Inconsistent Widening Operation Rules in Java
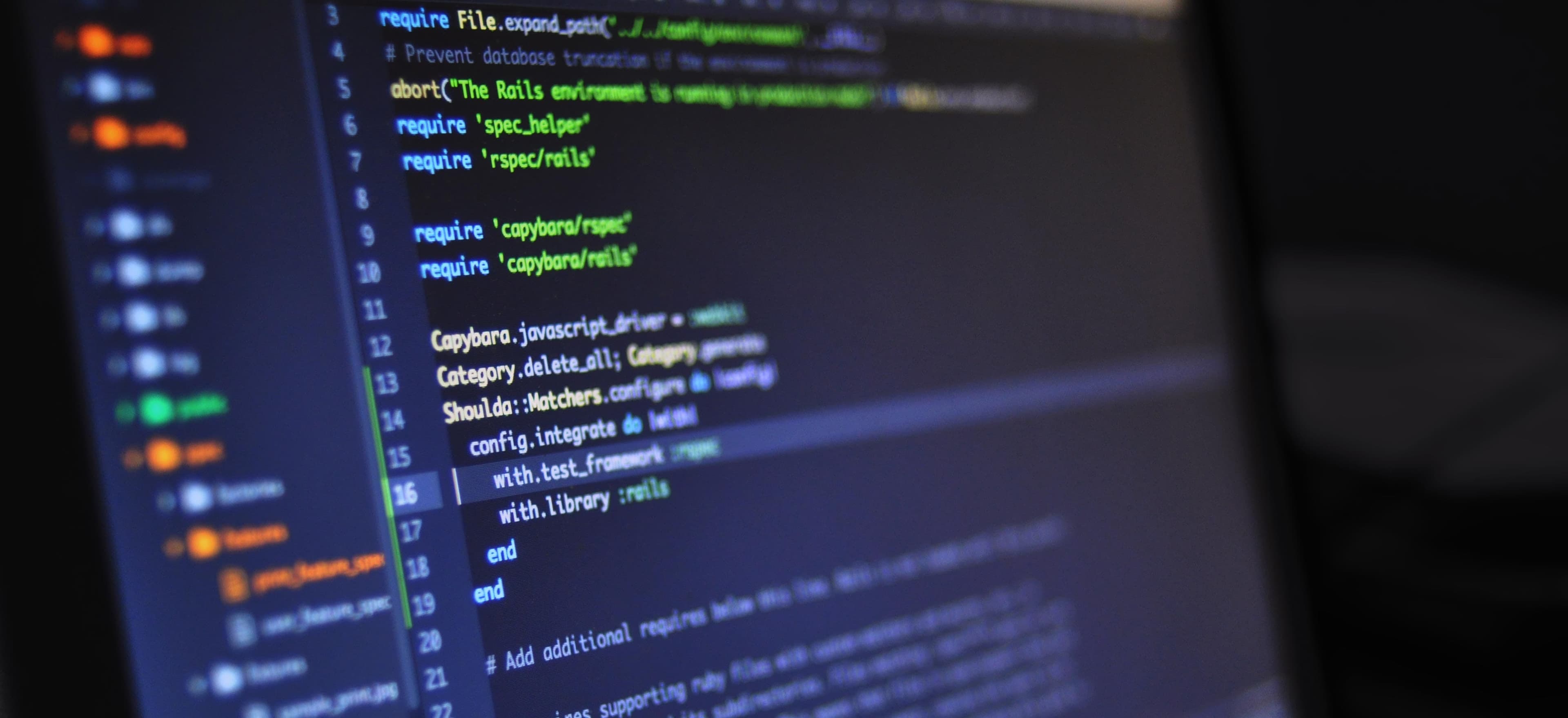
- Published on
Inconsistent Widening Operation Rules in Java
When working with numeric data types in Java, developers often encounter the concept of widening operations. Widening refers to converting a value from one data type to a larger data type, such as converting an integer to a double. While this process generally follows a set of rules, there are instances where the behavior can be inconsistent, leading to potential pitfalls and unexpected results. In this article, we will delve into the world of widening operations in Java, explore the rules that govern them, and uncover scenarios where these rules may not align with expectations.
Understanding Widening in Java
Widening, also known as implicit type conversion, occurs when a value of a smaller data type is automatically promoted to a larger data type. This process is built into the language to facilitate operations involving different numeric types without requiring explicit casting. For example, converting an int
to a long
or a float
to a double
are common widening operations in Java.
The Rules of Widening
In Java, the rules for widening operations are well-defined and generally straightforward:
- Smaller to Larger: Java allows automatic widening from a smaller data type to a larger data type. For example, converting a
byte
to anint
or ashort
to adouble
is permissible. - Integral Promotion: When performing arithmetic operations, smaller integral types (byte, short, char) are first promoted to
int
before further evaluation. This rule aims to avoid unexpected results and loss of precision in calculations. - Floating-Point Widening: Widening is also applicable between floating-point types, where a
float
can be automatically widened to adouble
.
Following these rules ensures predictable behavior and correct outcomes when working with different numeric types in Java.
Inconsistencies in Widening Operations
While Java's widening rules are generally reliable, there are circumstances where inconsistencies arise, leading to potential confusion and programming errors. Let's explore a few scenarios where Java's widening operations may behave unexpectedly.
Widening vs. Overloading
In Java, widening conversions can clash with method overloading, especially when working with wrapper classes. Consider the following example:
public class WideningExample {
public void display(double num) {
System.out.println("Double: " + num);
}
public void display(float num) {
System.out.println("Float: " + num);
}
public static void main(String[] args) {
WideningExample example = new WideningExample();
example.display(10);
}
}
In this example, the method display(double num)
is expecting a double
, while display(float num)
is expecting a float
. However, when invoking example.display(10)
, which method will be called? This is where widening comes into play. Java widens the integer literal 10
to a double
, resulting in a call to display(double num)
. This behavior might not align with the programmer's intention and could lead to unexpected method invocation.
Widening with Ambiguous Types
Another area of inconsistency arises when dealing with mixed integral and floating-point operations. Consider the following code snippet:
public class WideningAmbiguity {
public static void main(String[] args) {
int x = 5;
float y = 2.5f;
System.out.println("Result: " + (x * y));
}
}
The expected outcome of multiplying an int
and a float
is a float
value. However, Java's widening operation rules dictate that the int
will be implicitly widened to a float
before the multiplication, resulting in a float
value. While this behavior is consistent with widening, it may not align with the programmer's mental model of the operation.
Widening with Constants
Java's treatment of numerical constants during widening operations can also lead to unexpected results. Consider the following scenario:
public class WideningConstants {
public static void main(String[] args) {
byte b1 = 5;
byte b2 = 10;
byte b3 = b1 + b2; // Error: incompatible types: possible lossy conversion from int to byte
}
}
In this example, adding two byte
variables b1
and b2
should result in a byte
value. However, Java widens the result of the addition to an int
before attempting to store it in b3
, resulting in a compilation error. While the integral promotion rule is being followed, it can lead to confusion when working with constants and smaller data types.
Mitigating Inconsistencies
To address the potential pitfalls and inconsistencies associated with widening operations in Java, developers can adopt several strategies:
-
Explicit Casting: Although widening is automatic in Java, explicit casting can be employed to clarify the intended data type conversion. This approach can enhance code readability and prevent unexpected behaviors.
-
Awareness and Documentation: Understanding the nuances of widening operations and documenting the potential inconsistencies within the codebase can help mitigate unexpected results and aid in maintaining code clarity for future developers.
-
Testing and Validation: Performing thorough testing, especially when dealing with mixed numeric types and widening, can unveil potential issues and lead to code refinements that align with anticipated outcomes.
Wrapping Up
Java's widening operations play a crucial role in handling numeric data types, facilitating seamless interactions between different primitive types. While the rules governing widening are generally predictable, there exist instances of inconsistency that can lead to programming pitfalls. By navigating the intricacies of widening operations, employing explicit casting when necessary, and promoting awareness of potential inconsistencies, developers can enhance the reliability and clarity of their Java codebases.
Understanding the rules and potential inconsistencies associated with widening operations empowers developers to write more robust and predictable Java code. By addressing these anomalies head-on and leveraging best practices, developers can optimize the use of widening operations and minimize the risk of unexpected behaviors, ensuring the stability and comprehensibility of their Java applications.
Learning about the rules and inconsistencies of widening operations in Java can greatly enhance a developer's ability to produce reliable and efficient code. By accounting for these nuances, developers can ensure that their Java programs not only perform as intended but also maintain clear and understandable logic throughout their lifecycle.