Understanding Pessimistic Locking in Hibernate
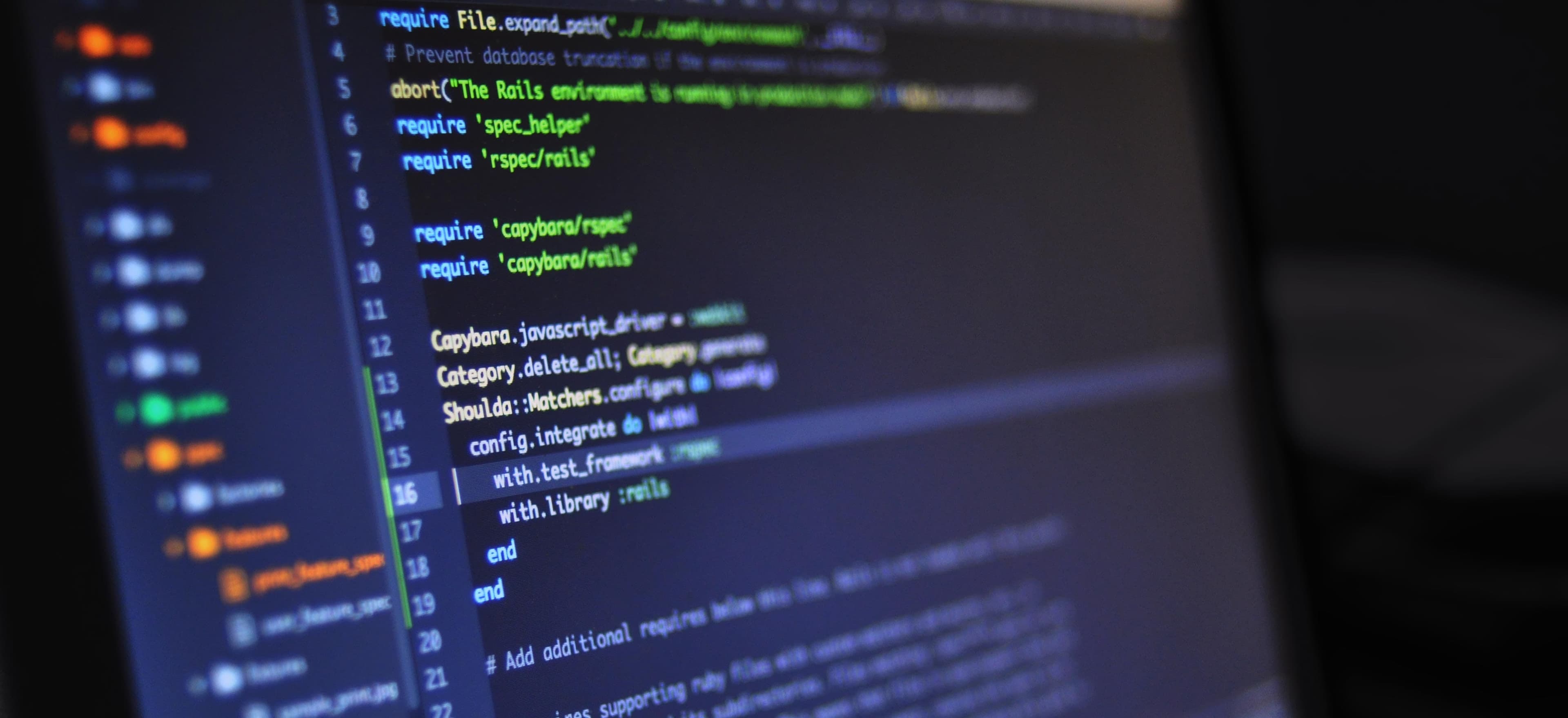
- Published on
A Comprehensive Guide to Pessimistic Locking in Hibernate
When it comes to maintaining data integrity in a multi-user database environment, concurrency control is of utmost importance. Concurrency control ensures that multiple users can access and modify the data concurrently without compromising its integrity. One popular approach to achieve this is through database locks.
In this article, we'll delve into the concept of pessimistic locking in the context of Hibernate, a widely-used object-relational mapping (ORM) tool for Java applications. We'll explore the need for pessimistic locking, its implementation in Hibernate, and provide practical examples to solidify understanding.
Understanding Pessimistic Locking
Pessimistic locking, as the name suggests, takes a cautious approach by acquiring locks on the data to prevent other transactions from modifying it. This inhibits potential conflicts and ensures that only one transaction can access the locked data at a time, thereby maintaining consistency.
In Hibernate, pessimistic locking is utilized when a transaction needs to hold a lock on a database record for an extended period, typically during a series of read-update-write operations. By applying pessimistic locks, Hibernate can prevent other transactions from interfering with the data being manipulated.
Implementing Pessimistic Locking in Hibernate
Now, let's get into the practical implementation of pessimistic locking in Hibernate. Hibernate provides a set of methods to apply pessimistic locks on entities, such as LockModeType.PESSIMISTIC_READ
and LockModeType.PESSIMISTIC_WRITE
.
Example 1: Pessimistic Locking for Read Operation
// Acquiring a pessimistic lock for read operation
entityManager.find(Employee.class, empId, LockModeType.PESSIMISTIC_READ);
In this example, the LockModeType.PESSIMISTIC_READ
ensures that the retrieved Employee
entity is locked for the duration of the transaction, preventing any concurrent write operations on it.
Example 2: Pessimistic Locking for Write Operation
// Acquiring a pessimistic lock for write operation
Employee employee = entityManager.find(Employee.class, empId, LockModeType.PESSIMISTIC_WRITE);
employee.setSalary(newSalary);
// The lock will be released at the end of the transaction
Here, the LockModeType.PESSIMISTIC_WRITE
is used to acquire a lock on the Employee
entity for a write operation, ensuring exclusive access to modify the data.
Why Use Pessimistic Locking?
You might be wondering, "When should I use pessimistic locking in my Hibernate application?" Well, the answer lies in scenarios where long transactions involving multiple database operations are prone to race conditions and need to maintain data consistency.
Suppose you have a banking application where a user is updating their account balance through a series of transactions. In such a case, applying pessimistic locks on the account record can prevent other users from concurrently modifying the balance, thus avoiding inconsistencies.
Best Practices for Pessimistic Locking
While pessimistic locking offers data consistency, it comes with its own set of considerations:
-
Minimize Lock Duration: Pessimistic locks should be held for the shortest duration possible to minimize contention and enhance concurrency.
-
Avoid Deadlocks: Careful analysis of transaction sequences and lock acquisition order is crucial to avoid potential deadlocks that can halt the application.
-
Use with Caution: Pessimistic locking can impact application performance, so it's essential to evaluate the trade-offs and use it judiciously.
The Closing Argument
In conclusion, pessimistic locking in Hibernate plays a crucial role in maintaining data consistency and integrity in concurrent transaction environments. By understanding its implementation and best practices, you can leverage pessimistic locking effectively in your Hibernate applications.
To further enhance your understanding of Hibernate and concurrency control, consider exploring how optimistic locking complements pessimistic locking in ORM frameworks like Hibernate.
With these insights, you are now equipped to make informed decisions on when and how to implement pessimistic locking in your Hibernate applications, ensuring robust concurrency control and data integrity.
So, go ahead and embrace the power of pessimistic locking in Hibernate for seamless multi-user data management!
Feel free to delve deeper into the topic by referring to the official Hibernate documentation and Java Persistence API (JPA) specification. Happy coding!