Managing Git submodules with JGit: Handling nested repositories
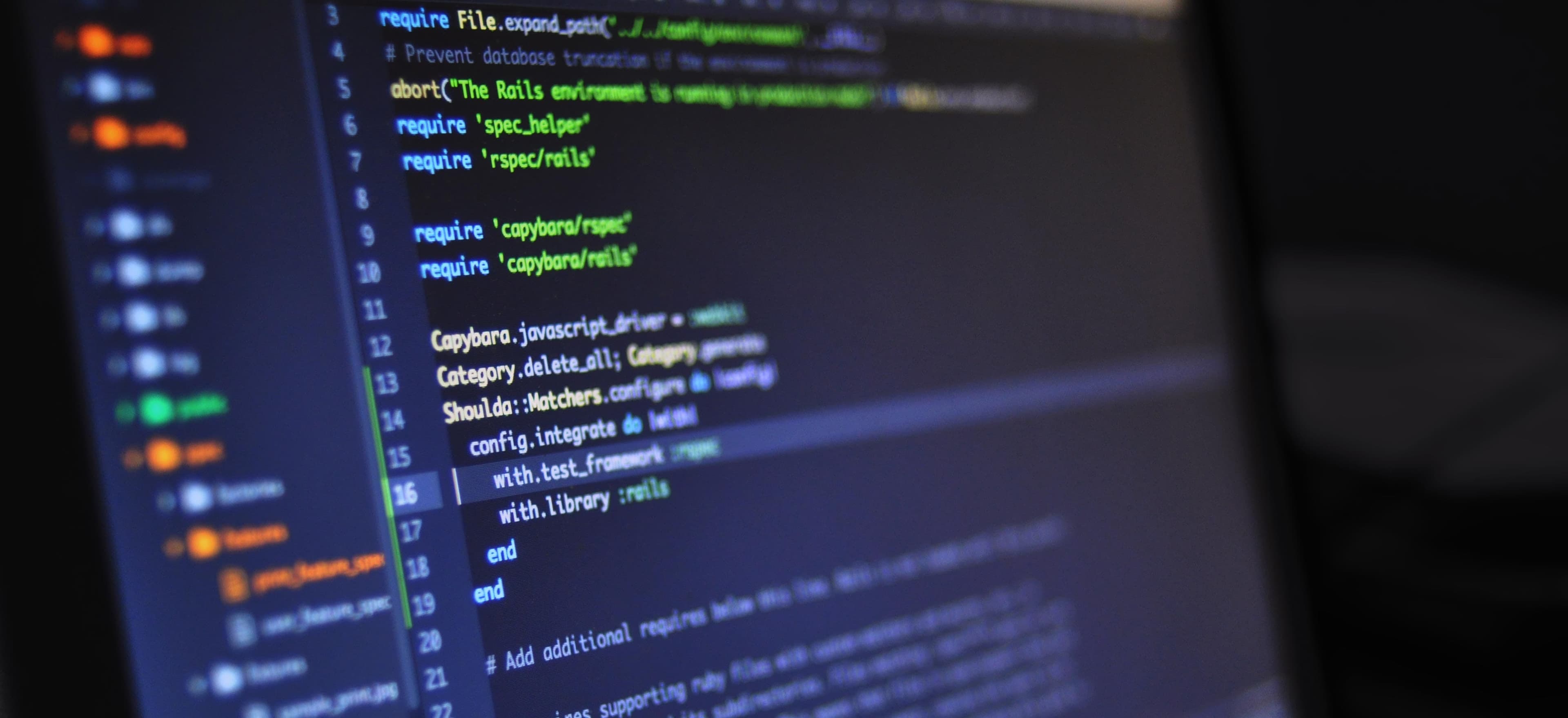
- Published on
Managing Git submodules with JGit: Handling nested repositories
Git submodules are a powerful feature that allows you to include other Git repositories as sub-folders within a parent repository. This is useful when you want to include external dependencies or libraries in your project. However, working with nested repositories can sometimes be challenging, especially when using JGit, the Java implementation of Git. In this blog post, we will explore how to effectively manage Git submodules with JGit, with a focus on handling nested repositories.
What are Git submodules?
Git submodules allow you to include other Git repositories within your own repository. This is useful for managing dependencies and keeping your project modular. When you add a submodule to your repository, Git records the exact commit that the submodule is at. This allows you to track and update the submodule independently from the parent repository.
Working with Git submodules in JGit
JGit is a lightweight, pure Java library for accessing Git repositories. It provides a powerful set of APIs for working with Git repositories programmatically. When working with Git submodules in JGit, it's important to understand how to effectively handle nested repositories.
Cloning a repository with submodules using JGit
When you clone a Git repository that contains submodules using JGit, you need to explicitly initialize and update the submodules after cloning the parent repository. This can be done using the SubmoduleWalk
class provided by JGit. Here's an example of how to clone a repository with submodules and initialize/update the submodules using JGit:
try (Git git = Git.cloneRepository()
.setURI("https://github.com/example/repository.git")
.setDirectory(new File("/path/to/clone"))
.setCloneAllBranches(true)
.call()) {
SubmoduleWalk walk = SubmoduleWalk.forIndex(git.getRepository());
while (walk.next()) {
Repository submoduleRepo = walk.getRepository();
// Initialize or update submodule repository
... // Your submodule handling logic here
}
}
In this code snippet, we use the SubmoduleWalk
class to iterate over the submodules in the cloned repository and perform the necessary operations for each submodule, such as initialization or update.
Committing changes to a repository with submodules using JGit
When you make changes to a submodule within a parent repository, you need to explicitly add and commit the changes to the parent repository using JGit. This ensures that the parent repository records the updated commit of the submodule. Here's an example of how to commit changes to a repository with submodules using JGit:
try (Git git = Git.open(new File("/path/to/repository"))) {
// Make changes to submodule
... // Your submodule changes here
// Add submodule changes to the index
git.add().addFilepattern("path/to/submodule").call();
// Commit submodule changes
RevCommit commit = git.commit()
.setMessage("Updated submodule")
.call();
}
In this code snippet, we use the git.add()
method to add the submodule changes to the index, and then use the git.commit()
method to commit the changes to the parent repository.
Handling nested repositories in JGit
Nested repositories occur when a submodule itself contains submodules. This can make managing dependencies and updates more complex. When working with nested repositories in JGit, it's important to handle the initialization and updates of nested submodules recursively.
Recursively initializing and updating nested submodules
When dealing with nested repositories in JGit, it's important to recursively initialize and update the submodules at each level of nesting. This ensures that all submodules, including nested submodules, are properly initialized and updated. Here's an example of how to recursively initialize and update nested submodules using JGit:
public void initializeNestedSubmodules(Git git) throws GitAPIException {
SubmoduleWalk walk = SubmoduleWalk.forIndex(git.getRepository());
while (walk.next()) {
Repository submoduleRepo = walk.getRepository();
// Initialize or update submodule repository
... // Your submodule handling logic here
// If the submodule itself contains submodules, recursively initialize/update them
if (submoduleRepo.hasSubmodules()) {
Git submoduleGit = Git.wrap(submoduleRepo);
initializeNestedSubmodules(submoduleGit);
}
}
}
In this code snippet, the initializeNestedSubmodules
method recursively initializes and updates nested submodules by iterating over each submodule and handling nested submodules if they exist.
Closing Remarks
Managing Git submodules with JGit, especially when dealing with nested repositories, can be challenging but also rewarding. By understanding how to effectively initialize, update, and commit changes to submodules, and by handling nested repositories recursively, you can ensure that your project's dependencies are managed efficiently.
In this blog post, we explored how to manage Git submodules with JGit, focusing on handling nested repositories. We discussed cloning repositories with submodules, committing changes to repositories with submodules, and handling nested repositories in JGit. By following these best practices, you can effectively manage nested repositories in your Git projects using JGit.
For more information on managing Git submodules with JGit, you can refer to the official JGit documentation. Additionally, you can explore the JGit API to further customize your Git submodule management logic in Java.
If you found this blog post helpful, feel free to share it with your colleagues and peers. Happy coding with JGit and Git submodules!