Mastering GraphQL: Your Essential Cheatsheet
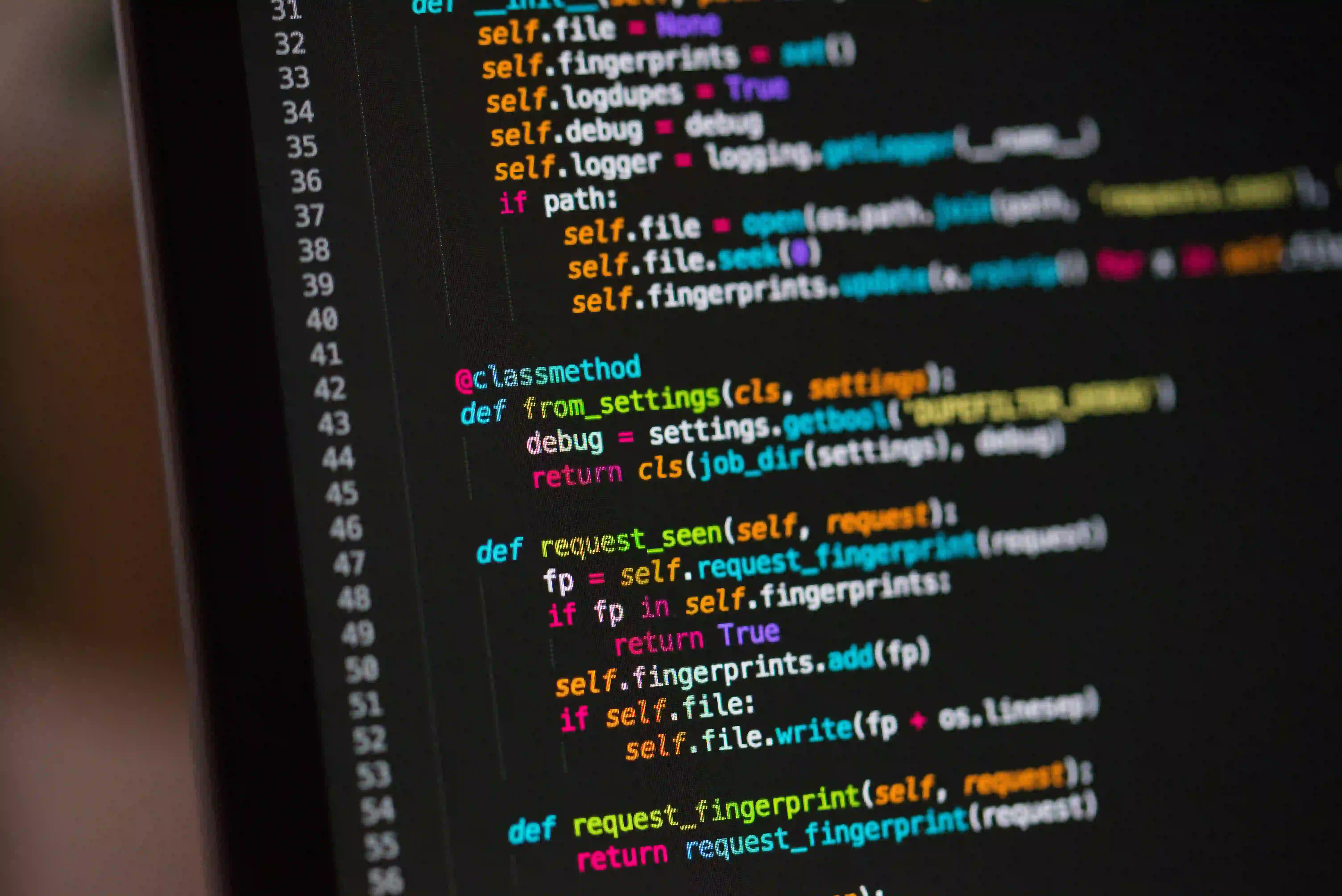
Mastering GraphQL: Your Essential Cheatsheet
GraphQL has emerged as a powerful and efficient way to manage data in modern web applications. This query language provides a more flexible, efficient, and powerful alternative to the traditional REST API. Understanding and mastering GraphQL is essential for any developer looking to stay competitive in the industry.
In this essential cheatsheet, we will dive into the core concepts of GraphQL and provide you with a concise reference to help you master this revolutionary technology.
What is GraphQL?
GraphQL is a query language for APIs and a runtime environment for executing those queries with your data. It was developed by Facebook and released as an open-source project. Unlike REST, where you have to make multiple requests to different endpoints to fetch related data, GraphQL allows you to fetch all the required data in a single request.
GraphQL vs. REST
1. Flexibility
- REST: Fixed data structure returned by the server.
- GraphQL: Clients can request only the data they need.
2. Over-fetching and Under-fetching
- REST: Over-fetching (receiving more data than needed) and under-fetching (not getting all the required data) are common issues.
- GraphQL: Clients can request only the necessary data, avoiding over-fetching and under-fetching.
3. Endpoint Management
- REST: Multiple endpoints for different resources.
- GraphQL: A single endpoint for all data operations.
Basic GraphQL Schema
A GraphQL schema is at the core of any GraphQL server implementation. It defines the types and capabilities of the API. Let's look at a basic example of a GraphQL schema:
type Query {
hello: String
}
In this schema, we have a single Query
type with a single field hello
that returns a String
.
Querying Data with GraphQL
In GraphQL, queries are used to fetch data from the server. Let's see a simple query to fetch the hello
field from the previous schema:
query {
hello
}
This query will return the value of the hello
field, which is a String
.
Mutations in GraphQL
Mutations in GraphQL are used to write or update data on the server. Here's an example of a basic mutation in GraphQL:
type Mutation {
setMessage(message: String): String
}
In this example, we have a Mutation
type with a setMessage
field that takes a String
parameter message
and returns a String
.
Resolver Functions
Resolver functions are responsible for fetching the data for the fields in the schema. Let's see an example of resolver functions in a GraphQL server using JavaScript and the popular library Apollo Server:
const resolvers = {
Query: {
hello: () => 'Hello, World!'
},
Mutation: {
setMessage: (_, { message }) => {
// Logic to set the message
return message;
}
}
};
In this example, we define resolver functions for the Query
and Mutation
types. The resolver for hello
simply returns the string 'Hello, World!', while the resolver for setMessage
sets the message and returns it.
Nested Types and Relationships
One of the powerful features of GraphQL is its ability to handle nested types and relationships. Let's consider an example where we have a User
type and a Post
type, and each user can have multiple posts:
type User {
id: ID
name: String
posts: [Post]
}
type Post {
id: ID
title: String
content: String
author: User
}
In this example, the User
type has a field posts
that returns an array of Post
objects, and the Post
type has a field author
that returns a User
object.
Querying Nested Data
With GraphQL, querying nested data is straightforward. Here's an example of a query to fetch a user with their posts:
query {
user(id: "123") {
name
posts {
title
content
}
}
}
This query will fetch the name
of the user with ID "123" and their posts
with their title
and content
.
To Wrap Things Up
Mastering GraphQL is becoming increasingly essential for developers building modern web applications. Its flexibility, efficiency, and power make it a compelling choice for managing data in today's complex applications. With this essential cheatsheet, you're now equipped with the fundamental knowledge to dive deeper into GraphQL and take your skills to the next level.
Now that you've got a good grasp of the basics, take the next step to explore more about GraphQL.
Remember, practice makes perfect, so don't hesitate to experiment with GraphQL yourself to solidify your understanding. Happy coding!