Troubleshoot Common JSF Validation Errors
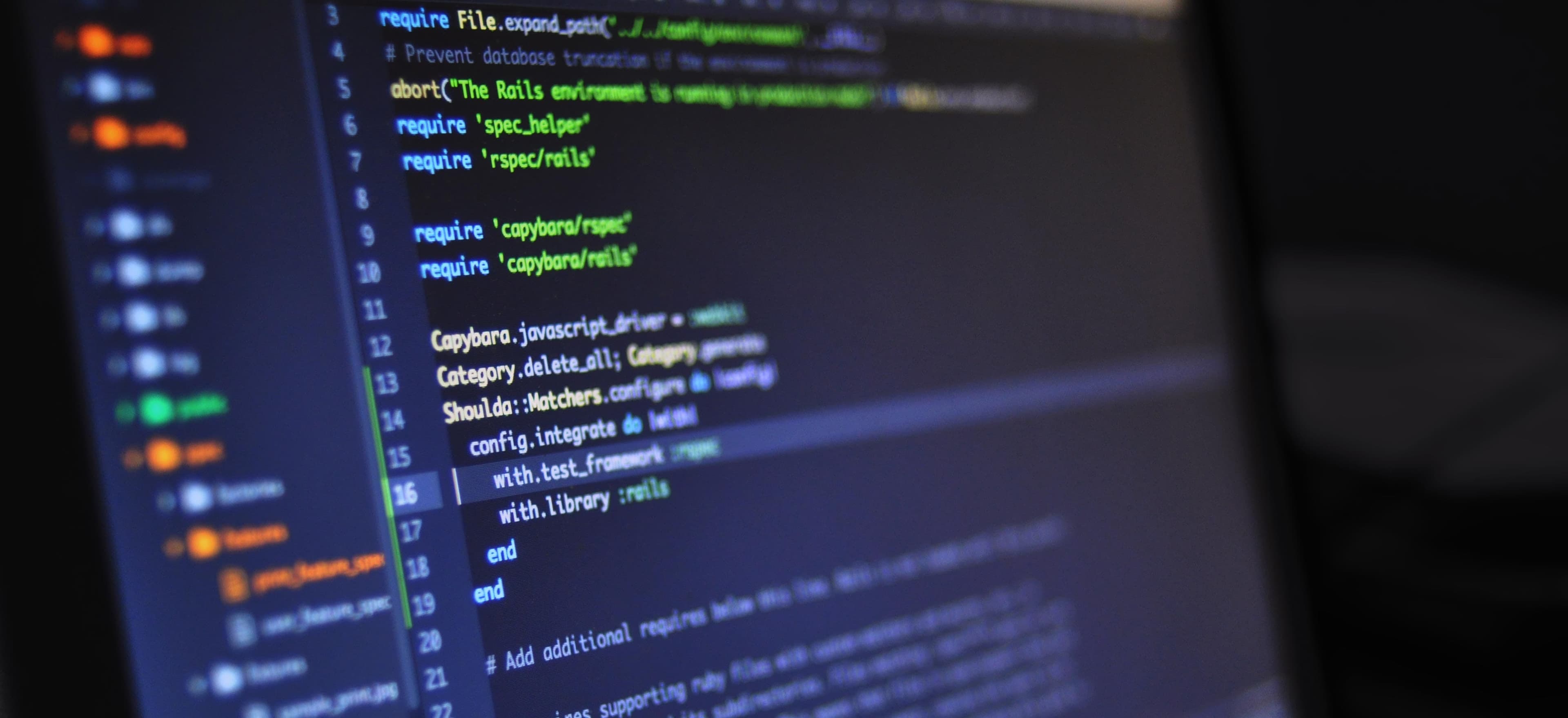
- Published on
Troubleshoot Common JSF Validation Errors
JavaServer Faces (JSF) is a Java specification for building component-based user interfaces for web applications. JSF provides a robust framework for handling form validation, ensuring that data entered by users meets specific criteria. However, during development, you may encounter common validation errors that can be frustrating to debug. In this post, we'll explore some of these common JSF validation errors and discuss how to troubleshoot and resolve them.
Understanding JSF Validation
In JSF, validation is an essential part of the application flow. It ensures that the data entered by the user is in the correct format and meets certain criteria before processing it further. Validation can be performed using built-in validators provided by JSF or by creating custom validators tailored to specific requirements.
Common JSF Validation Errors
1. Required Field Validation
One of the most common validation errors in JSF is related to required field validation. This error occurs when the required fields are not properly marked or validated.
<h:inputText id="username" value="#{userBean.username}" required="true" />
<h:message for="username" />
In this code snippet, the h:inputText
component has the required
attribute set to true
, indicating that the username
field is mandatory. The h:message
component is used to display any validation error messages associated with the username
field.
2. Input Format Validation
Another common validation error is related to input format validation. This error occurs when the user input does not match the specified format, such as date, email, or number formats.
<h:inputText id="email" value="#{userBean.email}">
<f:validateRegex pattern="^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$" />
</h:inputText>
<h:message for="email" />
In this example, the h:inputText
component is validated using the f:validateRegex
tag, which ensures that the entered email address matches the specified pattern.
3. Custom Validation Error Messages
Sometimes, customizing validation error messages can lead to errors if not done correctly. It's crucial to ensure that the error messages are associated with the correct input fields and are being displayed appropriately.
<h:message for="customField" />
<h:inputText id="customField" value="#{userBean.customField}">
<f:validateLength minimum="5" maximum="10" />
</h:inputText>
In this snippet, the f:validateLength
tag is used to specify the minimum and maximum length of the customField
input. The associated h:message
tag displays any validation error messages related to the customField
input.
Troubleshooting Common Validation Errors
When encountering validation errors in JSF, it's essential to follow a systematic approach to troubleshoot and resolve them effectively.
1. Check Component IDs and Associated Messages
Ensure that the for
attribute of the h:message
component matches the id
attribute of the input component it's associated with. This association is vital for displaying the correct error messages next to the corresponding input fields.
2. Review Validation Logic
Double-check the validation logic implemented for each input field. Ensure that the validation criteria, such as required fields, input format, or custom validation rules, are correctly defined and implemented.
3. Use Unified EL for Validation Logic
When referencing managed bean properties for validation, make sure to use Unified Expression Language (EL) syntax correctly. Incorrect EL references can lead to validation errors or unexpected behavior.
4. Leverage Built-in Validators
JSF provides a wide range of built-in validators that cover most common validation scenarios. Utilize these validators whenever possible to ensure consistent and reliable validation logic.
5. Test With Diverse Input Data
Test the validation logic with various input data to cover different scenarios. This helps identify any edge cases or specific input patterns that may trigger validation errors.
Key Takeaways
In conclusion, understanding and troubleshooting common JSF validation errors is essential for building robust and user-friendly web applications. By grasping the nuances of JSF validation and following a systematic approach to troubleshooting, developers can ensure smooth and reliable validation logic within their applications.
Remember, thorough testing and validation are crucial for delivering a seamless user experience in web applications built with JSF. By addressing common validation errors effectively, developers can create intuitive and error-free input forms, enhancing the overall quality of their JSF-based applications.
If you're interested in further expanding your knowledge of JSF validation and error handling, check out the official JavaServer Faces documentation on validation. Additionally, exploring real-world examples and best practices can provide valuable insights into handling complex validation scenarios in JSF applications.
Happy coding!
Checkout our other articles