The Impact of Logging on System Performance
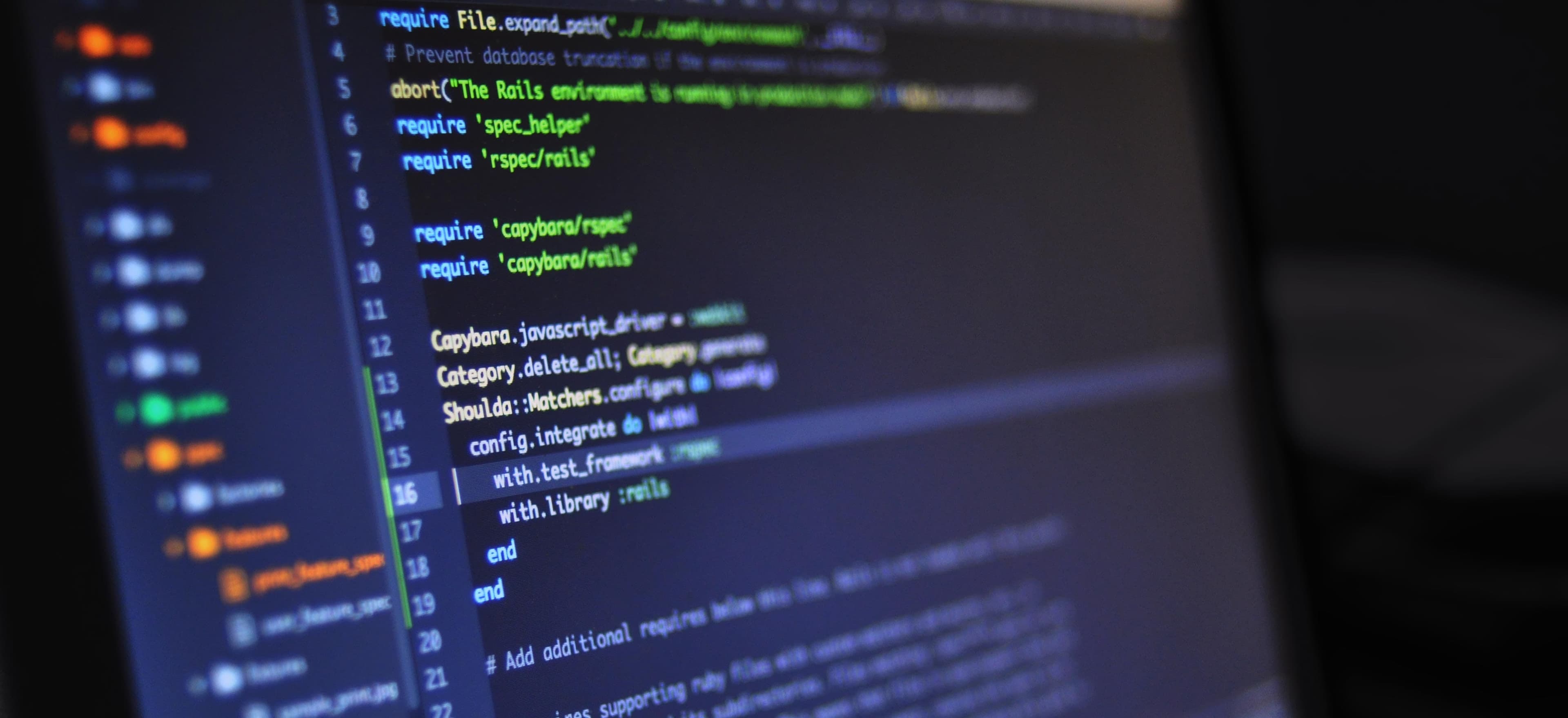
- Published on
The Impact of Logging on System Performance
In the world of software development, logging is an essential practice for monitoring and debugging applications. Logging involves recording relevant information about the execution of a program to provide insight into its behavior. While logging is crucial for identifying and diagnosing issues, it can also have a significant impact on system performance if not implemented thoughtfully. In this blog post, we will explore the importance of logging, its potential impact on system performance, and best practices to mitigate any adverse effects.
The Importance of Logging
Logging plays a crucial role in understanding the behavior of an application. It provides visibility into various aspects of an application's execution, including error conditions, warning messages, informational events, and performance metrics. By examining log data, developers and system administrators can gain valuable insights into the runtime behavior of an application, troubleshoot issues, and monitor system performance.
Properly implemented logging can also aid in auditing, compliance, and security monitoring, helping to ensure that an application operates as intended and meets regulatory requirements. Additionally, logging is valuable for post-mortem analysis, allowing teams to understand the sequence of events leading up to failures or unexpected behaviors.
The Impact of Logging on System Performance
While logging is crucial for understanding and maintaining a healthy application, it is not without its drawbacks. Excessive or inefficient logging can have a substantial impact on system performance, leading to increased resource consumption and degraded responsiveness. Here are some ways in which logging can impact system performance:
Disk I/O and Storage
Logging often involves writing data to disk, which can introduce latency and consume valuable I/O resources. If an application generates a high volume of log messages or writes verbose logs to disk frequently, it can lead to increased disk I/O operations, potentially impacting the overall responsiveness of the system.
CPU Utilization
Logging operations, especially when involving serialization and formatting of log messages, can consume CPU resources. This becomes more pronounced in scenarios where complex objects or data structures are being logged without proper consideration for performance implications.
Memory Usage
Inefficient handling of log data can result in excessive memory usage. For example, holding excessively large log buffers in memory or retaining log entries for extended periods can strain the available memory resources, leading to increased garbage collection overhead and potential memory pressure.
Network Overhead
In distributed systems, logging often involves transmitting log data over the network to centralized logging services or storage. This can introduce network overhead, especially in high-traffic environments, impacting the overall network performance and bandwidth utilization.
Best Practices for Mitigating the Impact of Logging on System Performance
To mitigate the potential impact of logging on system performance, it is essential to follow best practices for implementing and managing logging within an application. By adopting the following practices, developers can strike a balance between gaining valuable insights from logs and minimizing performance overhead:
1. Log Level and Granularity
Carefully consider the appropriate log levels (e.g., DEBUG, INFO, WARN, ERROR) for different types of log messages. Avoid excessive verbosity in production environments and utilize fine-grained log levels for diagnosing specific issues. Restrict verbose logging to non-production environments where detailed debugging is necessary.
2. Asynchronous Logging
Utilize asynchronous logging mechanisms to offload log processing from the critical path of the application. Asynchronous logging ensures that log operations do not block the main execution flow, allowing the application to continue functioning without being significantly affected by logging overhead.
3. Log Message Formatting
Opt for efficient log message formatting techniques to minimize CPU overhead. Avoid expensive string concatenation or formatting operations, especially for log messages that are frequently generated. Consider using parameterized logging or structured logging to defer message construction until it is necessary.
4. Log Aggregation and Rotation
Implement log aggregation and rotation strategies to manage log files effectively. Periodically rotate log files to prevent them from growing excessively and consuming excessive disk space. Consider leveraging log aggregation tools for consolidating log data from multiple sources and managing log retention policies.
5. Monitoring and Profiling
Regularly monitor the impact of logging on system performance using profiling tools and monitoring solutions. Evaluate the resource consumption attributed to logging operations and identify areas for optimization. Profiling can reveal potential bottlenecks related to logging, helping to fine-tune logging configurations for minimal performance impact.
6. Contextual Logging
Include contextual information in log messages to aid in correlation and analysis. Incorporate relevant contextual data such as request identifiers, user sessions, and transaction IDs to facilitate traceability and diagnostics. Contextual logging can streamline the process of understanding the flow of operations across distributed systems.
Code Example: Asynchronous Logging in Java
Let's explore a code example demonstrating how to implement asynchronous logging in a Java application using the Logback framework. Asynchronous logging ensures that log operations are processed in a separate thread, preventing them from blocking the main application flow.
import ch.qos.logback.classic.Logger;
import org.slf4j.LoggerFactory;
public class AsynchronousLoggingExample {
private static final Logger logger = (Logger) LoggerFactory.getLogger(AsynchronousLoggingExample.class);
public static void main(String[] args) {
// Configure Logback for asynchronous logging
System.setProperty("logback.configurationFile", "path/to/logback.xml");
// Perform application logic
logger.info("Application started");
// ...
logger.info("Application finished");
}
}
In the above example, the Logback framework is used for logging, and the asynchronous logging behavior is configured via the logback.xml
configuration file. By offloading logging operations to a separate thread, the impact of logging on the main application logic is mitigated.
In Conclusion, Here is What Matters
In conclusion, logging is an indispensable tool for understanding the runtime behavior of an application and diagnosing issues. However, it is crucial to be mindful of the potential impact of logging on system performance. By following best practices such as careful log level management, asynchronous logging, efficient message formatting, and proactive monitoring, developers can ensure that logging overhead is minimized without sacrificing valuable insights from logs.
As the saying goes, "with great logs comes great responsibility." By striking a balance between insightful logging and minimal performance impact, developers can unleash the full potential of logging as a powerful tool for maintaining and monitoring the health of their applications.
In the ever-evolving landscape of software development, the orchestration of logging practices will continue to be an essential consideration for building high-performing and resilient systems.
Are there other aspects of logging and system performance that you find particularly challenging? Let's keep the conversation going.