Understanding JSON Binding in Adapters
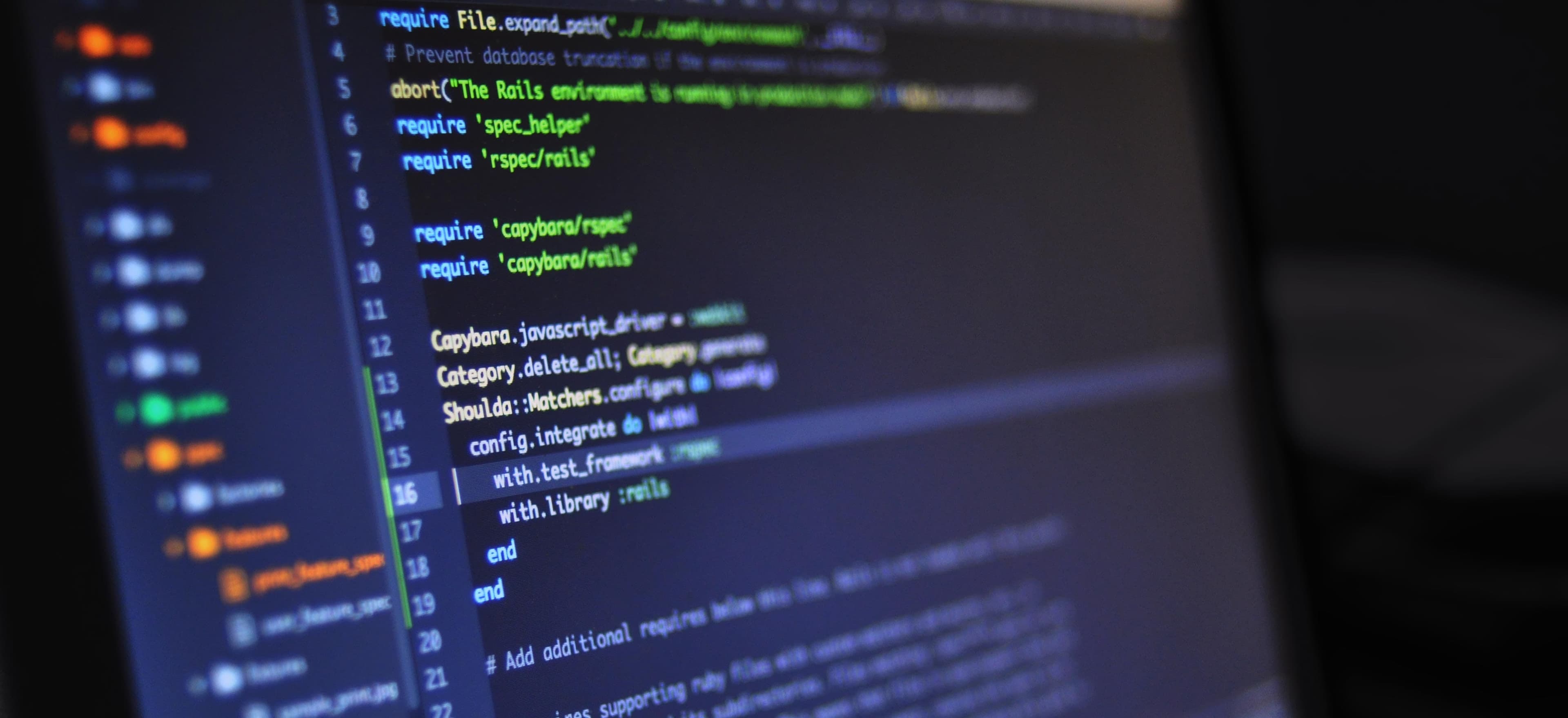
- Published on
JSON (JavaScript Object Notation) is a lightweight data interchange format that is widely used in web development. With the rise of RESTful web services and APIs, the ability to serialize and deserialize Java objects to and from JSON has become increasingly important. In Java, JSON binding provides a convenient way to work with JSON data, and adapters play a crucial role in customizing the serialization and deserialization process.
What Are Adapters in JSON Binding?
Adapters in JSON binding (such as the ones provided by the javax.json.bind.adapter
package) allow developers to customize how Java objects are converted to JSON and vice versa. They provide a way to handle special cases where the default serialization/deserialization behavior does not meet the specific requirements of an application.
Why Use Adapters?
Adapters are essential because they allow you to handle scenarios where the default serialization and deserialization processes may not be sufficient. For example, you may need to transform data from one form to another, or you may need to handle complex mappings between Java objects and JSON representations.
Creating a Simple Adapter
Let's start by creating a simple adapter that converts a LocalDate
object to and from a JSON string. Suppose you have a Java class Person
with a LocalDate
field representing the person's birthdate.
First, define the adapter class implementing JsonbAdapter
:
import java.time.LocalDate;
import javax.json.bind.adapter.JsonbAdapter;
public class LocalDateAdapter implements JsonbAdapter<LocalDate, String> {
@Override
public String adaptToJson(LocalDate date) throws Exception {
return date.toString(); // Serialize LocalDate to String
}
@Override
public LocalDate adaptFromJson(String date) throws Exception {
return LocalDate.parse(date); // Deserialize String to LocalDate
}
}
In this example, the LocalDateAdapter
class implements the JsonbAdapter
interface and provides custom logic for serializing LocalDate
objects to strings and deserializing strings back to LocalDate
objects. This allows you to control how LocalDate
objects are represented in the JSON output.
Using the Adapter in JSON Binding
To use the adapter, you need to annotate the LocalDate
field in the Person
class with @JsonbTypeAdapter
:
import java.time.LocalDate;
import javax.json.bind.annotation.JsonbTypeAdapter;
public class Person {
private String name;
@JsonbTypeAdapter(LocalDateAdapter.class)
private LocalDate birthdate;
// Constructor, getters, setters
}
Here, the @JsonbTypeAdapter
annotation specifies the adapter class to be used for serializing and deserializing the birthdate
field in the Person
class.
When to Use Adapters
Adapters are particularly useful when working with third-party APIs, legacy systems, or when dealing with complex data transformations. They allow you to seamlessly integrate custom serialization and deserialization logic without modifying the existing domain model.
For example, imagine you need to work with an external API that expects dates in a specific format different from the standard ISO 8601 format. Adapting the date serialization logic using a custom adapter allows you to meet the requirements of the external API without affecting the internal representation of dates in your application.
The Bottom Line
In conclusion, adapters in JSON binding provide a powerful mechanism to customize the serialization and deserialization of Java objects to and from JSON. By creating custom adapters, you can tailor the JSON representation of your Java objects to suit specific requirements, making your application more flexible and adaptable to various data formats and APIs.
Adapters are a fundamental part of the JSON binding process, and mastering their usage is essential for developing robust and flexible JSON processing solutions in Java. Utilize adapters to address specialized data mapping needs, ensuring smooth integration with external systems and seamless data manipulation within your applications.
For further insights into JSON binding and adapters, you can refer to the official documentation of the Java API for JSON Processing (JSON-P) and the Java Architecture for XML Binding (JAXB). These resources offer comprehensive guidance on working with JSON and adapters in the Java ecosystem.