Understanding Kotlin's Reified Type Parameters
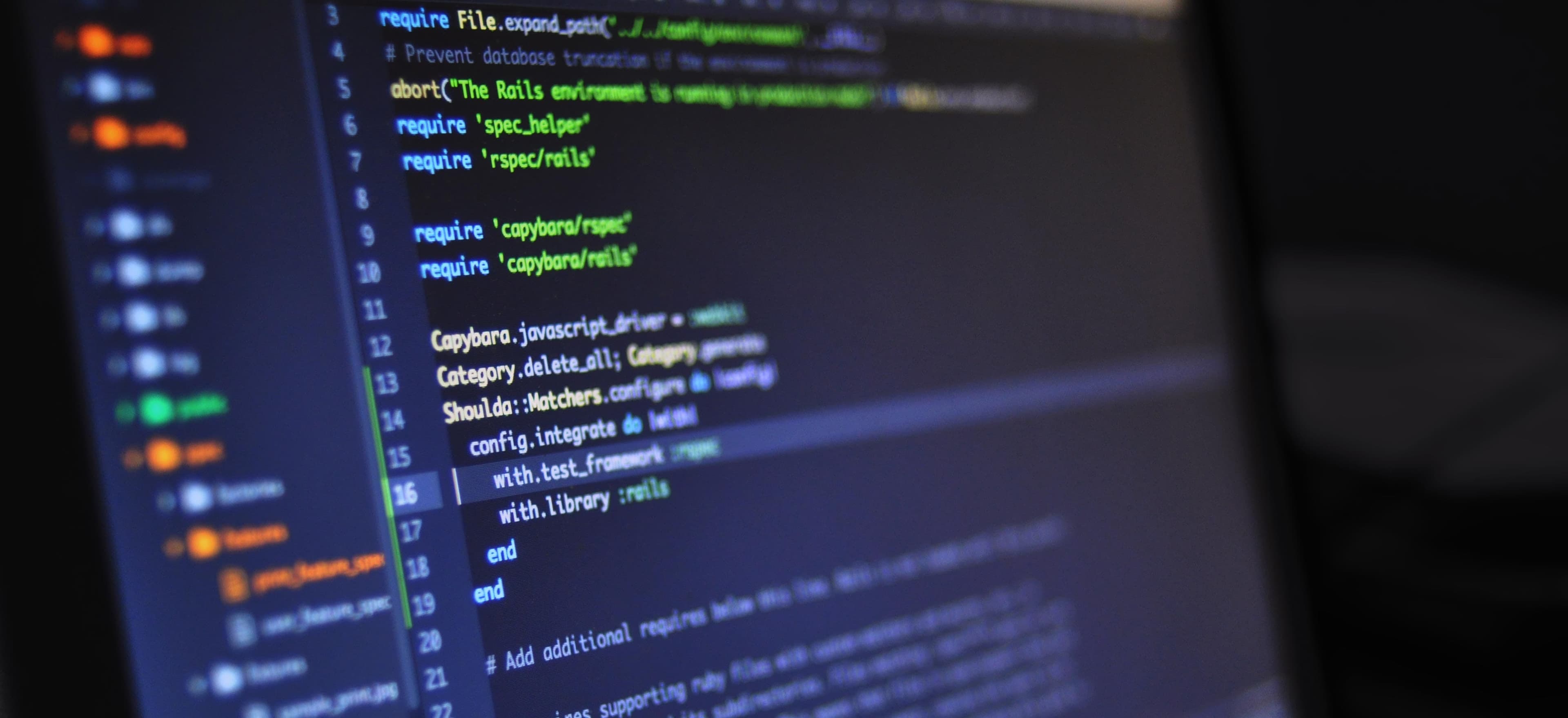
- Published on
Understanding Kotlin's Reified Type Parameters
Kotlin's reified type parameters are a powerful feature that allows developers to work with concrete types within inline functions, addressing the limitations of Java's generic types. This allows for more flexibility and type-safe operations at runtime. In this blog post, we'll explore reified type parameters in Kotlin, understand their significance, and learn how to utilize them effectively.
What are Type Parameters?
In Kotlin, type parameters enable us to create generic classes, interfaces, and functions. It allows us to write code that can work with a variety of data types in a type-safe manner. However, one limitation of type parameters in Kotlin is the inability to access the actual class of a type parameter at runtime due to type erasure, similar to Java's generics.
The Limitations of Type Erasure
In Java, generic types suffer from type erasure, which means that the information about actual types used in the generic class is not available at runtime. This limitation restricts the ability to perform certain operations like checking the type of a generic parameter at runtime, leading to the need for explicit casting and potential runtime errors.
Enter Reified Type Parameters
Kotlin introduces the reified
modifier to address the limitations imposed by type erasure. When a type parameter is marked as reified
, it allows us to access the actual type at runtime within inline functions. This effectively provides the ability to perform type checks, type casts, and create instances of the type parameter at runtime.
By using reified
type parameters, we can write more concise and type-safe code within inline functions, eliminating the need for explicit casts and improving the overall reliability of our code.
How to Use Reified Type Parameters
To use reified type parameters, the function where the type parameter is declared must be marked as inline
. This instructs the compiler to inline the function's bytecode at the call site, allowing the reified type information to be captured and utilized at runtime.
Let's take a look at an example of a generic function that utilizes reified
type parameters:
inline fun <reified T> printIfInstance(value: Any) {
if (value is T) {
println(value)
}
}
In this example, the function printIfInstance
is marked as inline
and uses a reified
type parameter T
. Within the function, we perform a type check using the is
operator, which is only possible due to the reified
modifier.
Now, let's see how we can call the printIfInstance
function:
printIfInstance<String>("Hello, World!") // Output: Hello, World!
printIfInstance<Int>("Hello, World!") // No output
In the first invocation, the printIfInstance
function successfully prints the string "Hello, World!" because the type T
is reified and the type check value is T
can be performed at runtime. In the second invocation, no output is produced because the type check fails.
Benefits of Reified Type Parameters
The use of reified type parameters brings several benefits to Kotlin developers:
-
Type-safe Operations: Reified type parameters enable type-safe operations within inline functions, eliminating the need for explicit casts and reducing the likelihood of runtime errors.
-
Improved Code Readability: By leveraging reified type parameters, the code becomes more concise and readable as the type information is available at runtime, simplifying conditional logic and type-based operations.
-
Enhanced API Design: APIs utilizing reified type parameters can offer more intuitive and streamlined interfaces, as the type information can be leveraged directly within the function body.
Limitations and Considerations
While reified type parameters provide significant advantages, there are some limitations and considerations to keep in mind:
-
Only for Inline Functions: Reified type parameters can only be used within inline functions, which restricts their applicability to certain contexts.
-
Memory Overhead: Using reified type parameters within inline functions may lead to increased memory overhead, as the bytecode of the inline function is duplicated at each call site.
-
Reflective Operations: While reified type parameters allow for type checks and casts, they do not support general reflective operations, such as obtaining class references or working with reflection APIs.
Final Considerations
In conclusion, Kotlin's reified type parameters offer a powerful solution to the limitations imposed by type erasure, enabling developers to work with concrete types within inline functions and perform type-safe operations at runtime. By leveraging reified type parameters, developers can write more robust and readable code, enhancing the overall reliability and maintainability of their Kotlin applications.
Understanding the significance and usage of reified type parameters is essential for developers looking to harness the full potential of Kotlin's type system and build robust, type-safe applications.
For further exploration, consider reviewing the official Kotlin documentation on reified type parameters to deepen your understanding and discover advanced use cases.
Incorporate reified type parameters into your Kotlin projects to elevate your code quality and leverage the full potential of Kotlin's flexible and expressive type system.