Prevent Unnecessary Spring Component Scanning
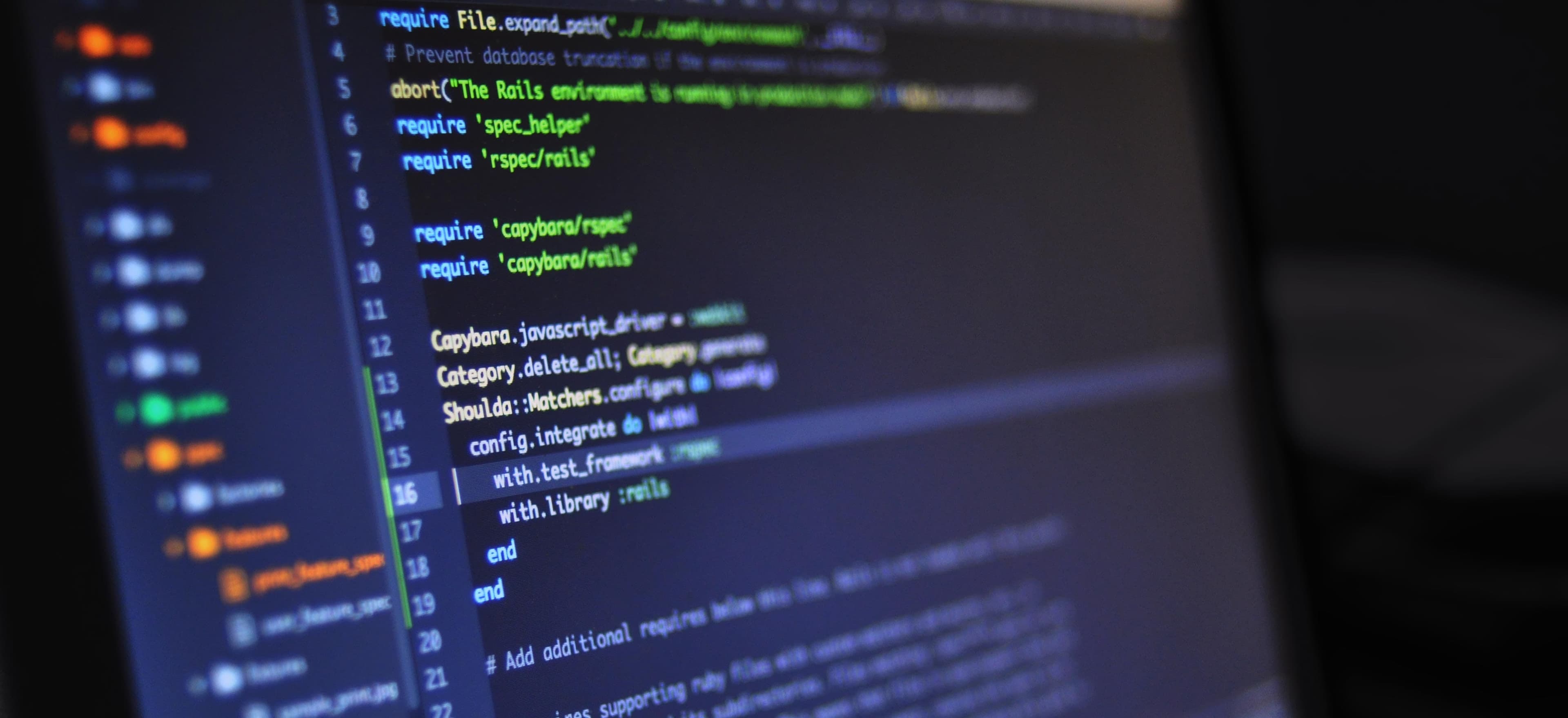
- Published on
How to Prevent Unnecessary Spring Component Scanning
When working with large Spring applications, component scanning is a vital aspect of the framework. However, component scanning can become a performance bottleneck in some cases. Unnecessary scanning can lead to longer startup times and increased memory consumption.
In this post, we will discuss how to prevent unnecessary component scanning in your Spring application to enhance performance. We will explore different strategies and best practices to optimize component scanning and improve the overall efficiency of your application.
Understanding Component Scanning
In Spring, component scanning is the process of automatically discovering and registering Spring-managed components, such as @Component
, @Service
, @Repository
, and @Controller
classes, within the application context. Spring scans the specified packages and registers the annotated components so that they can be injected and used throughout the application.
While component scanning is essential for creating a modular and easy-to-maintain application, excessive scanning can lead to performance issues, especially in large codebases with numerous classes and packages.
Using Base Packages
One way to prevent unnecessary component scanning is by specifying base packages for scanning. By explicitly defining the packages to scan, you can avoid scanning the entire application classpath, which can be resource-intensive.
To configure base packages for component scanning, you can use the @ComponentScan
annotation at the application's main configuration class, specifying the base packages as follows:
@Configuration
@ComponentScan(basePackages = {"com.example.package1", "com.example.package2"})
public class AppConfig {
// Configuration settings and bean definitions
}
By explicitly defining the base packages, you can restrict the scanning process to specific areas of your application, thus improving startup times and reducing unnecessary resource consumption.
Using Component Scan Filters
In addition to specifying base packages, you can further fine-tune component scanning by using include and exclude filters. This allows you to selectively include or exclude certain types from the scanning process based on custom criteria.
For example, you can use include filters to only scan for classes that implement a specific interface:
@Configuration
@ComponentScan(basePackages = {"com.example.package"})
@ComponentScan.Filter(type = FilterType.ASSIGNABLE_TYPE, classes = MyInterface.class)
public class AppConfig {
// Configuration settings and bean definitions
}
In this example, only classes that implement MyInterface
will be included in the component scanning process, reducing unnecessary scanning of unrelated classes.
Similarly, exclude filters can be used to exclude specific classes or packages from the scanning process, further optimizing the component scanning mechanism.
Using Classpath Scanning Limitations
Another method to prevent unnecessary component scanning is by limiting the classpath scanning to specific jars or packages. Spring provides options to define the classpath scanning limitations, preventing the framework from scanning irrelevant or third-party libraries.
You can use the @ComponentScan
annotation with the basePackageClasses
attribute to specify specific classes as the starting point for component scanning:
@Configuration
@ComponentScan(basePackageClasses = {MyComponent.class, MyAnotherComponent.class})
public class AppConfig {
// Configuration settings and bean definitions
}
By limiting the classpath scanning to specific classes, you can avoid scanning unnecessary third-party or system classes, thereby improving the overall application startup performance.
Analyzing and Profiling Scanning Activity
To identify and address unnecessary component scanning, it's crucial to analyze and profile the scanning activity of your Spring application. Utilizing tools like VisualVM, YourKit, or Java Mission Control can provide insights into the scanning process, revealing areas of improvement and potential optimization opportunities.
Profiling the component scanning activity allows you to pinpoint classes or packages that contribute to excessive scanning, enabling you to take targeted actions to optimize the scanning mechanism.
My Closing Thoughts on the Matter
In conclusion, optimizing component scanning in your Spring application is essential for improving startup times and overall performance. By carefully defining base packages, utilizing include and exclude filters, limiting classpath scanning, and analyzing scanning activity, you can prevent unnecessary scanning and enhance the efficiency of your application.
Efficient component scanning contributes to a more responsive and scalable application, making it a crucial aspect of Spring application development.
By implementing the strategies discussed in this post, you can effectively prevent unnecessary component scanning and ensure the optimal performance of your Spring application.