Troubleshooting "NoClassDefFoundError" for SLF4J LoggerFactory
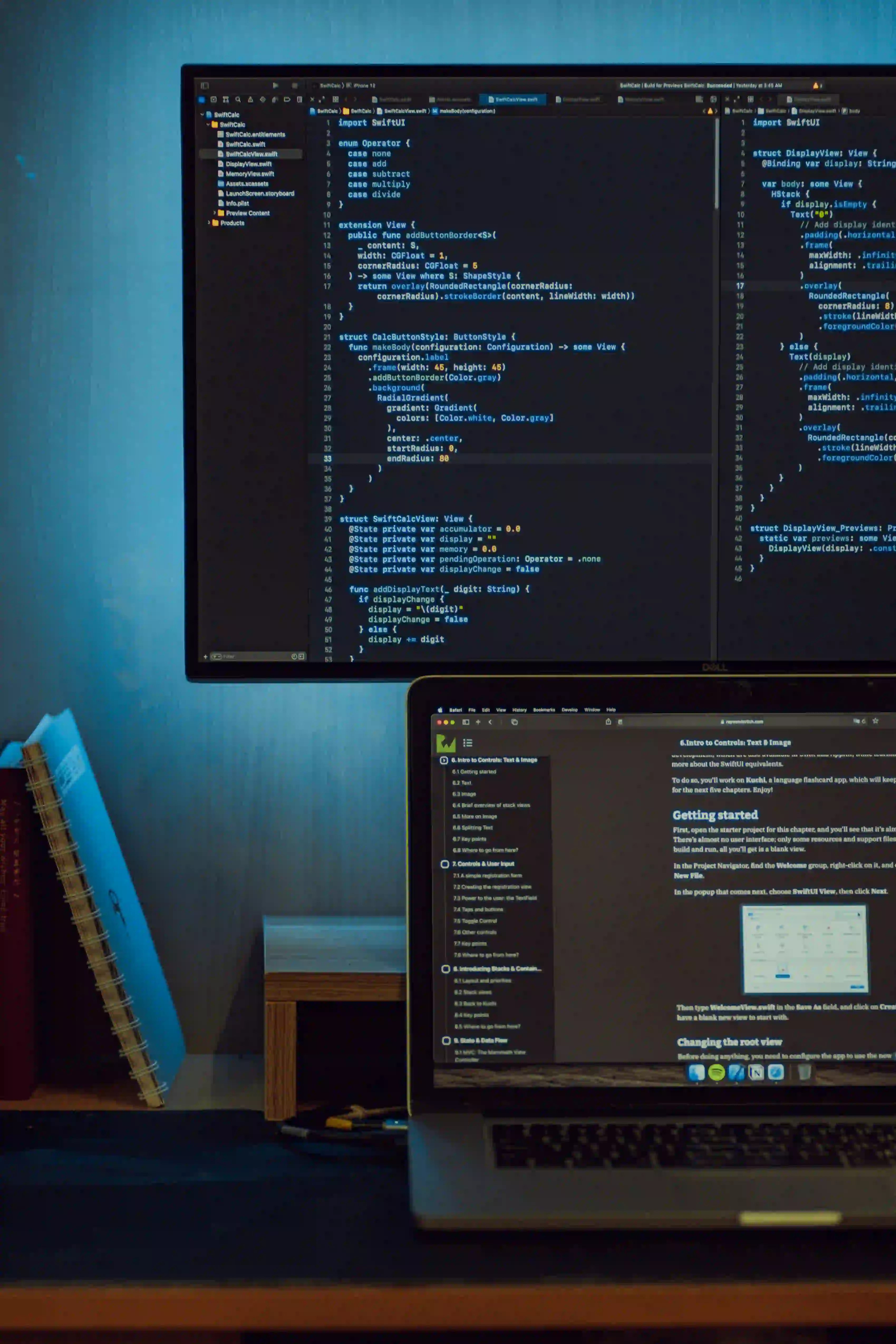
Troubleshooting "NoClassDefFoundError" for SLF4J LoggerFactory
When working with Java applications, it's not uncommon to encounter the dreaded "NoClassDefFoundError". This error occurs when the Java Virtual Machine (JVM) or a ClassLoader instance tries to load a class file but cannot find the definition for the requested class. In this blog post, we will delve into troubleshooting the "NoClassDefFoundError" specifically related to the SLF4J (Simple Logging Facade for Java) LoggerFactory class.
Understanding SLF4J and LoggerFactory
SLF4J serves as a simple facade or abstraction for various logging frameworks, allowing the end user to plug in the desired logging framework at deployment time. LoggerFactory, a core component of SLF4J, provides methods for obtaining loggers, enabling applications to write log messages.
A typical scenario where "NoClassDefFoundError" occurs with SLF4J LoggerFactory is when the LoggerFactory class is referenced in the code, but the necessary SLF4J JAR file is not present in the classpath during runtime.
Verify SLF4J Dependency
The first step in troubleshooting "NoClassDefFoundError" for SLF4J LoggerFactory is to ensure that the SLF4J dependency is correctly added to the project. This involves verifying the presence of the SLF4J JAR files in the project's classpath.
Gradle
implementation 'org.slf4j:slf4j-api:1.7.32'
implementation 'org.slf4j:slf4j-simple:1.7.32' // Example: using simple implementation
Maven
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.32</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.7.32</version> <!-- Example: using simple implementation -->
</dependency>
Ensure that these dependencies are included in the project's build configuration file and that the correct version numbers are specified.
Classpath Configuration
If the SLF4J dependencies are correctly included in the project, the next step is to verify the classpath configuration during runtime. The presence of SLF4J JAR files in the classpath during compilation might not guarantee their presence during runtime.
Ensure that the JAR files containing SLF4J classes are included in the classpath when executing the application. This can be achieved by verifying the runtime environment, build scripts, or any configuration files responsible for setting the classpath.
Dependency Conflict
Another potential cause of "NoClassDefFoundError" for SLF4J LoggerFactory is a dependency conflict. It's possible that another library in the project includes a conflicting version of SLF4J, leading to runtime issues.
To identify and resolve dependency conflicts, utilize tools like mvn dependency:tree
(Maven) or ./gradlew dependencies
(Gradle). These commands provide insights into the dependency tree, enabling you to identify conflicting versions and exclude them as necessary.
Logger Integration
Assuming the SLF4J dependencies and classpath configuration are correct, it's important to ensure that the proper integration of SLF4J's LoggerFactory into the codebase is in place.
Here's an example of how to integrate SLF4J LoggerFactory:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ExampleClass {
private static final Logger logger = LoggerFactory.getLogger(ExampleClass.class);
public void doSomething() {
logger.info("Logging a message");
}
}
In this example, the LoggerFactory.getLogger
method is used to obtain a logger for the class. Ensure that the correct import statements are used, and the logger is instantiated using the appropriate class.
Wrapping Up
In conclusion, troubleshooting "NoClassDefFoundError" for SLF4J LoggerFactory involves verifying the presence of SLF4J dependencies, ensuring proper classpath configuration, resolving dependency conflicts, and integrating the LoggerFactory into the codebase correctly.
By following the steps outlined in this post, you can effectively address and resolve issues related to the absence of SLF4J LoggerFactory at runtime, enabling seamless logging functionality within your Java applications.
Remember, meticulously examining the project's dependencies, classpath configuration, and code integration can often unearth the root cause of "NoClassDefFoundError" for SLF4J LoggerFactory, paving the way for a smooth-running Java application.
For further information on SLF4J and LoggerFactory, refer to the official SLF4J documentation and LoggerFactory Javadoc.
Happy coding!