Streamlining List Operations in Scala
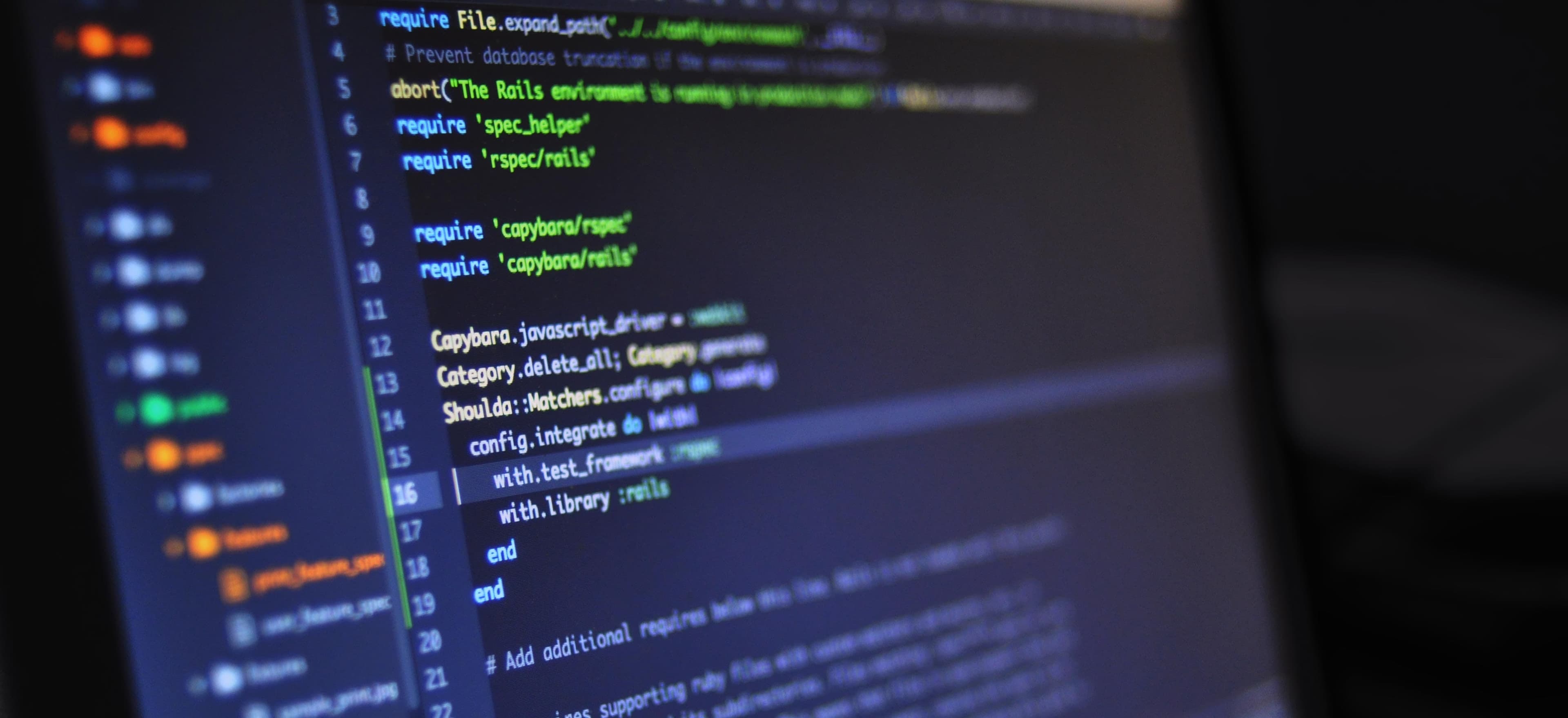
- Published on
Streamlining List Operations in Scala
When working with collections in Scala, the ability to perform operations in a concise yet efficient manner is crucial. Lists, being one of the most commonly used collection types in Scala, offer a wide range of operations that can be streamlined to enhance readability, maintainability, and performance.
In this post, we'll explore some advanced techniques to streamline list operations in Scala, leveraging powerful features such as higher-order functions, pattern matching, and immutability. We'll delve into examples that demonstrate the elegance and power of functional programming when working with lists in Scala.
Mapping Elements
One of the most versatile operations on lists is mapping. Mapping allows us to transform each element in a list based on a given function, producing a new list with the transformed elements.
Example 1: Applying a transformation function to each element of a list.
val numbers = List(1, 2, 3, 4, 5)
val squaredNumbers = numbers.map(n => n * n)
In this example, the map
operation applies the function n => n * n
to each element of the numbers
list, resulting in a new list squaredNumbers
containing the squares of the original elements.
Filtering Elements
Filtering a list involves selecting elements that match a given condition while discarding the rest. This is a fundamental operation when dealing with data manipulation and extraction.
Example 2: Filtering elements based on a condition.
val numbers = List(1, 2, 3, 4, 5)
val evenNumbers = numbers.filter(n => n % 2 == 0)
The filter
operation here retains only the elements that are even, creating a new list evenNumbers
containing [2, 4]
.
Reducing Elements
When we need to aggregate the elements of a list into a single value, the reduce
operation comes into play.
Example 3: Reducing a list of numbers to their sum.
val numbers = List(1, 2, 3, 4, 5)
val sum = numbers.reduce((acc, n) => acc + n)
Here, the reduce
operation accumulates the sum of all elements in the list, resulting in sum
being equal to 15
.
Chaining Operations
In many cases, we need to perform a series of operations on a list. Instead of using intermediate variables, we can chain these operations together, resulting in more concise and readable code.
Example 4: Chaining map
, filter
, and reduce
operations.
val numbers = List(1, 2, 3, 4, 5)
val result = numbers
.map(n => n * 2)
.filter(n => n > 5)
.reduce((acc, n) => acc + n)
In this example, we first double each element, then filter out those greater than 5, and finally sum the remaining elements. This results in a single value stored in result
.
Pattern Matching
Pattern matching in Scala allows for concise and powerful conditional expressions, making it particularly useful for working with lists.
Example 5: Pattern matching to handle different list scenarios.
val list = List(1, 2, 3, 4, 5)
list match {
case head :: tail => println(s"Head: $head, Tail: $tail")
case Nil => println("Empty list")
}
In this example, the match
expression checks whether the list is non-empty and binds the head and tail to variables, or if it's empty, it prints a message. This succinctly handles both cases.
Immutable Lists
Scala promotes immutable data structures, and lists are no exception. Immutable lists increase safety and concurrency by preventing unintended modifications, and Scala's collections library offers various operations to create new lists based on existing ones without modifying them.
Example 6: Creating a new list by appending an element to an existing list.
val list1 = List(1, 2, 3)
val list2 = list1 :+ 4
Here, list1 :+ 4
doesn't modify list1
; it produces a new list list2
with 4 appended to it. This ensures that list1
remains unchanged.
Final Thoughts
When working with lists in Scala, leveraging advanced techniques not only streamlines operations but also promotes functional programming principles, leading to more robust, maintainable, and expressive code. Through the examples provided, we've demonstrated the power and elegance of Scala's list operations, emphasizing their conciseness and clarity.
With a strong foundation in functional programming, pattern matching, and immutability, you can optimize your list operations in Scala while embracing a more expressive and safe coding style.
To further explore Scala and its capabilities with lists, refer to the Scala documentation.
Incorporating these techniques and practices into your Scala codebase will not only enhance your productivity but also pave the way for creating more resilient and efficient software solutions.