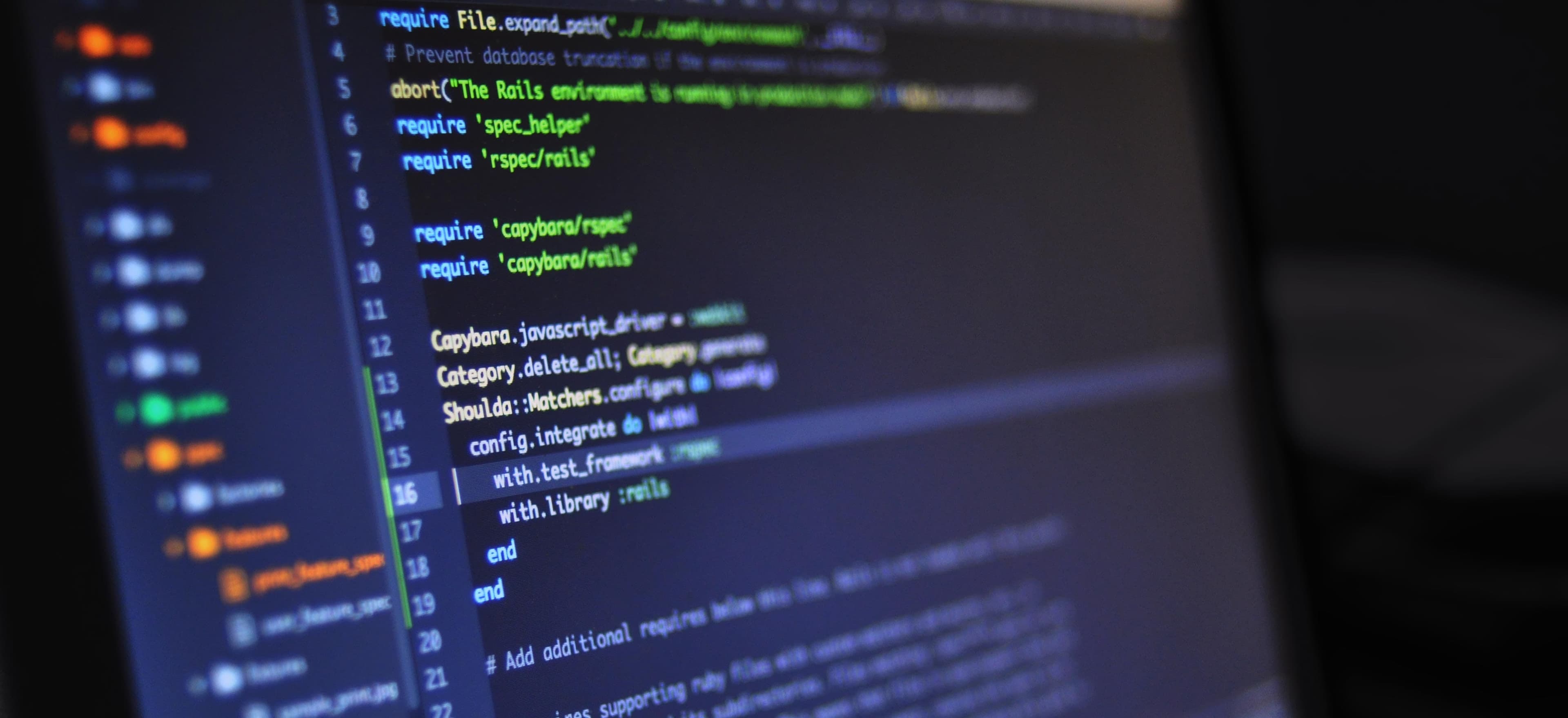
- Published on
Optimizing Java Logging for Efficient Development
Logging plays a crucial role in the development and maintenance of Java applications. A well-optimized logging system not only aids in debugging and troubleshooting but also provides valuable insights into the application's behavior and performance. In this article, we will explore various strategies to optimize Java logging for efficient development.
Importance of Logging in Java Applications
Logging is an essential aspect of Java application development as it allows developers to:
- Debug Issues: Logs provide valuable information for diagnosing and fixing issues within the application.
- Monitor Performance: Logging can be used to track the performance of the application, identifying bottlenecks, and areas for optimization.
- Audit Trail: Logs serve as an audit trail, capturing important events and actions within the application.
- Compliance and Security: Logging is crucial for compliance requirements and security audits.
- Troubleshooting in Production: Logs are indispensable for troubleshooting issues in production environments.
Now, let's dive into some best practices for optimizing Java logging.
1. Choosing the Right Logging Framework
Java offers various logging frameworks such as Log4j, Logback, and JUL (Java Util Logging). When optimizing logging in a Java application, it's crucial to choose the right logging framework based on the specific requirements and constraints of the project. For instance, Log4j is known for its flexibility and extensive configuration options, while Logback is praised for its performance and sleek design.
Let's consider an example where we choose Logback as our logging framework:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyClass {
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void myMethod() {
logger.debug("This is a debug message");
// Your method logic here
}
}
In the above code snippet, we use SLF4J with Logback as its implementation. This combination provides a high-performance logging solution.
2. Logging Levels and Their Impact
Choosing the appropriate logging level for different scenarios is vital for optimizing logging. Logging levels such as DEBUG, INFO, WARN, ERROR, and TRACE serve different purposes and have varying impacts on performance and disk space.
- DEBUG: Used for detailed debugging information. Should be disabled in production.
- INFO: Provides information on the application's state and important events.
- WARN: Indicates potential issues that do not necessarily disrupt the application's flow.
- ERROR: Logs errors that impact the normal flow of the application.
- TRACE: Provides very detailed information, more than DEBUG, and is usually used for diagnosing intricate issues.
By employing the appropriate logging levels, we can ensure that the logs are informative, while minimizing the impact on performance and storage.
3. Log Message Optimization
When logging messages, it's essential to carefully craft messages that provide meaningful context without being verbose. This optimization helps in efficiently utilizing disk space and makes log analysis more manageable.
Here's an example of optimizing log messages by providing contextual information:
public void processOrder(Order order) {
// Log the order processing
logger.info("Processing order with ID: {}", order.getId());
// Process the order
}
In the above snippet, we log a concise message with the order ID, providing valuable context without unnecessary verbosity.
4. Asynchronous Logging
In high-throughput applications, synchronous logging can introduce performance overhead. Asynchronous logging ensures that the application's performance is not significantly impacted by logging operations.
With libraries such as Log4j2, asynchronous logging can be achieved by configuring asynchronous loggers and appenders. This significantly improves the application's throughput and response times.
5. Log Rotation and Retention Policies
To prevent log files from consuming excessive disk space, it's crucial to implement log rotation and retention policies. Log rotation involves the archiving or deletion of old log files based on predefined criteria, such as file size or date.
For instance, Log4j2 provides easy configuration for log rotation and retention policies through its RollingFileAppender. By configuring the appender with a suitable policy, we can ensure that log files are managed efficiently.
6. Integration with Centralized Logging Systems
In distributed environments, integrating with centralized logging systems such as ELK (Elasticsearch, Logstash, Kibana) or Splunk can provide a centralized view of logs across multiple services. This facilitates easier log analysis, troubleshooting, and monitoring.
By using appenders and handlers provided by logging frameworks, we can seamlessly integrate our Java application with these centralized logging systems.
My Closing Thoughts on the Matter
Optimizing Java logging is crucial for efficient development, troubleshooting, and performance monitoring. By choosing the right logging framework, defining appropriate logging levels, optimizing log messages, implementing asynchronous logging, managing log rotation, and integrating with centralized logging systems, developers can ensure that logging provides maximum value with minimal impact on the application's performance. These practices contribute to a more efficient and manageable development process.
In this article, we've explored the importance of logging in Java applications and discussed various strategies for optimizing Java logging to enhance development efficiency.
When optimizing Java logging, it's essential to consider the specific requirements and constraints of the project, choose the right logging framework, and employ best practices such as managing logging levels, optimizing log messages, implementing asynchronous logging, and integrating with centralized logging systems.
By following these best practices, developers can establish a robust and efficient logging system that contributes to seamless application development and maintenance.
Logging is an integral part of Java application development, and optimizing it for efficiency is paramount for the success of any project.
Remember, efficient logging leads to efficient development!
Checkout our other articles