"Maximizing Efficiency with Java 8 Streams API"
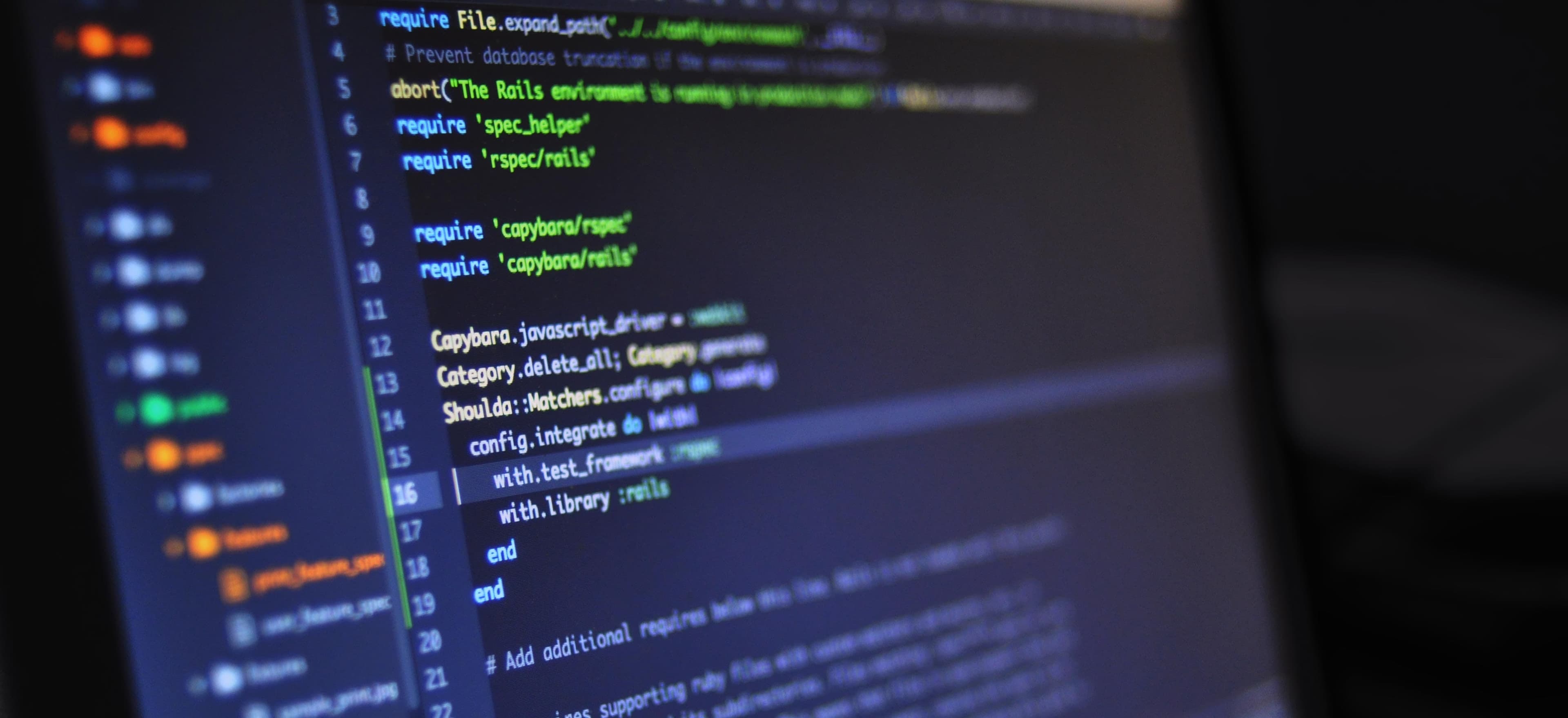
- Published on
Maximizing Efficiency with Java 8 Streams API
In the world of Java programming, efficiency is key. With the release of Java 8, a powerful new tool was introduced to make code more concise, readable, and efficient: the Streams API. Designed to work seamlessly with lambda expressions, the Streams API allows for functional-style operations on streams of elements, providing a clear and effective way to perform data processing.
What are Java 8 Streams?
Java 8 Streams represent a sequence of elements that support sequential and parallel aggregate operations. They are designed to provide a clean and concise way to work with collections and aggregate data, enabling developers to write more expressive and efficient code. Streams are particularly powerful for processing large data sets without the need for explicit iteration, making it easier to write parallelizable code.
Advantages of Using Java 8 Streams
Concise and Readable Code
One of the primary advantages of using Java 8 Streams is the ability to write concise and readable code. With the use of lambda expressions and method references, stream operations can be written in a declarative style, making the code easier to understand and maintain.
Parallelism
Streams API supports parallel execution, allowing developers to take advantage of multi-core architectures without having to write complex multithreading code. This can lead to significant performance improvements when working with large datasets.
Reusability
Since streams are built to be chained together, the same stream can be used for multiple operations, promoting reusability and reducing code duplication.
Declarative Style
By using stream operations, developers can focus on what needs to be done rather than how it should be done, promoting a more declarative style of programming.
Common Stream Operations
Filtering
The filter
operation is used to select elements based on a given predicate. This allows for the creation of a new stream containing only elements that satisfy the specified condition.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Filter names that start with 'A'
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
In the above example, the filter
operation is used to create a new stream containing names that start with the letter 'A'.
Mapping
The map
operation is used to transform each element in a stream using a given function. This is useful for converting or extracting specific properties from the elements.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Convert names to uppercase
List<String> uppercaseNames = names.stream()
.map(String::toUpperCase) // Method reference
.collect(Collectors.toList());
Here, the map
operation is used to convert all names to uppercase using a method reference.
Reduction
The reduce
operation allows for the combination of all elements in a stream into a single result. This can be used to calculate a sum, find the maximum value, or perform any other form of aggregation.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Calculate the sum of all numbers
int sum = numbers.stream()
.reduce(0, Integer::sum);
In this example, the reduce
operation is used to calculate the sum of all numbers in the stream, starting from an initial value of 0.
Using Streams for Enhanced Efficiency
By leveraging the power of Java 8 Streams, developers can significantly enhance the efficiency of their code. Let's consider a practical example to demonstrate the benefits of using Streams for data processing.
Scenario:
Suppose we have a list of employee objects, and we need to calculate the total salary expense for all employees.
Traditional Approach:
In the traditional approach, we would iterate through the list of employees and sum up their salaries.
List<Employee> employees = Arrays.asList(
new Employee("Alice", 50000),
new Employee("Bob", 60000),
new Employee("Charlie", 75000),
new Employee("David", 40000)
);
int totalSalary = 0;
for (Employee emp : employees) {
totalSalary += emp.getSalary();
}
While this approach gets the job done, it's not very expressive and may not be suitable for parallel processing large datasets.
Stream Approach:
Using Java 8 Streams, we can achieve the same result with a more concise and efficient code.
List<Employee> employees = Arrays.asList(
new Employee("Alice", 50000),
new Employee("Bob", 60000),
new Employee("Charlie", 75000),
new Employee("David", 40000)
);
int totalSalary = employees.stream()
.mapToInt(Employee::getSalary)
.sum();
In this streamlined approach, we use the mapToInt
operation to extract the salaries as integers and then use sum
to calculate the total salary expense. This code is more expressive, easier to understand, and can be effortlessly parallelized for better performance.
In Conclusion, Here is What Matters
Java 8 Streams API empowers developers to write clean, expressive, and efficient code for data processing. By leveraging the functional-style operations provided by streams, developers can maximize code reusability, embrace a declarative programming style, and harness the power of parallel execution for enhanced performance.
In summary, Java 8 Streams API is a valuable tool for enhancing code efficiency, and its adoption can lead to more maintainable, scalable, and performant applications.
Now that you have a better understanding of Java 8 Streams API, take the opportunity to explore more about Java 8 Streams and level up your coding skills!