Uncovering the Hidden Costs of Traditional Development
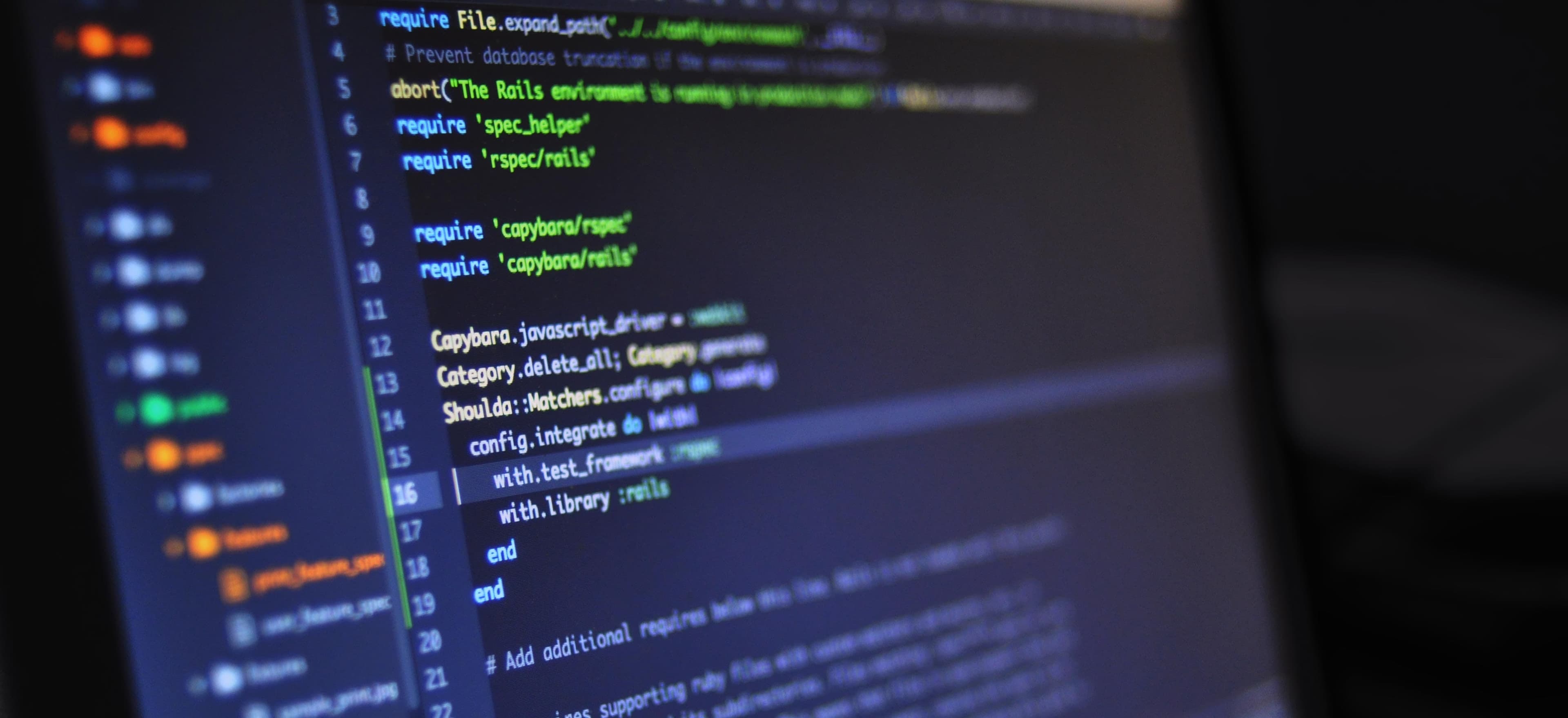
- Published on
Uncovering the Hidden Costs of Traditional Development
In the realm of software development, Java continues to hold its ground as a versatile and powerful language. Its ability to provide cross-platform support, strong performance, and extensive libraries makes it a popular choice. However, even with its prowess, there are often hidden costs associated with traditional Java development that developers and businesses may not initially consider. In this article, we will delve into some of these hidden costs and explore how they can impact a project.
The Overhead of Manual Memory Management
Java's automatic memory management, facilitated by garbage collection, is one of its most beloved features. It frees developers from manually allocating and deallocating memory, thus reducing the occurrence of memory leaks and segmentation faults. However, this convenience comes at a cost. The garbage collector (GC) periodically pauses the application to perform its tasks, leading to potential performance hiccups. Furthermore, improper object lifecycle management can inadvertently cause memory leaks, impacting the application's stability and performance.
// Example of Java garbage collection
public class MemoryManagement {
public static void main(String[] args) {
while (true) {
// Memory-intensive operations
}
}
}
In this example, the continuous memory-intensive operations would eventually trigger the garbage collector, resulting in application pauses and reduced performance.
Complexity of Multi-Threading
While multi-threading in Java allows for efficient concurrent execution, it also introduces complexities that can amplify development costs. Identifying and mitigating race conditions, deadlocks, and thread interference demands meticulous attention to detail. Additionally, the debugging of multi-threaded applications can be arduous, as issues may arise intermittently due to the non-deterministic nature of thread scheduling.
// Example of Java multi-threading
public class MultiThreading {
public static void main(String[] args) {
Thread threadA = new Thread(() -> {
// Thread A operations
});
Thread threadB = new Thread(() -> {
// Thread B operations
});
threadA.start();
threadB.start();
}
}
In this scenario, ensuring proper synchronization and handling of shared resources between threadA
and threadB
adds complexity to the development process.
Version Compatibility and Support
Java's rich ecosystem encompasses numerous libraries, frameworks, and tools, each with its own release cycle and version dependencies. Ensuring compatibility across these components, especially when transitioning to newer Java versions, can lead to unexpected challenges. Legacy code may require adjustments to align with updated APIs and language features, consuming additional time and resources.
Mitigating Hidden Costs
To address these hidden costs and streamline Java development, several strategies can be employed:
Utilize Profiling Tools
Profiling tools such as Java Mission Control and VisualVM provide insights into garbage collection behavior, thread utilization, and memory allocation. By pinpointing performance bottlenecks, developers can optimize resource utilization and minimize the impact of automatic memory management.
Embrace Concurrency Utilities
Java's Concurrency Utilities, including the Executor framework and concurrent data structures, offer higher-level abstractions for managing concurrent tasks. Leveraging these utilities reduces the intricacies associated with low-level thread management and enhances code maintainability.
Automated Compatibility Testing
Implementing automated compatibility tests using tools like JUnit ensures seamless integration of Java updates and minimizes the effort required to maintain version compatibility. Continuous testing aids in identifying compatibility issues early in the development cycle, averting last-minute surprises.
In Conclusion, Here is What Matters
While Java remains a stalwart in the world of software development, it's crucial to recognize and address the hidden costs that can accompany traditional Java development. By embracing best practices, leveraging advanced tools, and adopting modern approaches to concurrency, developers can mitigate these costs and fortify their Java projects for sustainable success.
By shedding light on these hidden costs and offering actionable strategies, we can pave the way for more efficient and cost-effective Java development that aligns with the contemporary demands of software engineering.
In conclusion, the allure of Java's flexibility and performance is undeniable, but it's essential to navigate the hidden costs with foresight and proactive measures.