Troubleshooting Delays in Asynchronous Business Transactions
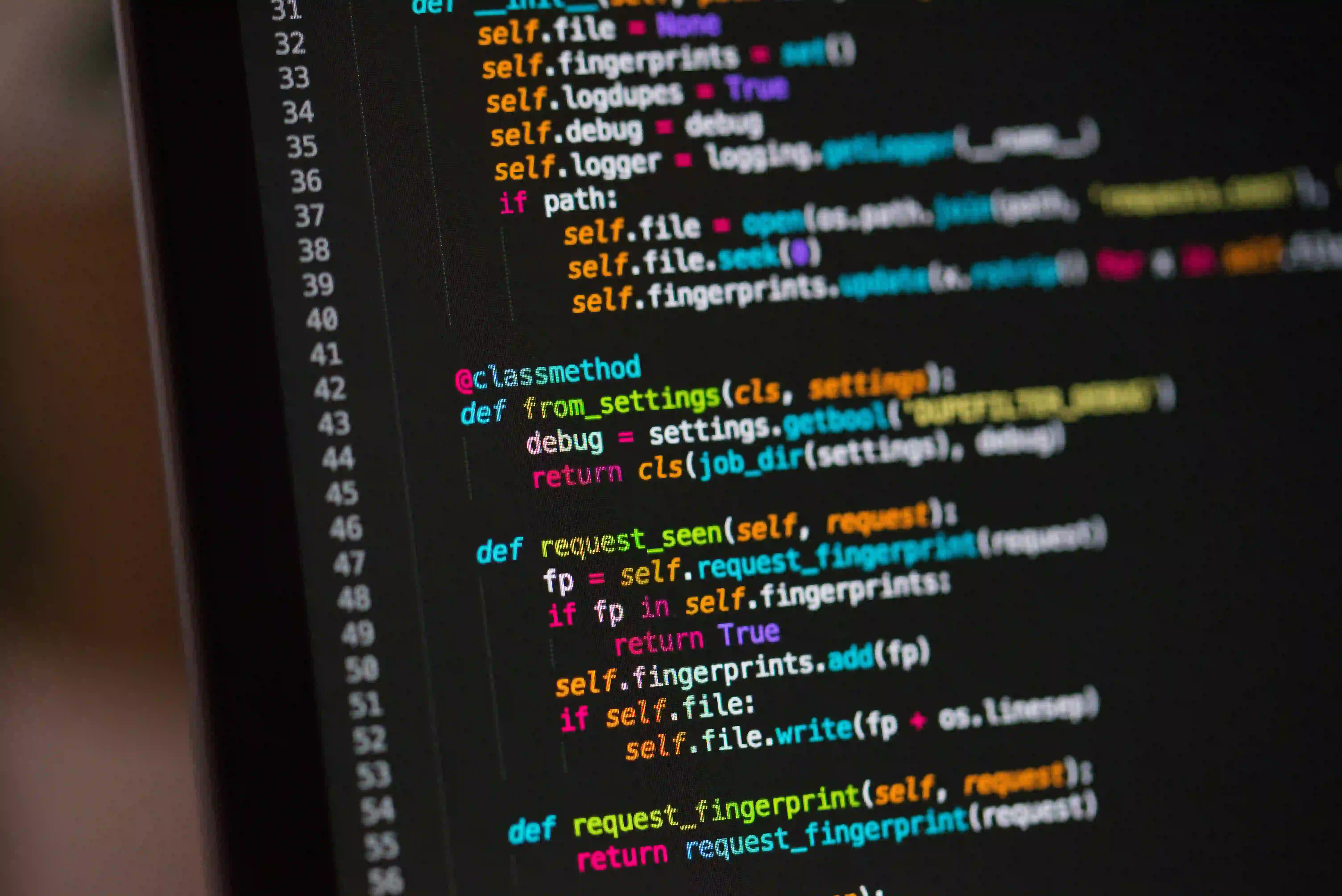
Understanding and Resolving Delays in Asynchronous Business Transactions
In many enterprise applications, asynchronous processing of business transactions is a common practice. This approach allows for improved system responsiveness and scalability as tasks are executed independently from the main flow of the application. However, asynchronous processing can introduce complexities, and delays may occur, leading to potential issues and challenges. In this blog post, we will explore the common reasons behind delays in asynchronous business transactions and discuss effective troubleshooting strategies using Java.
The Challenges of Asynchronous Business Transactions
Asynchronous processing involves breaking down tasks into smaller units of work, often executed in a separate thread or process. While this approach offers benefits such as improved throughput and responsiveness, it also introduces challenges related to coordination, error handling, and resource management. Delays in asynchronous business transactions can stem from various sources, including but not limited to:
-
Concurrency Issues: When multiple threads or processes interact with shared resources, contention and synchronization problems can lead to delays and performance degradation.
-
Network Latency: Asynchronous business transactions often involve communication over networks, and latency in network operations can contribute to delays in processing.
-
External Service Dependencies: Dependencies on external services or systems can introduce variability and unpredictability, impacting the overall execution time of asynchronous transactions.
Identifying Delayed Transactions
Before diving into troubleshooting, it's crucial to identify and categorize the delayed transactions. Monitoring tools, logging mechanisms, and performance profiling can help in pinpointing the transactions experiencing delays. When dealing with a Java-based application, tools like VisualVM, YourKit, or Java Flight Recorder can provide valuable insights into the behavior of asynchronous transactions.
Troubleshooting Strategies in Java
Once the delayed transactions are identified, it's time to apply targeted troubleshooting strategies. In the context of Java development, several techniques and best practices can be employed to address delays in asynchronous business transactions.
1. Thread Pool Management
Java provides a robust concurrency framework with classes like ExecutorService
and ThreadPoolExecutor
for managing thread pools. Review the configuration of thread pools to ensure an optimal number of threads, proper handling of task queues, and appropriate thread pool sizing based on the nature of asynchronous tasks. Here's an example of using an ExecutorService
with a fixed-size thread pool:
ExecutorService executor = Executors.newFixedThreadPool(10);
executor.execute(() -> {
// Perform asynchronous task
});
executor.shutdown();
Why: Inadequate thread pool configuration can lead to thread starvation or excessive context switching, causing delays in task execution. By optimizing the thread pool settings, the overall throughput of asynchronous transactions can be improved.
2. Asynchronous I/O and Non-Blocking Operations
When dealing with I/O-bound operations in asynchronous transactions, leveraging non-blocking I/O and asynchronous I/O capabilities provided by Java NIO can mitigate delays caused by waiting for I/O operations to complete. The java.nio
package offers channels, buffers, and selectors to enable efficient I/O operations. Consider the following example of asynchronous file I/O using Java NIO:
AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(Paths.get("file.txt"));
ByteBuffer buffer = ByteBuffer.allocate(1024);
fileChannel.read(buffer, 0, buffer, (result, attachment) -> {
// Process the read data asynchronously
});
Why: Traditional blocking I/O operations can introduce significant delays, especially in asynchronous scenarios. Utilizing non-blocking and asynchronous I/O capabilities can improve overall throughput and responsiveness in I/O-bound asynchronous transactions.
3. Reactive Programming with RxJava
Reactive programming has gained traction in the Java ecosystem, offering a paradigm for handling asynchronous and event-based scenarios. Libraries like RxJava provide abstractions for representing and composing asynchronous sequences of data or events. By leveraging reactive programming, you can express complex asynchronous workflows in a more declarative and composable manner. Consider a simple example using RxJava to handle asynchronous data processing:
Observable.fromCallable(() -> {
// Perform asynchronous task and return result
})
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(result -> {
// Handle the result asynchronously
}, error -> {
// Handle errors
});
Why: The reactive approach simplifies the handling of asynchronous operations and offers powerful operators for dealing with delays, errors, and concurrency, making it a valuable tool for troubleshooting and improving the performance of asynchronous business transactions in Java applications.
4. Circuit Breaker Pattern and Resilience4j
When dealing with external service dependencies in asynchronous transactions, resilience mechanisms become crucial. The Circuit Breaker pattern, along with libraries such as Resilience4j, can help in isolating and handling failures of external services, preventing cascading failures and mitigating delays caused by unreliable dependencies. Here's an example of integrating Resilience4j's Circuit Breaker in Java:
CircuitBreakerConfig config = CircuitBreakerConfig.custom()
.failureRateThreshold(20)
.waitDurationInOpenState(Duration.ofSeconds(30))
.build();
CircuitBreaker circuitBreaker = CircuitBreakerRegistry.of(config).circuitBreaker("myService");
Supplier<String> supplier = CircuitBreaker.decorateSupplier(circuitBreaker, () -> {
// Call the external service
return externalService.call();
});
String result = Try.ofSupplier(supplier)
.recover(throwable -> "Fallback value")
.get();
Why: By applying resilience patterns like the Circuit Breaker, you can proactively manage delays and failures caused by external service dependencies, improving the overall robustness of asynchronous transactions.
Key Takeaways
Delays in asynchronous business transactions can have detrimental effects on system performance, reliability, and user experience. By understanding the challenges, identifying delayed transactions, and employing targeted troubleshooting strategies in the context of Java development, you can mitigate delays and improve the efficiency of asynchronous processing. From thread pool management to reactive programming and resilience patterns, Java offers a rich set of tools and techniques to address and resolve delays in asynchronous business transactions, ensuring the smooth operation of enterprise applications.
To delve deeper into asynchronous processing in Java, check out the official Java Concurrency documentation and the Reactive Streams API provided by ReactiveX to enrich your understanding and mastery of asynchronous programming paradigms.