Importing External Data Models for JBoss BPM Suite: Common Challenges
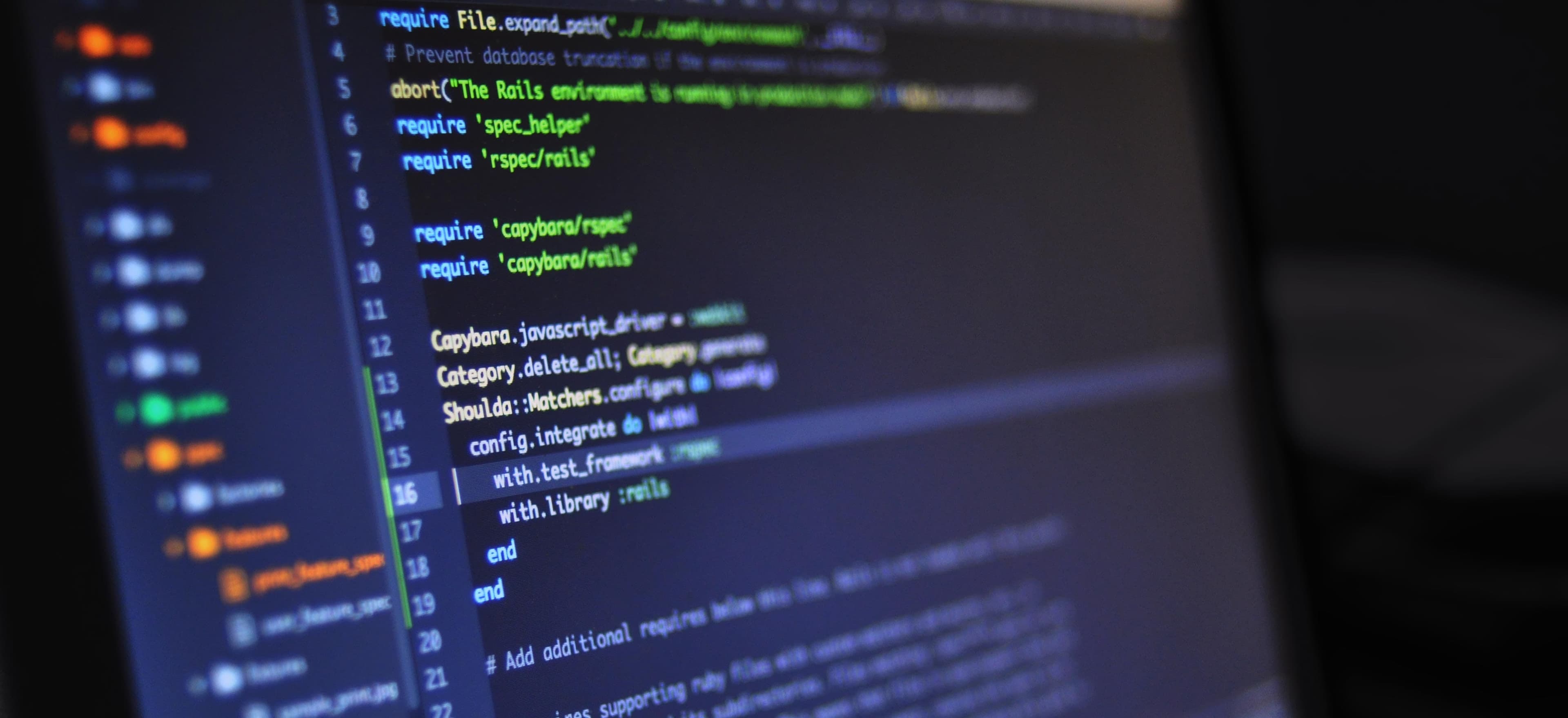
- Published on
Importing External Data Models for JBoss BPM Suite: Common Challenges
In the world of enterprise application development, the ability to import external data models into a Business Process Management (BPM) suite is crucial for building robust and dynamic business processes. JBoss BPM Suite is a powerful tool that allows developers to model, execute, and monitor business processes. However, importing external data models into JBoss BPM Suite can present various challenges. In this article, we'll explore some of the common challenges faced when importing external data models into JBoss BPM Suite and discuss strategies to overcome them.
Understanding External Data Models
External data models are representations of the data structures used by an organization, often defined and maintained in separate systems such as databases, enterprise resource planning (ERP) systems, or other business applications. Importing these external data models into JBoss BPM Suite allows business processes to interact with and manipulate this data, enabling seamless integration between processes and the organization's data ecosystem.
Challenge 1: Data Model Mismatch
One of the primary challenges when importing external data models into JBoss BPM Suite is dealing with potential mismatches between the data models used in the BPM suite and those used in the external systems. This misalignment can manifest in differences in data types, structures, and naming conventions, making it difficult to seamlessly integrate the external data models with the BPM processes.
Strategy: Data Model Transformation
To address data model mismatches, developers can employ data model transformation techniques. This involves mapping the attributes and fields of the external data models to the corresponding elements in the BPM suite's data model. Additionally, tools such as JBoss Data Virtualization can be utilized to create virtual data layers that abstract the underlying data model differences, providing a unified view for the BPM processes while preserving the integrity of the external data.
Example:
// Example of mapping external data model to BPM data model
public class DataTransformer {
public BPMData transformExternalData(ExternalData externalData) {
BPMData bpmData = new BPMData();
bpmData.setField1(externalData.getAttributeA());
bpmData.setField2(externalData.getAttributeB());
// Additional mapping logic
return bpmData;
}
}
In this example, the DataTransformer
class demonstrates the mapping of external data to the BPM data model, showcasing the transformation process to align the external data with the BPM suite's requirements.
Challenge 2: Data Consistency and Synchronization
Another common challenge is ensuring data consistency and synchronization between the BPM suite and the external data models. As business processes interact with external data, it is critical to maintain the integrity and coherence of the shared data to avoid discrepancies and errors.
Strategy: Synchronization Mechanisms
Developers can implement robust synchronization mechanisms to ensure data consistency between the BPM suite and external systems. This involves leveraging technologies like Java Message Service (JMS) to establish asynchronous communication and data replication strategies to propagate changes across the systems in a coordinated manner.
Example:
// Example of using JMS for data synchronization
public class DataSynchronizer {
private JMSContext jmsContext;
public void synchronizeData(BPMData bpmData) {
// Convert BPM data to message
Message message = jmsContext.createTextMessage(bpmData.toString());
// Send message to external data system queue
jmsContext.createProducer().send(externalDataQueue, message);
}
}
In this example, the DataSynchronizer
class utilizes JMS to synchronize BPM data with the external data system, demonstrating the implementation of a synchronization mechanism to ensure data consistency.
Challenge 3: Versioning and Evolution
The evolution of external data models over time presents another challenge, as changes in the structure and schema of external data can impact the existing BPM processes and integrations. Versioning and managing these changes in a controlled manner is essential to prevent disruptions to the BPM workflows.
Strategy: Semantic Versioning and Adapters
Applying semantic versioning to external data models and implementing adapters can mitigate the impact of data model evolution on BPM processes. By maintaining backward compatibility and providing adapters to handle different versions of the data models, developers can ensure that BPM processes remain resilient to changes in the external data structures.
Example:
// Example of using adapters for versioned data models
public interface DataModelAdapter {
void processVersionedData(V1Data v1Data);
// Additional methods for handling other versions
}
public class V2DataModelAdapter implements DataModelAdapter {
@Override
public void processVersionedData(V1Data v1Data) {
// Adapt V1 data to V2 data
V2Data v2Data = adaptV1ToV2(v1Data);
// Process V2 data
// ...
}
}
In this example, the DataModelAdapter
interface and V2DataModelAdapter
class illustrate the use of adapters to process different versions of the external data models, enabling seamless integration with evolving data structures.
Final Thoughts
Importing external data models into JBoss BPM Suite is a critical aspect of building robust and integrated business processes. By understanding and addressing common challenges such as data model mismatch, data consistency and synchronization, and versioning and evolution, developers can enhance the interoperability and resilience of BPM processes with external data systems.
In conclusion, while importing external data models presents challenges, employing strategies such as data model transformation, synchronization mechanisms, semantic versioning, and adapters can empower developers to effectively integrate external data with JBoss BPM Suite, unlocking the full potential of BPM-driven business process automation.
By overcoming these challenges, developers can harness the full power of JBoss BPM Suite to orchestrate and automate business processes seamlessly, resulting in increased efficiency and agility within the organization.
For a deeper understanding of BPM and data integration, check out Red Hat's guide to business process management and JBoss BPM Suite documentation.