Overcoming Common REST API Integration Challenges
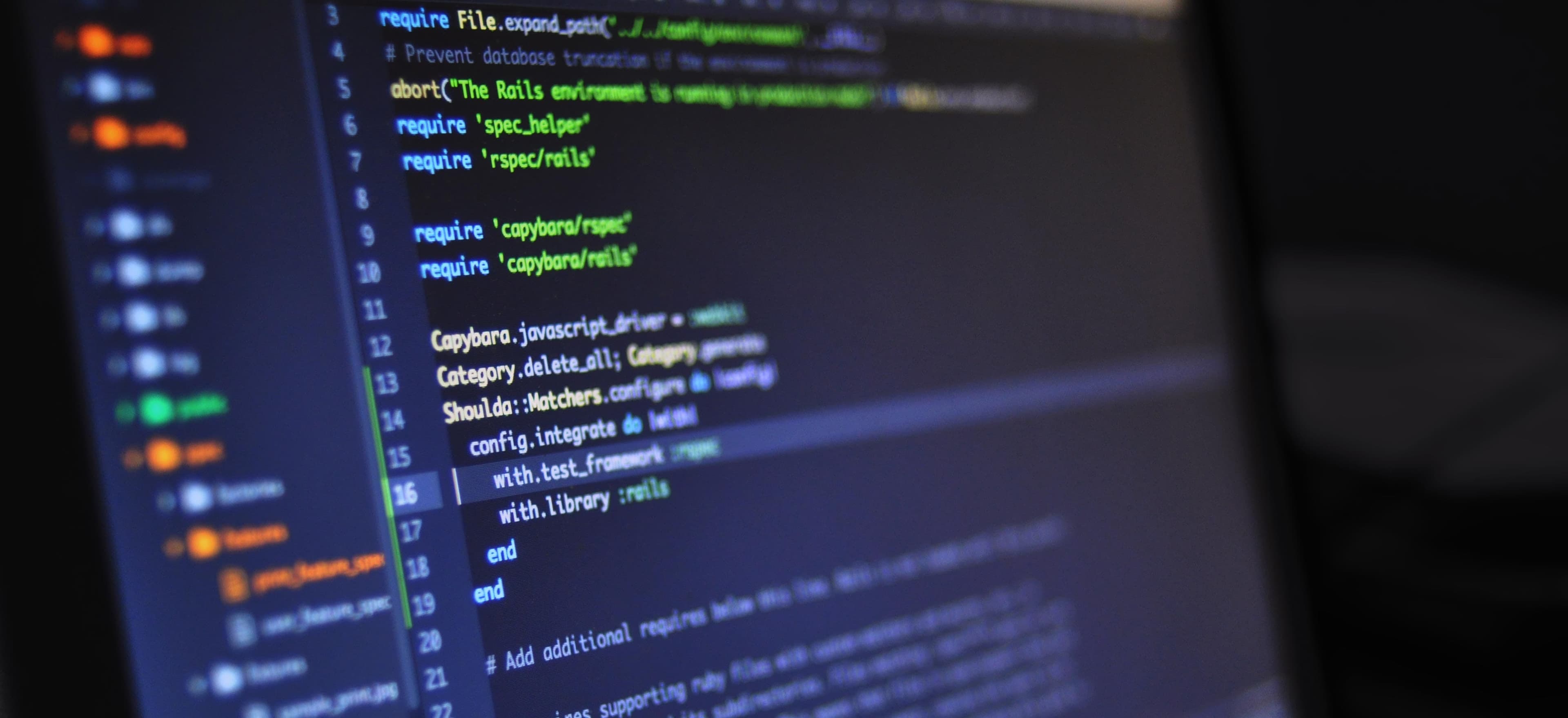
- Published on
Overcoming Common REST API Integration Challenges
In the digital world, accessing and exchanging data seamlessly is crucial. REST (Representational State Transfer) APIs have emerged as a dominant architectural style for web services due to their simplicity and scalability. However, integrating REST APIs can present various challenges. In this blog post, we will explore common REST API integration challenges and provide practical solutions to overcome them.
Understanding REST APIs
Before delving into the challenges, let's quickly recap what a REST API is. REST is an architectural style that uses standard HTTP methods (GET, POST, PUT, DELETE) for communication between a client and a server. The key principles behind REST include:
- Statelessness: Each request from the client to the server must contain all the information needed to understand and process the request.
- Resource-based: REST APIs are oriented around resources, typically represented by URLs.
- Standardized Methods: Uses standard HTTP methods to perform operations on resources.
Common Challenges in REST API Integration
1. Handling Authentication and Authorization
One of the primary challenges in integrating REST APIs is dealing with authentication and authorization. Many APIs require some form of security, often implemented through OAuth or API keys.
Solution: Use Authentication Libraries
Using established libraries that handle authentication can simplify the process. For example, libraries such as OAuth 2.0 and JSON Web Tokens (JWT) allow for secure communications. Here’s a simple example using JWT in Java:
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
public class JwtUtil {
private String secretKey = "mySecretKey";
// Generate JWT
public String generateToken(String username) {
return Jwts.builder()
.setSubject(username)
.signWith(SignatureAlgorithm.HS256, secretKey)
.compact();
}
// Validate Token
public Boolean validateToken(String token, String username) {
final String extractedUsername = extractUsername(token);
return (extractedUsername.equals(username) && !isTokenExpired(token));
}
// Extract the username
public String extractUsername(String token) {
return extractAllClaims(token).getSubject();
}
// ... other utility methods
}
Why Use This? This approach demonstrates how to securely generate and validate tokens within your Java application. Using libraries helps manage signatures and token expirations efficiently.
2. Error Handling and Status Codes
APIs may fail or return errors due to various reasons, such as network issues, incorrect parameters, or server-side problems. Understanding the various HTTP status codes (e.g., 404, 500) is essential for effective error handling.
Solution: Centralized Error Handling
Creating a centralized error handler can streamline how errors are managed in your application. Here's a simple implementation in Spring Boot:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse(ex.getMessage(), HttpStatus.NOT_FOUND);
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleGenericException(Exception ex) {
ErrorResponse errorResponse = new ErrorResponse("An unexpected error occurred", HttpStatus.INTERNAL_SERVER_ERROR);
return new ResponseEntity<>(errorResponse, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Why Use This? By using a global exception handler, you ensure that your application can respond gracefully to errors without cluttering your main application logic.
3. Versioning the API
API changes are inevitable. Without proper versioning, existing clients can break when new features or changes are deployed.
Solution: Use URL Versioning
A straightforward way to implement versioning is through the URL. For example:
GET /api/v1/users
GET /api/v2/users
In your Java application, you can separate the logic accordingly:
@RestController
@RequestMapping("/api/v1/users")
public class UserControllerV1 {
@GetMapping
public List<User> getUsers() {
// Logic for V1
}
}
@RestController
@RequestMapping("/api/v2/users")
public class UserControllerV2 {
@GetMapping
public List<UserDTO> getUsers() {
// Logic for V2
}
}
Why Use This? URL versioning allows you to make extensive modifications to the API's behavior without disrupting existing clients.
4. Managing Rate Limits
Many APIs enforce rate limits to protect against abuse. Hitting the limit can result in blocked requests and a less-than-stellar user experience.
Solution: Implement Client-Side Throttling
Implementing client-side throttling can help manage how many requests you send within a given time. Here’s a simple rate-limiting mechanism in Java:
import java.util.concurrent.TimeUnit;
public class RateLimiter {
private final long maxRequests;
private long lastRequestTime = 0;
private long requestCount = 0;
public RateLimiter(long maxRequests) {
this.maxRequests = maxRequests;
}
public synchronized boolean tryAcquire() {
long now = System.currentTimeMillis();
if (now - lastRequestTime > TimeUnit.SECONDS.toMillis(1)) {
requestCount = 0;
lastRequestTime = now;
}
if (requestCount < maxRequests) {
requestCount++;
return true;
}
return false;
}
}
Why Use This? A rate limiter helps avoid hitting API limits, protecting your application from unnecessary failures.
5. Data Transformation
Often, the data format between your client and the API doesn’t match perfectly. You may need to transform data before making requests or after receiving responses.
Solution: Use Mapping Libraries
Libraries like Jackson or Dozer in Java can help automate these transformations. Here's an example with Jackson:
import com.fasterxml.jackson.databind.ObjectMapper;
public class UserTransformer {
private ObjectMapper objectMapper = new ObjectMapper();
// Convert API response to user object
public User mapApiResponseToUser(String apiResponse) throws IOException {
return objectMapper.readValue(apiResponse, User.class);
}
// Convert user object to API request
public String mapUserToApiRequest(User user) throws JsonProcessingException {
return objectMapper.writeValueAsString(user);
}
}
Why Use This? This minimizes manual data conversion, leading to cleaner code and fewer errors.
6. CORS Configuration
Cross-Origin Resource Sharing (CORS) allows web applications to bypass the same-origin policy. Ensuring that your API supports CORS correctly is necessary when integrating with front-end applications.
Solution: Configure CORS in Spring Boot
You can enable CORS in your Spring Boot application easily:
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("https://your-client-url.com")
.allowedMethods("GET", "POST", "PUT", "DELETE");
}
}
Why Use This? Proper CORS configuration allows web applications to securely interact with your APIs from various origins.
To Wrap Things Up
Integrating REST APIs can certainly pose challenges, but by implementing robust solutions and best practices, developers can navigate these hurdles effectively. Properly addressing issues around authentication, error handling, versioning, rate limits, data transformation, and CORS configuration will lead to smoother API integrations and better application performance.
If you're interested in diving deeper into REST API best practices, consider checking out the RESTful API Design Rulebook or the documentation for REST APIs. By continuing to learn and adapting to the latest practices, you'll be well-equipped to tackle any API integration challenge that comes your way.