How to Handle Versioning in Client API Library Upgrades
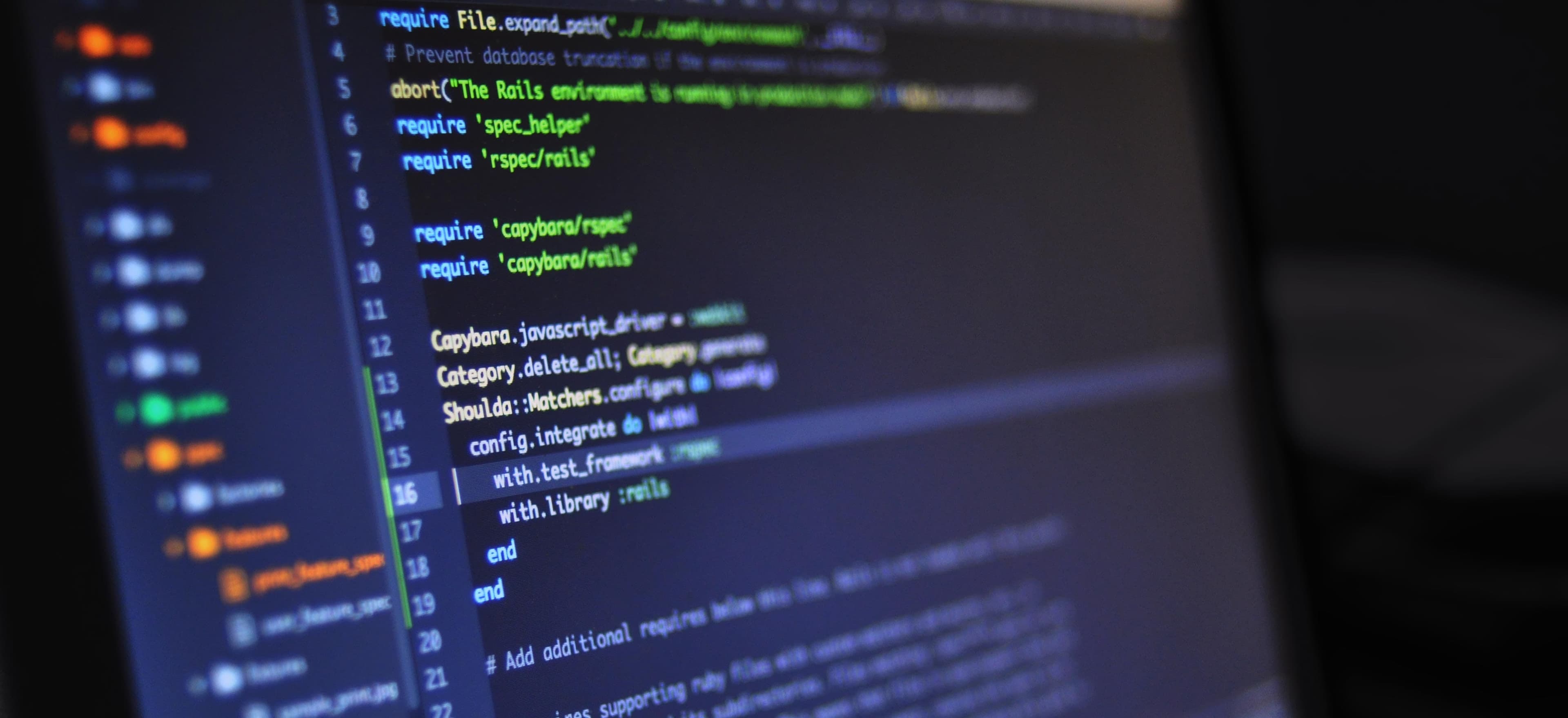
- Published on
Handling Versioning in Client API Library Upgrades
When dealing with client API library upgrades, versioning becomes a crucial aspect to ensure seamless transition without breaking changes. In this article, we'll delve into the importance of versioning, the challenges it poses, and how to effectively manage versioning during client API library upgrades in Java.
Why is Versioning Important?
Versioning is essential as it allows developers to track changes, manage dependencies, and ensure compatibility with existing codebases. When client API libraries undergo upgrades, versioning provides a structured approach to communicate changes and maintain backward compatibility.
Semantic Versioning
Semantic versioning (SemVer) is a widely adopted versioning scheme that provides a clear set of rules and requirements for version numbers. It consists of three parts: MAJOR, MINOR, and PATCH, represented as MAJOR.MINOR.PATCH
. Each part has a specific meaning:
- MAJOR version for incompatible API changes
- MINOR version for adding functionality in a backward-compatible manner
- PATCH version for making backward-compatible bug fixes
Adhering to Semantic Versioning simplifies dependency management and enables consumers of the library to make informed decisions when upgrading.
Challenges in Versioning
Ambiguous API Changes
Understanding the impact of version changes on the client's codebase can be challenging. It's crucial to provide clear release notes and documentation detailing the modifications, additions, and deprecations to facilitate a smooth transition for users.
Dependency Management
When an API library undergoes version upgrades, maintaining consistency and resolving dependencies across different modules or projects becomes complex. Careful consideration is necessary to prevent conflicts and ensure the stable functioning of the overall system.
Managing Versioning in Client API Library Upgrades
Backward Compatibility
When introducing changes in a client API library, preserving backward compatibility is paramount. Utilize techniques such as deprecation warnings, gradual phase-out of outdated methods, and providing migration guides to assist users in updating their code.
Clear Release Notes
Comprehensive release notes play a vital role in communicating version changes. Specify the modifications, bug fixes, and deprecations with examples to aid users in understanding the impact of the upgrade.
Automated Testing
Thorough test coverage, including unit tests, integration tests, and regression tests, is indispensable to validate the compatibility of the upgraded library with existing client code. Continuous integration pipelines can automate this process, ensuring that the changes do not introduce regressions.
@Test
public void testLibraryUpgradeCompatibility() {
// Write comprehensive test cases to validate the functionality
// of the upgraded client API library with existing code.
}
Dependency Resolution
Utilize dependency management tools like Apache Maven or Gradle to handle library upgrades seamlessly. Define version ranges or specific versions in the dependency declarations to control the compatibility and stability of the libraries used within the project.
Communication
Open channels of communication with the users of the client API library. Collect feedback, address concerns, and provide support during the migration process. Engaging with the community fosters a collaborative environment and aids in understanding the impact of version upgrades on diverse use cases.
Example: Retrofit Library Upgrade in Java
Let's consider the scenario of upgrading the Retrofit library, a widely used HTTP client for Java and Android, to a new version. Retrofit follows Semantic Versioning, making it an exemplary case for managing version upgrades.
Understanding Version Changes
Before initiating the upgrade, review the release notes of the newer version of Retrofit. Identify the major, minor, and patch changes, along with any deprecations or breaking modifications. Understanding these alterations is crucial for assessing the impact on the existing codebase.
Dependency Declaration
In the project's build configuration file (e.g., build.gradle
for Gradle or pom.xml
for Maven), update the Retrofit dependency to the new version. Consider specifying the version range or a fixed version based on the compatibility and stability requirements of the project.
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
Testing for Compatibility
Comprehensive testing is essential to ensure that the upgraded version of Retrofit functions seamlessly with the existing code. Execute unit tests, integration tests, and validate the behavior of API calls and response handling to detect any compatibility issues.
Handling Deprecated Functionality
If the upgraded version of Retrofit deprecates certain methods or classes, address these deprecations in the codebase. Use the provided migration guides or documentation to adapt the usage of the library to the latest conventions and features.
Continuous Integration
Integrate the upgraded Retrofit library into the continuous integration pipeline to automate the testing process. This ensures that any future changes to the codebase do not introduce regressions related to the client API library upgrade.
In conclusion, versioning plays a pivotal role in ensuring smooth client API library upgrades. By understanding the significance of semantic versioning, addressing backward compatibility, communicating effectively, and employing robust testing and dependency management practices, developers can navigate version upgrades with confidence and minimal disruption to existing systems and applications.
By following these best practices and embracing the principles of versioning, developers can streamline the process of client API library upgrades, fostering a harmonious and efficient development ecosystem in Java.
Remember, maintaining clear documentation, seeking feedback, and fostering open communication channels are as essential as writing impeccable code. With these guidelines, you're well-equipped to navigate client API library upgrades in Java effectively. Happy coding!
For more detailed information on handling library upgrades in Java, refer to Managing Dependencies in Java.