Revisiting Decorator Pattern in Java EE
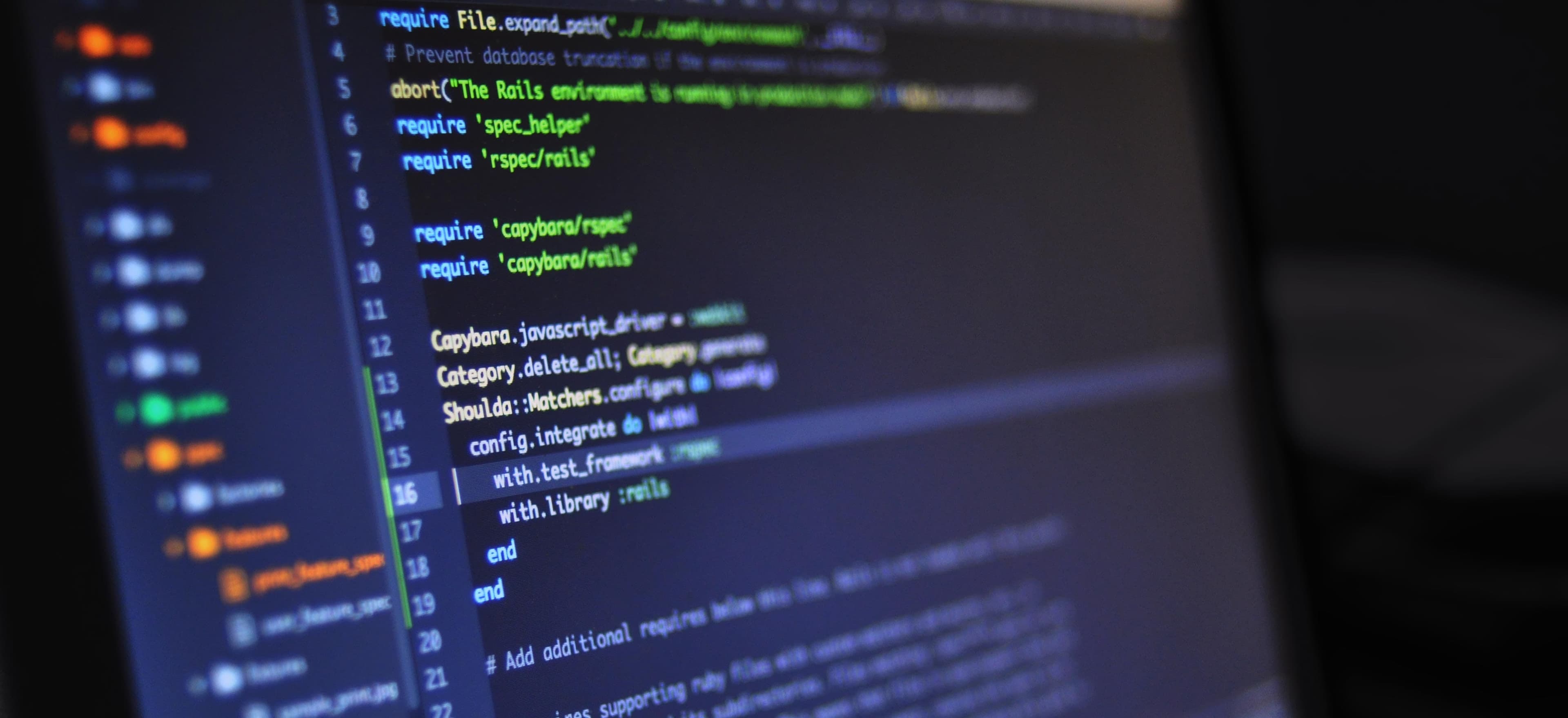
- Published on
Revisiting Decorator Pattern in Java EE
In the world of software development, patterns can be incredibly powerful tools for creating maintainable, scalable, and flexible code. One such pattern that is commonly used in Java EE is the Decorator pattern. The Decorator pattern allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class. This blog post aims to revisit the Decorator pattern in Java EE, exploring its implementation, use cases, and benefits.
What is the Decorator Pattern?
The Decorator pattern is a structural pattern that allows behavior to be added to objects at runtime. It is useful for modifying the functionality of individual objects without affecting other objects of the same class. In essence, the Decorator pattern is all about adding additional responsibilities to objects dynamically.
Implementing the Decorator Pattern in Java EE
Let's explore how the Decorator pattern can be implemented in Java EE. Consider a simple example of a Coffee
interface and its concrete implementation SimpleCoffee
. We want to be able to add condiments, such as milk or sugar, to the coffee without altering the base SimpleCoffee
class.
Step 1: Define the Coffee Interface
public interface Coffee {
double getCost();
String getIngredients();
}
Step 2: Create the Concrete Component
public class SimpleCoffee implements Coffee {
@Override
public double getCost() {
return 1.0;
}
@Override
public String getIngredients() {
return "Coffee";
}
}
Step 3: Create the Decorator
public abstract class CoffeeDecorator implements Coffee {
protected final Coffee decoratedCoffee;
public CoffeeDecorator(Coffee decoratedCoffee) {
this.decoratedCoffee = decoratedCoffee;
}
public double getCost() {
return decoratedCoffee.getCost();
}
public String getIngredients() {
return decoratedCoffee.getIngredients();
}
}
Step 4: Create Concrete Decorators
public class Milk extends CoffeeDecorator {
public Milk(Coffee decoratedCoffee) {
super(decoratedCoffee);
}
@Override
public double getCost() {
return super.getCost() + 0.5;
}
@Override
public String getIngredients() {
return super.getIngredients() + ", Milk";
}
}
public class Sugar extends CoffeeDecorator {
public Sugar(Coffee decoratedCoffee) {
super(decoratedCoffee);
}
@Override
public double getCost() {
return super.getCost() + 0.2;
}
@Override
public String getIngredients() {
return super.getIngredients() + ", Sugar";
}
}
Step 5: Putting it all Together
public class Main {
public static void main(String[] args) {
Coffee simpleCoffee = new SimpleCoffee();
System.out.println("Cost: " + simpleCoffee.getCost() + ", Ingredients: " + simpleCoffee.getIngredients());
Coffee milkCoffee = new Milk(simpleCoffee);
System.out.println("Cost: " + milkCoffee.getCost() + ", Ingredients: " + milkCoffee.getIngredients());
Coffee sugarMilkCoffee = new Sugar(milkCoffee);
System.out.println("Cost: " + sugarMilkCoffee.getCost() + ", Ingredients: " + sugarMilkCoffee.getIngredients());
}
}
In the example above, we have successfully applied the Decorator pattern to the Coffee
interface by creating a base component SimpleCoffee
and multiple decorators (Milk
and Sugar
) to dynamically add behavior without altering the base component.
Benefits of Using the Decorator Pattern
The Decorator pattern offers several benefits, including:
- Flexibility: It allows for dynamic behavior modification at runtime, providing flexibility in adding or removing responsibilities to objects.
- Scalability: New functionality can be easily added to the application by creating new decorators.
- Open/Closed Principle: It follows the open/closed principle, as it allows for extending the behavior of individual objects without modifying the original class.
- Maintainability: It promotes a clean and maintainable codebase by separating concerns and allowing for easy code reusability.
Use Cases for the Decorator Pattern in Java EE
The Decorator pattern is commonly used in Java EE for scenarios such as:
- Extending Functionality: When you want to add new functionality to an object without subclassing.
- Runtime Configuration: When you need to configure or customize objects at runtime.
- GUI Components: In graphical user interface development, the Decorator pattern is used to add new behaviors to GUI components.
Final Thoughts
In conclusion, the Decorator pattern is a powerful tool in Java EE for adding behavior to objects dynamically. By following the principles of the Decorator pattern, developers can write more maintainable, scalable, and flexible code. Its ability to extend functionality at runtime, maintain the open/closed principle, and promote maintainability makes it a valuable pattern in software development.
In your Java EE projects, consider the Decorator pattern for scenarios where dynamic behavior modification is required without altering the original class. It's a fundamental pattern that can greatly enhance the design and flexibility of your applications.
To delve deeper into the Decorator pattern and its application in Java EE, check out the official Oracle documentation and explore real-world examples in the context of enterprise application development.
Happy decorating with Java EE!