Overcoming Common Java Performance Issues
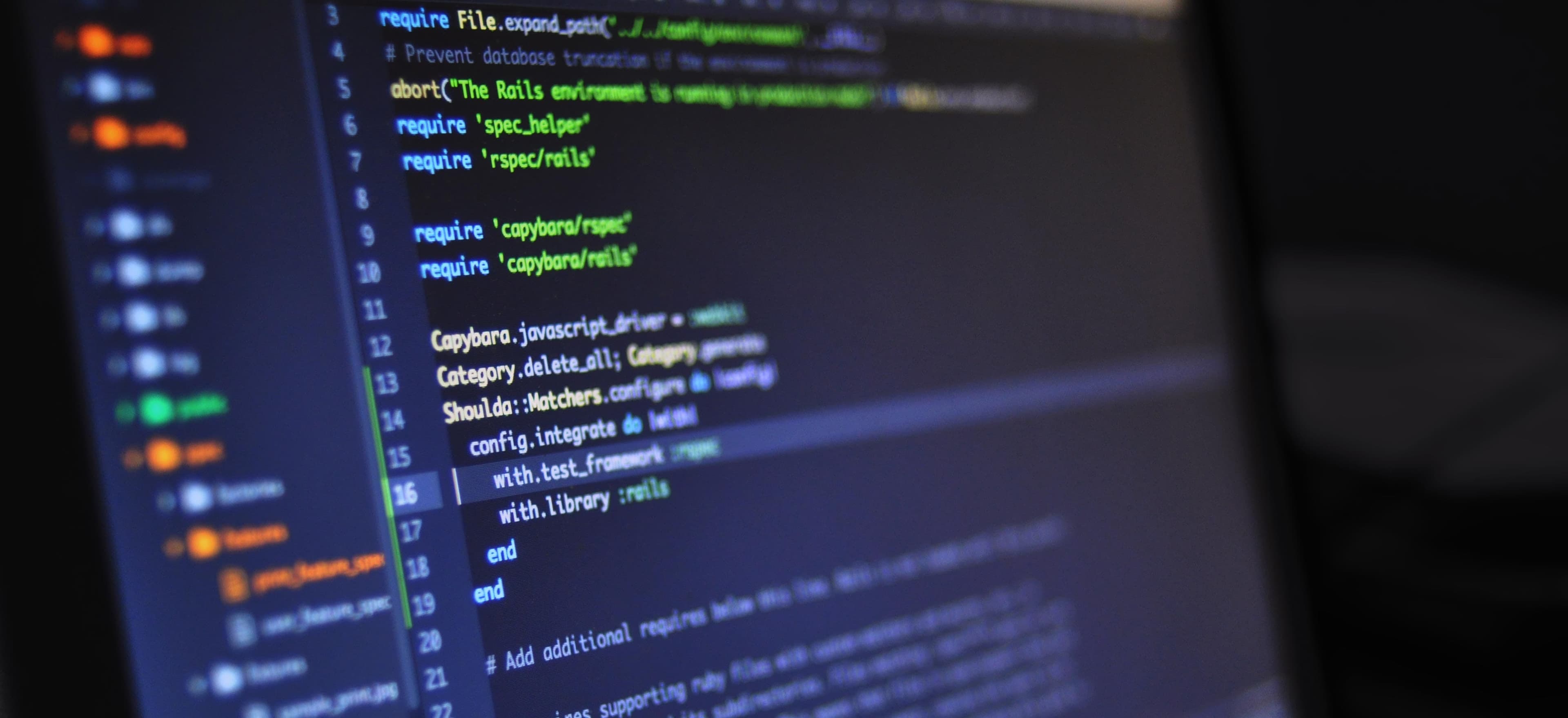
- Published on
Overcoming Common Java Performance Issues
Java is a powerful and versatile programming language, widely used in enterprise applications, mobile apps, and web development. However, Java applications can sometimes face performance issues that can degrade user experience and impact business operations. In this blog post, we will explore some common Java performance issues and discuss strategies to overcome them effectively.
1. Memory Leaks
Memory leaks occur when an application fails to release memory that is no longer in use, eventually leading to an out-of-memory error. This can severely impact the stability and performance of the Java application.
Identifying Memory Leaks
One way to identify memory leaks is by using profilers such as YourKit or VisualVM. These tools can help pinpoint areas of the code where memory is not being released properly.
Resolving Memory Leaks
One common cause of memory leaks in Java is improper handling of object lifecycles, such as not closing database connections or streams after their use. It’s important to use try-with-resources or finally blocks to ensure proper resource cleanup.
try (Connection connection = DriverManager.getConnection(url, user, password)) {
// Do database operations
} catch (SQLException e) {
// Handle exception
}
Another approach is to analyze the object references and make sure there are no unnecessary strong references that prevent objects from being garbage collected.
2. Inefficient Algorithms and Data Structures
Using inefficient algorithms and data structures can significantly impact the performance of a Java application.
Identifying Inefficiencies
Profiling tools can again be valuable in identifying areas of the code where inefficient algorithms or data structures are causing performance bottlenecks.
Resolving Inefficiencies
Utilize built-in data structures and algorithms from the Java Collections Framework, which provides efficient implementations of common data structures such as ArrayList, LinkedList, HashMap, and TreeSet.
List<String> list = new ArrayList<>(); // Prefer ArrayList over Vector for better performance
Map<String, Integer> map = new HashMap<>(); // Choose HashMap over Hashtable for improved performance
It’s also crucial to analyze the time complexity of algorithms and optimize them for better performance. For instance, using binary search instead of linear search for large datasets can significantly improve search performance.
3. Excessive Garbage Collection
Frequent garbage collection can lead to performance spikes and application pauses, causing degraded user experience.
Identifying Garbage Collection Issues
Monitoring tools like Java Mission Control and VisualVM can help in identifying excessive garbage collection and its impact on the application.
Resolving Garbage Collection Issues
One approach to mitigate garbage collection overhead is to tune the JVM parameters. Adjusting the heap size, generation sizes, and garbage collection algorithms can help optimize garbage collection performance based on the application's memory usage patterns.
java -Xmx2G -Xms512M // Set the maximum and initial heap size
java -XX:+UseG1GC // Use the G1 garbage collector for improved performance
It’s also beneficial to reduce object creation by reusing objects, utilizing object pooling where applicable, and being mindful of unnecessary object allocations.
4. Suboptimal Database Access
Inefficient database access can be a major performance bottleneck for Java applications that rely on database interactions.
Identifying Suboptimal Database Access
Database profiling tools can be used to identify slow queries, excessive round trips, and inefficient use of database indexes.
Resolving Suboptimal Database Access
Utilize database connection pooling to re-use connections and minimize the overhead of connection establishment for each request.
DataSource dataSource = // Initialize and configure the data source
try (Connection connection = dataSource.getConnection()) {
// Perform database operations
} catch (SQLException e) {
// Handle exception
}
Optimize database queries by using appropriate indexes, avoiding unnecessary joins, and fetching only the required data to reduce network overhead.
Bringing It All Together
Java performance issues can be effectively overcome by identifying the root causes and applying targeted solutions. By leveraging tools for profiling and monitoring, optimizing algorithms and data structures, tuning JVM parameters, and improving database access patterns, Java applications can deliver optimal performance and a seamless user experience.
In conclusion, proactive performance optimization is crucial for the success of Java applications, especially in the context of modern, high-demand environments. By addressing common performance issues, Java developers can ensure their applications remain responsive, scalable, and efficient.
For further insights into Java performance optimization, you can refer to the Java Performance Optimization Guide and Java Memory Management Best Practices.
Remember, consistently monitoring and fine-tuning the performance of Java applications is an ongoing process that requires attention to detail and a commitment to delivering high-quality, performant software solutions.
Start optimizing your Java applications today!