Optimizing Cloud Factory Architectural Elements
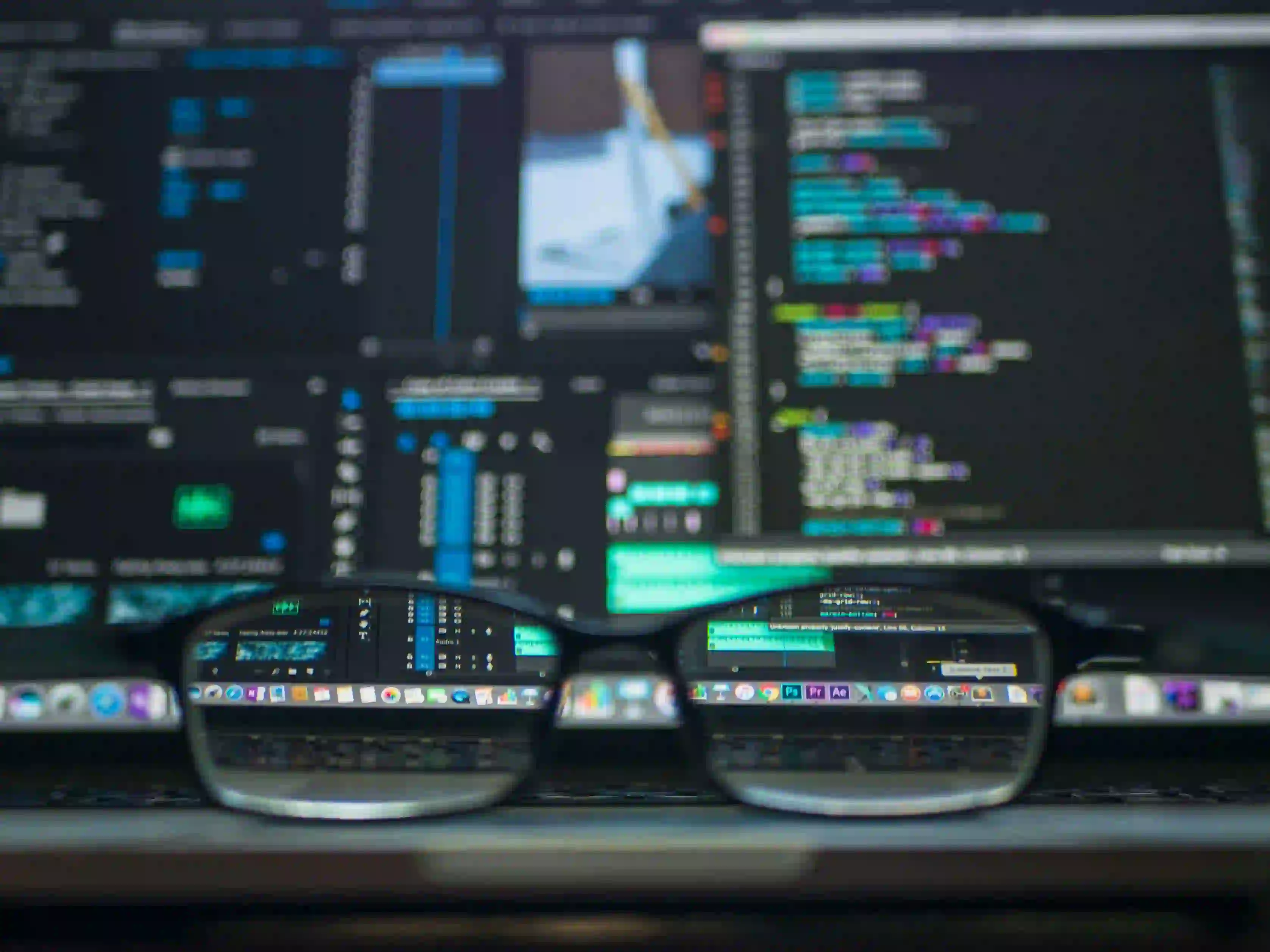
Understanding Cloud Factory Architectural Elements in Java
Cloud computing has become an essential part of modern software development, and Java is a widely used language for building applications that run on cloud infrastructure. When developing Java applications for the cloud, it's crucial to optimize the architectural elements to ensure scalability, reliability, and performance. In this article, we'll explore how to optimize the architectural elements of a Java-based cloud application, focusing on key components such as microservices, containers, and serverless computing.
Microservices Architecture
Microservices architecture is a popular approach for building cloud-native applications, where an application is composed of small, independent services that are loosely coupled and can be deployed, scaled, and managed independently. Java provides robust support for building microservices using frameworks like Spring Boot and Dropwizard. When developing microservices in Java, it's important to:
Use Lightweight Frameworks
Using lightweight frameworks such as Spring Boot allows for rapid development and deployment of microservices. Spring Boot auto-configures the application, reducing the need for manual setup and configuration, and optimizing the performance of the microservices.
Embrace Asynchronous Communication
Utilize asynchronous communication between microservices to improve responsiveness and scalability. Java provides libraries such as Reactor and Akka for building responsive and resilient microservices that can handle concurrent requests efficiently.
Implement Circuit Breaker Pattern
Incorporate the circuit breaker pattern, using libraries like Hystrix, to prevent cascading failures and improve fault tolerance in microservices. Circuit breakers can efficiently handle and recover from network issues and service failures, optimizing the reliability of the microservices architecture.
// Example of using Hystrix for circuit breaker pattern
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String sampleMethod() {
// Method implementation
}
Containerization with Docker and Kubernetes
Containerization has revolutionized the way applications are deployed and managed in the cloud. Docker and Kubernetes have emerged as the de facto standards for container orchestration and management. When deploying Java applications in containers, it's essential to optimize the containerization process:
Create Efficient Docker Images
Optimize Docker images for Java applications by using multi-stage builds to minimize the image size. This reduces the deployment time and improves the overall performance of the containers.
Utilize Kubernetes for Orchestration
Kubernetes provides powerful orchestration capabilities for containerized Java applications. Utilize features such as horizontal pod autoscaling and load balancing to optimize the scalability and reliability of Java applications running in Kubernetes clusters.
Implement Health Probes
Use Kubernetes health probes to monitor the readiness and liveness of Java application instances. By implementing custom health checks, Java applications can provide accurate status information to Kubernetes, optimizing the overall health and resilience of the application.
// Example of implementing readiness probe in Kubernetes
@Component
public class ReadinessProbe implements HealthIndicator {
@Override
public Health health() {
// Custom health check logic
}
}
Serverless Computing with Java
Serverless computing, enabled by platforms like AWS Lambda and Azure Functions, allows developers to focus on writing code without the need to manage the underlying infrastructure. When leveraging serverless computing with Java, it's important to optimize for performance and cost efficiency:
Minimize Cold Start Time
Optimize Java functions for serverless platforms to minimize cold start time. Techniques such as using lightweight initialization and pre-warming can reduce the latency of Java functions running in a serverless environment.
Efficient Resource Utilization
Ensure efficient memory allocation and resource utilization for Java functions in a serverless environment. Fine-tuning memory settings and optimizing the code for resource efficiency can significantly reduce the cost of running Java functions in a serverless architecture.
Leverage Native Integrations
Utilize native integrations provided by serverless platforms to seamlessly integrate Java functions with other cloud services. Leveraging native integrations can optimize the performance and interoperability of Java functions in a serverless architecture.
My Closing Thoughts on the Matter
Optimizing the architectural elements of Java applications for cloud computing is essential for achieving scalability, reliability, and performance. By embracing microservices architecture, containerization with Docker and Kubernetes, and serverless computing with Java, developers can build highly optimized cloud-native applications that leverage the full potential of cloud infrastructure.
As Java continues to evolve with advancements in cloud-native technologies, it's imperative for developers to stay updated with best practices and optimizations to harness the full power of the cloud.
In conclusion, optimizing the architectural elements of Java applications for cloud computing is essential for achieving scalability, reliability, and performance. By leveraging microservices architecture, containerization with Docker and Kubernetes, and serverless computing with Java, developers can build highly optimized cloud-native applications that fully exploit the potential of cloud infrastructure.
For additional insights on cloud computing with Java, check out Java Cloud Development: What You Need to Know, which delves into the latest trends and best practices in cloud-native Java development.
By optimizing the architectural elements of Java applications for cloud computing, developers can build highly optimized, scalable, and reliable solutions that fully leverage the potential of cloud infrastructure. If you're interested in diving deeper into cloud architecture design principles, take a look at Effective Java Microservices Architectures, a comprehensive guide to designing and building microservices-based applications with Java.
Remember, the key to successful cloud optimization lies in staying updated with the latest best practices and advancements in cloud-native technologies.